Code using c++ 3. From Person to People by CodeChum Admin Now that we have created a Person, it's time to create more Person and this tech universe shall be filled with people! Instructions: In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age and character value for its gender. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. In the main() function, there's a pre-created array of 5 Persons. Your task is to ask the user for the values of the age and gender of these Persons. Then, once you've set their ages and genders, call the displayPerson() function and pass them one by one. Input 1. A series of ages and genders of the 5 Persons Output Person·#1 Enter·Person's·age:·24 Enter·Person's·gender:·M Person·#2 Enter·Person's·age:·21 Enter·Person's·gender:·F Person·#3 Enter·Person's·age:·22 Enter·Person's·gender:·F Person·#4 Enter·Person's·age:·60 Enter·Person's·gender:·F Person·#5 Enter·Person's·age:·64 Enter·Person's·gender:·M PERSON·DETAILS: Age:·24 Gender:·Male PERSON·DETAILS: Age:·21 Gender:·Female PERSON·DETAILS: Age:·22 Gender:·Female PERSON·DETAILS: Age:·60 Gender:·Female PERSON·DETAILS: Age:·64 Gender:·Male
Code using c++ 3. From Person to People by CodeChum Admin Now that we have created a Person, it's time to create more Person and this tech universe shall be filled with people! Instructions: In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age and character value for its gender. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. In the main() function, there's a pre-created array of 5 Persons. Your task is to ask the user for the values of the age and gender of these Persons. Then, once you've set their ages and genders, call the displayPerson() function and pass them one by one. Input 1. A series of ages and genders of the 5 Persons Output Person·#1 Enter·Person's·age:·24 Enter·Person's·gender:·M Person·#2 Enter·Person's·age:·21 Enter·Person's·gender:·F Person·#3 Enter·Person's·age:·22 Enter·Person's·gender:·F Person·#4 Enter·Person's·age:·60 Enter·Person's·gender:·F Person·#5 Enter·Person's·age:·64 Enter·Person's·gender:·M PERSON·DETAILS: Age:·24 Gender:·Male PERSON·DETAILS: Age:·21 Gender:·Female PERSON·DETAILS: Age:·22 Gender:·Female PERSON·DETAILS: Age:·60 Gender:·Female PERSON·DETAILS: Age:·64 Gender:·Male
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter13: Structures
Section: Chapter Questions
Problem 2PP
Related questions
Question
Code using c++
3. From Person to People
by CodeChum Admin
Now that we have created a Person, it's time to create more Person and this tech universe shall be filled with people!
Instructions:
- In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age and character value for its gender. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. In the main() function, there's a pre-created array of 5 Persons.
- Your task is to ask the user for the values of the age and gender of these Persons.
- Then, once you've set their ages and genders, call the displayPerson() function and pass them one by one.
Input
1. A series of ages and genders of the 5 Persons
Output
Person·#1
Enter·Person's·age:·24
Enter·Person's·gender:·M
Person·#2
Enter·Person's·age:·21
Enter·Person's·gender:·F
Person·#3
Enter·Person's·age:·22
Enter·Person's·gender:·F
Person·#4
Enter·Person's·age:·60
Enter·Person's·gender:·F
Person·#5
Enter·Person's·age:·64
Enter·Person's·gender:·M
PERSON·DETAILS:
Age:·24
Gender:·Male
PERSON·DETAILS:
Age:·21
Gender:·Female
PERSON·DETAILS:
Age:·22
Gender:·Female
PERSON·DETAILS:
Age:·60
Gender:·Female
PERSON·DETAILS:
Age:·64
Gender:·Male
![Output
main.cpp
1 #include <iostream>
Person #1
2 using namespace std;
Enter Person's age: 24
4 typedef struct {
int age;
Enter Person's gender: M
char gender;
} Person;
Person #2
Enter Person's age: 21
8
Enter Person's gender: F
9 void displayPerson (Person);
10
11 int main(void) {
Person #3
12
Person persons[5];
Enter Person's age: 22
13
Enter Person's gender: F
14
15
16
return 0;
Person #4
Enter Person's age: 60
17 }
18
Enter Person's gender: F
19 void displayPerson(Person p) {
cout <« "PERSON DETAILS:" « endl;
cout <« "Age: " << p.age « endl;
cout << "Gender: ";
20
Person #5
21
Enter Person's age: 64
22
if(p.gender == 'M') {
cout <« "Male";
se
cout <« "Female";
}
cout <« endl « endl;
Enter Person's gender: M
23
24
25
PERSON DETAILS:
26
Age: 24
27
Gender: Male
28
29기
PERSON DETAILS:
Age: 21
Gender: Female
PERSON DETAILS:
Age: 22
Gender: Female
PERSON DETAILS:
Age: 60
Gender: Female
PERSON DETAILS:
Age: 64
Gender: Male](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2cf9b52f-1124-40f9-8586-0ece4ddd84ef%2Fbcb10397-ee53-4fa5-9a75-717ac9774f39%2Ftxbaklh_processed.png&w=3840&q=75)
Transcribed Image Text:Output
main.cpp
1 #include <iostream>
Person #1
2 using namespace std;
Enter Person's age: 24
4 typedef struct {
int age;
Enter Person's gender: M
char gender;
} Person;
Person #2
Enter Person's age: 21
8
Enter Person's gender: F
9 void displayPerson (Person);
10
11 int main(void) {
Person #3
12
Person persons[5];
Enter Person's age: 22
13
Enter Person's gender: F
14
15
16
return 0;
Person #4
Enter Person's age: 60
17 }
18
Enter Person's gender: F
19 void displayPerson(Person p) {
cout <« "PERSON DETAILS:" « endl;
cout <« "Age: " << p.age « endl;
cout << "Gender: ";
20
Person #5
21
Enter Person's age: 64
22
if(p.gender == 'M') {
cout <« "Male";
se
cout <« "Female";
}
cout <« endl « endl;
Enter Person's gender: M
23
24
25
PERSON DETAILS:
26
Age: 24
27
Gender: Male
28
29기
PERSON DETAILS:
Age: 21
Gender: Female
PERSON DETAILS:
Age: 22
Gender: Female
PERSON DETAILS:
Age: 60
Gender: Female
PERSON DETAILS:
Age: 64
Gender: Male
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
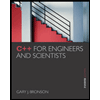
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
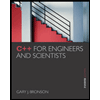
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr