C++ program VehicleonRoad Company provides car rental services. The company provides option to rent from three different types of vehicles – S, M or L for small, medium and large . These vehicle types are classified according to size based on the number of passengers it can carry. The rental prices are charged per day according to the initial cost. A once off refundable cost as deposit needs to paid when the car is picked up for collection. The rental prices and deposit required are listed in the table below. Type of Car Initial Cost Deposit S R500.00 R1130.00 M R620.50 R1136.25 L R740.87 R1240.50 You are tasked to create a C++ program that includes the following functions: amountDue() typeTotal() determineMost() and main() Each of the functions described must have the appropriate parameters. Take note of missing information indicated with ?? that you need to identify to complete function definitions. Your program must consist of the following functions: ?? AmtDue(?? carType, ?? daysHired, ?? InitialCost, ?? additionalCost, ?? finalAmountDue) The function must use the table provided to compute the final amount due based on the initial cost and the deposit cost for the specified car type per customer. A switch statement must be used. This function accepts as parameters carType and daysHired by value and accepts as parameters by reference InitialCost, additionalCost and finalAmountDue. The rental prices are charged per day based on the initial cost. A once off refundable deposit cost needs to be paid when the car is picked up for collection. A 15% VAT needs to added to determine the final amount due by the customer for car rental. The InitialCost, additionalCost and finalAmountDue need to be returned to the calling function. 2. ?? typeTotal(?? daysHired, ?? carType, ?? daysHiredTypeS, ?? daysHired TypeM, ?? daysHiredTypeL) The function must determine the total rented in days per car type. This function accepts as parameters daysHired and carType by value and accepts as parameters by reference daysHiredTypeS, daysHiredTypeM and daysHiredTypeL. The daysHiredTypeS, daysHiredTypeM and daysHiredTypeL needs to be returned to the calling function. 3. ?? detemineMost(?? daysHired, ?? clientName, ?? highestName, ?? highestDays) The procedure must determine the name of the client who rented the car for the longest duration. This procedure accepts as parameters daysHired and clientName by value and accepts as parameters by reference highestName and highestDays. The highestName and highestDays needs to be returned to the calling function. int main() Consider the definition of the function main partially provided (see figure 2.1). Take note of missing code indicated with ??? as well as comments to guide you through coding and completing the main function. The necessary preprocessor directives to compile and run your program is also provided. You are required to tests each of the functions defined above in main and complete the following: Declare all function prototypes. Use the client name as your sentinel, with a value of END to indicate the end of the clients. Use a Pre-test loop. Prompt the use to enter car type and number of days required to rent the specified car type. Calculate the final amount due for the car type specified for each client. Calculate and display accumulated totals for all clients. Determine and display total rented in days for each type car. Determine and display client who hired car for the most number of days Ensure you all format floating-point values on output operations to 2 decimal places.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
C++ program
VehicleonRoad Company provides car rental services. The company provides option to rent from three different types of vehicles – S, M or L for small, medium and large . These vehicle types are classified according to size based on the number of passengers it can carry. The rental prices are charged per day according to the initial cost. A once off refundable cost as deposit needs to paid when the car is picked up for collection. The rental prices and deposit required are listed in the table below.
Type of Car |
Initial Cost |
Deposit |
S |
R500.00 |
R1130.00 |
M |
R620.50 |
R1136.25 |
L |
R740.87 |
R1240.50 |
You are tasked to create a C++ program that includes the following functions:
- amountDue()
- typeTotal()
- determineMost() and
- main()
Each of the functions described must have the appropriate parameters. Take note of missing information indicated with ?? that you need to identify to complete function definitions. Your program must consist of the following functions:
- ?? AmtDue(?? carType, ?? daysHired, ?? InitialCost, ?? additionalCost, ??
finalAmountDue)
The function must use the table provided to compute the final amount due based on the initial cost and the deposit cost for the specified car type per customer. A switch statement must be used.
This function accepts as parameters carType and daysHired by value and accepts as parameters by reference InitialCost, additionalCost and finalAmountDue.
The rental prices are charged per day based on the initial cost. A once off refundable deposit cost needs to be paid when the car is picked up for collection. A 15% VAT needs to added to determine the final amount due by the customer for car rental. The InitialCost, additionalCost and finalAmountDue need to be returned to the calling function.
2.
?? typeTotal(?? daysHired, ?? carType, ?? daysHiredTypeS, ?? daysHired TypeM, ?? daysHiredTypeL)
The function must determine the total rented in days per car type.
This function accepts as parameters daysHired and carType by value and accepts as parameters by reference daysHiredTypeS, daysHiredTypeM and daysHiredTypeL. The daysHiredTypeS, daysHiredTypeM and daysHiredTypeL needs to be returned to the calling function.
3.
?? detemineMost(?? daysHired, ?? clientName, ?? highestName, ?? highestDays)
The procedure must determine the name of the client who rented the car for the longest duration.
This procedure accepts as parameters daysHired and clientName by value and accepts as parameters by reference highestName and highestDays.
The highestName and highestDays needs to be returned to the calling function.
- int main() Consider the definition of the function main partially provided (see figure 2.1). Take note of missing code indicated with ??? as well as comments to guide you through coding and completing the main function. The necessary preprocessor directives to compile and run your program is also provided.
You are required to tests each of the functions defined above in main and complete the following:
- Declare all function prototypes.
- Use the client name as your sentinel, with a value of END to indicate the end of the clients. Use a Pre-test loop.
- Prompt the use to enter car type and number of days required to rent the specified car type.
- Calculate the final amount due for the car type specified for each client.
- Calculate and display accumulated totals for all clients.
- Determine and display total rented in days for each type car.
- Determine and display client who hired car for the most number of days
- Ensure you all format floating-point values on output operations to 2 decimal places.
See figures 2.2 for sample output.
![File
Tools
View
DISTRIBUTE LOCKDOWN 10(2) (Protected View) - Word
PROTECTED VIEW Be careful-files from the Internet can contain viruses. Unless you need to edit, it's safer to stay in Protected View.
Enable Editing
//EXAM D CODE TOTAL[31]
// include preprocessive directives PROVIDED
#include <iostream>
#include <iomanip>
using namespace
std;
// DEFINE PROTOTYPES
// DEFINE PROTOTYPES AmtDue // (1)
// DEFINE PROTOTYPES detemineMost /I (1)
// DEFINE PROTOTYPES typeTotal // (1)
int main()
// VARIABLES PROVIDED
char cType; // small, mid-size, large car types
int dHired;
string customerName;
double InitCost;
double
addCost;
double fAmountDue;
double totAmountDue = 0.0;
int dHiredTypes = 0;
int dHiredTypeM = 0;
dHiredTypel = 0;
string highName = " ";
int highDays = 0;
// Use the client name as your sentinel, with a value of END to indicate the end of the
clients. Use a Pre-test loop.
// Prompt user for inputs
//Calculate the final amount due for the car type specified for each client.
// Calculate and display accumulated totals for all clients.
// Determine and display total rented in days for each type car.
// Determine
// Ensure you all format floating-point values on output operations to 2 decimal places.
and display client who hired car for the most number of days
return 0;
}
// Code AmtDue function. The procedure must use table provided to compute initial cost
and additional cost and final amount due. Include vat in the final calculation
// Code typeTotal function.
// Code determineMost function.
Figure 2.1
100%](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6d6ac471-6557-492d-aea2-9dab3cc4e91c%2F293d82a1-2d5d-435b-9431-3100637fedf1%2F8ylxa6n_processed.jpeg&w=3840&q=75)
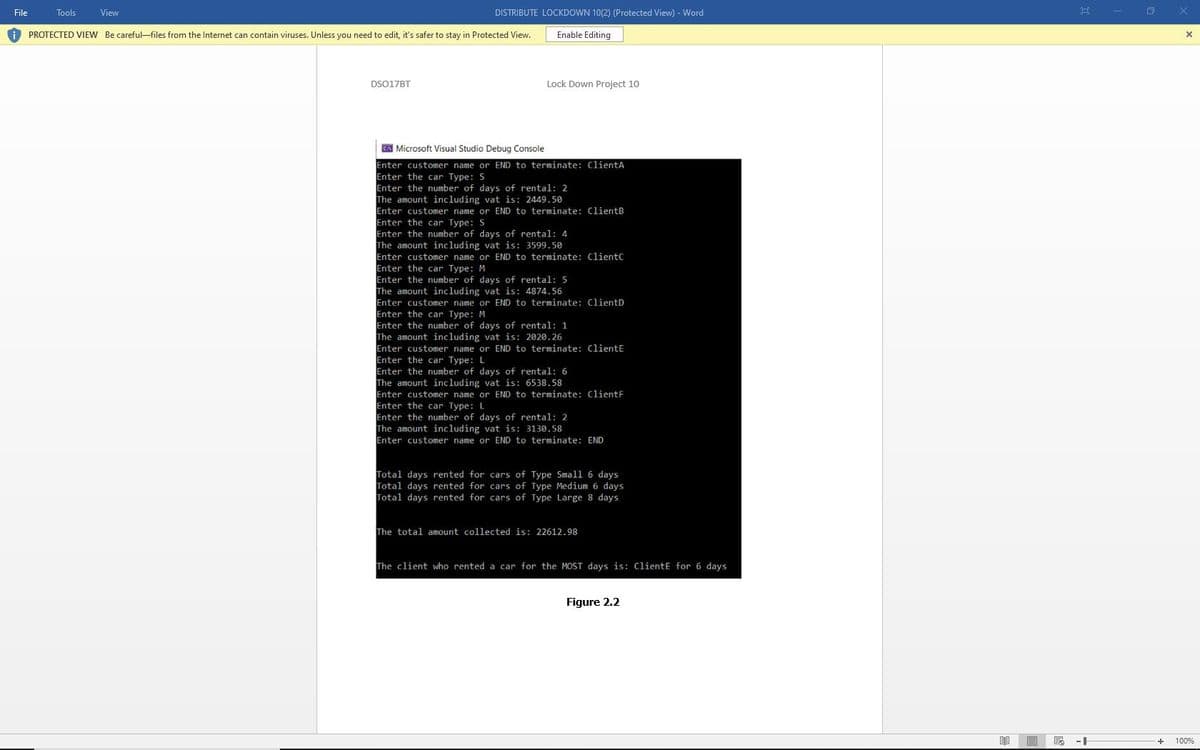

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

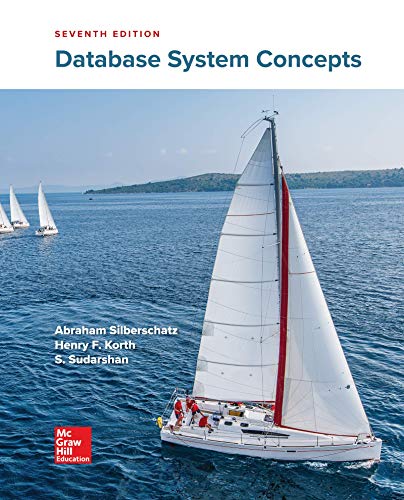
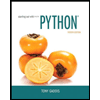
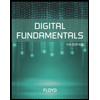
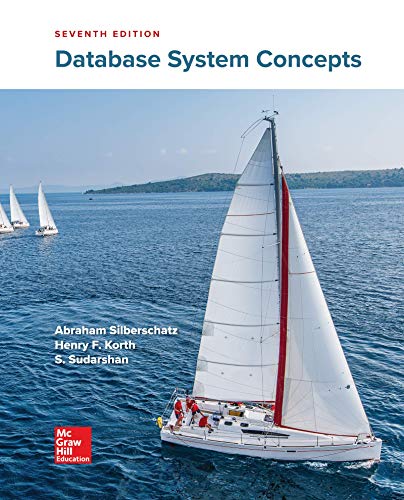
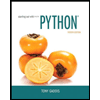
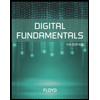
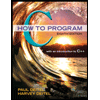
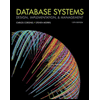
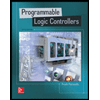