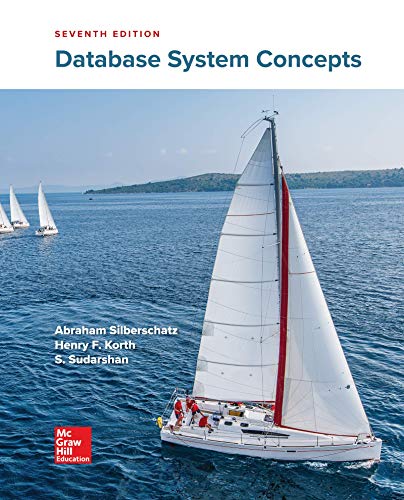
This is Java Programming! We are working with MaxHeap
Instructions: Don't modify the two codes below. You need to make a MaxHeapInterface.java that implements the bstMaxHeap in the Driver.java code. If you haven't look at the code, look at them now.
Driver.java code:
/**
A driver that demonstrates the class BstMaxHeap.
@author Frank M. Carrano
@author Timothy M. Henry
@version 5.0
*/
public class Driver
{
public static void main(String[] args)
{
String jared = "Jared";
String brittany = "Brittany";
String brett = "Brett";
String doug = "Doug";
String megan = "Megan";
String jim = "Jim";
String whitney = "Whitney";
String matt = "Matt";
String regis = "Regis";
MaxHeapInterface<String> aHeap = new BstMaxHeap<>();
aHeap.add(jared);
aHeap.add(brittany);
aHeap.add(brett);
aHeap.add(doug);
aHeap.add(megan);
aHeap.add(jim);
aHeap.add(whitney);
aHeap.add(matt);
aHeap.add(regis);
if (aHeap.isEmpty())
System.out.println("The heap is empty - INCORRECT");
else
System.out.println("The heap is not empty; it contains " +
aHeap.getSize() + " entries.");
System.out.println("The largest entry is " + aHeap.getMax());
System.out.println("\n\nRemoving entries in descending order:");
while (!aHeap.isEmpty())
System.out.println("Removing " + aHeap.removeMax());
System.out.println("\n\nTesting constructor with array parameter:\n");
String[] nameArray = {jared, brittany, brett, doug, megan,
jim, whitney, matt, regis};
MaxHeapInterface<String> anotherHeap = new BstMaxHeap<>(nameArray);
if (anotherHeap.isEmpty())
System.out.println("The heap is empty - INCORRECT");
else
System.out.println("The heap is not empty; it contains " +
anotherHeap.getSize() + " entries.");
System.out.println("The largest entry is " + anotherHeap.getMax());
System.out.println("\n\nRemoving entries in descending order:");
while (!anotherHeap.isEmpty())
System.out.println("Removing " + anotherHeap.removeMax());
} // end main
} // end Driver
HERE IS THE OUTPUT WE'RE SUPPOSED TO GET AFTER WE MAKE THE MAXHEAPINTERFACE CODE:
/*
The heap is not empty; it contains 9 entries.
The largest entry is Whitney
Removing entries in descending order:
Removing Whitney
Removing Regis
Removing Megan
Removing Matt
Removing Jim
Removing Jared
Removing Doug
Removing Brittany
Removing Brett
Testing constructor with array parameter:
The heap is not empty; it contains 9 entries.
The largest entry is Whitney
Removing entries in descending order:
Removing Whitney
Removing Regis
Removing Megan
Removing Matt
Removing Jim
Removing Jared
Removing Doug
Removing Brittany
*/

Step by stepSolved in 5 steps with 2 images

- Stuck on Java again. Have to complete this using for-loops but everything I've tried has just given me errors. Any help would be appreciated!arrow_forwardin Javaarrow_forwardImplement the Board class. Make sure to read through those comments so that you know what is required. import java.util.Arrays; import java.util.Random; public class Board { // You don't have to use these constants, but they do make your code easier to read public static final byte UR = 0; public static final byte R = 1; public static final byte DR = 2; public static final byte DL = 3; public static final byte L = 4; public static final byte UL = 5; // You need a random number generator in order to make random moves. Use rand below private static final Random rand = new Random(); private byte[][] board; /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. Note that making random moves * could result in the board being solved. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { // TODO } /** * Construct a puzzle board using a 2D array of bytes to indicate the contents *…arrow_forward
- THIS IS MEANT TO BE IN JAVA. What we've learned so far is variables, loops, and we just started learning some array today. The assignment is to get an integer from input, and output that integer squared, ending with newline. But i've been given some instructions that are kind of confusing to me. Please explain why and please show what the end result is. Here are some extra things i've been told to do with the small amount of code i've already written... Type 2 in the input box, then run the program so I can note that the answer is 4 Type 3 in the input box instead, run, and note the output is 6. Change the output statement to output a newline: System.out.println(userNumSquared);. Type 2 in the input box Change the program to use * rather than +, and try running with input 2 (output is 4) and 3 (output is now 9, not 6 as before). This is what I have so far... import java.util.Scanner; public class NumSquared {public static void main(String[] args) {Scanner scnr = new…arrow_forwardPlease help and thank you in advance. The language is Java. I have to write a program that takes and validates a date but it won't output the full date. Here is my current code: import java.util.Scanner; public class P1 { publicstaticvoidmain(String[]args) { // project 1 // date input // date output // Create a Scanner object to read from the keyboard. Scannerkeyboard=newScanner(System.in); // Identifier declarations charmonth; intday; intyear; booleanisLeapyear; StringmonthName; intmaxDay; // Asks user to input date System.out.println("Please enter month: "); month=(char)keyboard.nextInt(); System.out.println("Please enter day: "); day=keyboard.nextInt(); System.out.println("Please enter year: "); year=keyboard.nextInt(); // Formating months switch(month){ case1: monthName="January"; break; case2: monthName="February"; break; case3: monthName="March"; break; case4: monthName="April"; break; case5: monthName="May"; break; case6: monthName="June"; break; case7:…arrow_forwardThis is Java Programming! We are working with MaxHeap Instructions: Don't modify the two codes below. You need to make a MaxHeapInterface.java that implements the bstMaxHeap in the Driver.java code. If you haven't look at the code, look at them now. Driver.java code: /** A driver that demonstrates the class BstMaxHeap. @author Frank M. Carrano @author Timothy M. Henry @version 5.0*/public class Driver {public static void main(String[] args) { String jared = "Jared"; String brittany = "Brittany"; String brett = "Brett"; String doug = "Doug"; String megan = "Megan"; String jim = "Jim"; String whitney = "Whitney"; String matt = "Matt"; String regis = "Regis"; MaxHeapInterface<String> aHeap = new BstMaxHeap<>(); aHeap.add(jared); aHeap.add(brittany); aHeap.add(brett); aHeap.add(doug); aHeap.add(megan); aHeap.add(jim); aHeap.add(whitney); aHeap.add(matt); aHeap.add(regis); if (aHeap.isEmpty()) System.out.println("The heap is empty -…arrow_forward
- Please help me solve this with java .... just the HangMan HangMan instruction class : • HangMan is a game in which a player tries to guess a word based on a given hint. For example, if the given hint is “movie”, then the player must guess a movie name. If the given hint is a “country”, then the player must guess a country name, and so on.• The game starts by showing a message on the screen that shows the hint and all letters in the word but obscured as dashes (-). Then, the game will allow the player to guess 5 letters. If the player gives a letter that actually exists in the word, then this letter will be revealed. Afterwards, the game will ask the player to give the answer. If the given answer is correct, then the game will show a message that the player has won 5 points. Otherwise, the game will show a message that the player has lost. Below is one possible game scenario, in which the word is “iron man”, and the hint is “movie”. Note that the text in green color is the…arrow_forwardJava Question - Instructions are in the attached pictures. The picture titled "Screenshot..." are the instructions for building the specific code, while the "Final Output" picture shows what the output of the code should look like. Thank you.arrow_forwardPlease write in java. Add comments but make the comments short. No need for really long comments. Please keep code very neat and simple , dont add anything unneccesary in the code if you weren't instructed to do so. If youve answered this before please dont copy and paste from a previous question! (Rewrite it in another way)..... Also be sure to read the instructions below carefully. The Instructions attached below will explain everything you'll be doing. NO THIS is not a homework assignment, it's practice work. Please type the code out so that I can copy and paste it into my IDE and see the output for my self but also still provide a screenshot of your output!arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
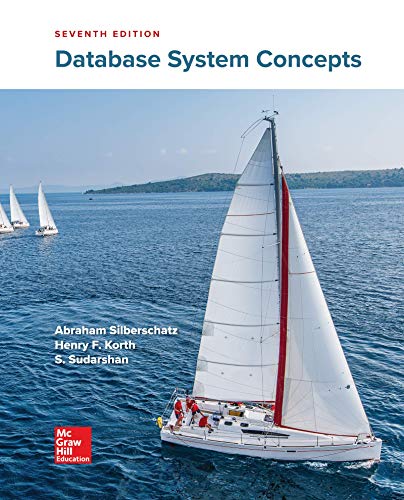
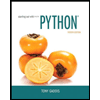
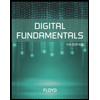
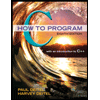
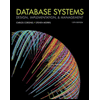
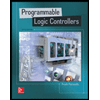