C++ programming language Please help me complete this program; i really appreciate it. Images attached are the task and what the Output should be. below is the provided code that i need to edit in order to make the program output the ideal output: #include #include using namespace std; class Pet{ // name; // age; // weight; public: Pet(); Pet(string n, int a, int w); void setName( string n); void setAge(int a); void setWeight(int w); string getName() ; int getAge() ; int getWeight() ; virtual string getLifespan(); //after learning polymorphism void display(); }; // end class Pet class Dog /* write code: Dog inherits from Pet*/ { // breed; public: Dog(); Dog(string n, int a, int w, string b); void setBreed(string b); string gerBreed(); string getLifespan(); //after learning polymorphism void display(); }; //end of Dog class class Rock /* write code: Rock inherits from Pet*/ { public: Rock(); string getLifespan(); //after learning polymorphism void display(); }; int main() { // inheritance cout<<"--------------Demonstration of the concept of inheritance----------------"<
C++ programming language
Please help me complete this program; i really appreciate it.
Images attached are the task and what the Output should be.
below is the provided code that i need to edit in order to make the program output the ideal output:
#include <iostream>
#include <string>
using namespace std;
class Pet{
// name;
// age;
// weight;
public:
Pet();
Pet(string n, int a, int w);
void setName( string n);
void setAge(int a);
void setWeight(int w);
string getName() ;
int getAge() ;
int getWeight() ;
virtual string getLifespan(); //after learning polymorphism
void display();
}; // end class Pet
class Dog /* write code: Dog inherits from Pet*/ {
// breed;
public:
Dog();
Dog(string n, int a, int w, string b);
void setBreed(string b);
string gerBreed();
string getLifespan(); //after learning polymorphism
void display();
}; //end of Dog class
class Rock /* write code: Rock inherits from Pet*/ {
public:
Rock();
string getLifespan(); //after learning polymorphism
void display();
};
int main()
{ // inheritance
cout<<"--------------Demonstration of the concept of inheritance----------------"<<endl;
// Declare a static object P1 of class type Pet, that holds an object of class type Pet
// write code here :
//....... P1........... ;
//Call the function display for the object P1
P1.display();
/* declare a static object P2 of class type Pet, that holds an object of class type Dog
name= “Lily”, age=6, weight =95 and breed= “Irish setter”.
*/
// write code here :
//........ P2.................................... ;
//Call the function display for the object P2
P2.display();
// declare a static object P3 of class type Pet, that holds an object of class type Rock,
// write code here :
//........ P3......;
// change its name to : “Statue”
// write code here :
//P3.............(“Statue”) ;
//Call the function display for the object P3
P3.display();
// -------------------------------- Polymorphism----------------------------------------//
// Note: we will use pointers to allocate dynamic memory.
// Declare a dynamic object P4 of class type Pet, that holds an object of class type Pet
// write code here :
//...........* P4 = new ...............................;
// declare a dynamic object P5 of class type Pet, that holds an object of class type Dog
//with name= “Bella”, age=5, weight =50 and breed= “Dalmatian”.
// write code here :
//...........* P5 = new ................................. ;
// declare a dynamic object P6 of class type Pet, that holds an object of class type Rock,
// write code here :
//...........* P6 = new ................................. ;
// change its name to : “Sculpture”
// write code here :
// P6.........................("Sculpture");
cout<<"\n--------------Demonstration of the concept of Polymorphism----------------"<<endl;
// Display the name and the Life span of P4, P5 and P6:
// write code here :
// cout<<P4............<<": "<<P4....................<<endl;
// write code here :
// cout<<P5............<<": "<<P5....................<<endl;
// write code here :
// cout<<P6............<<": "<<P6....................<<endl;
return 0;
}
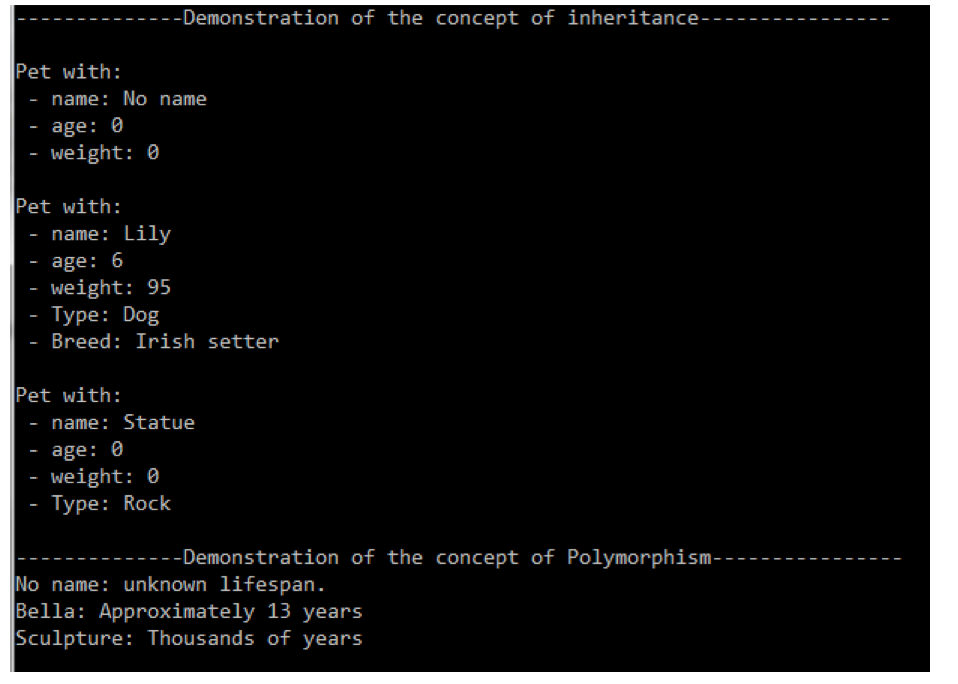
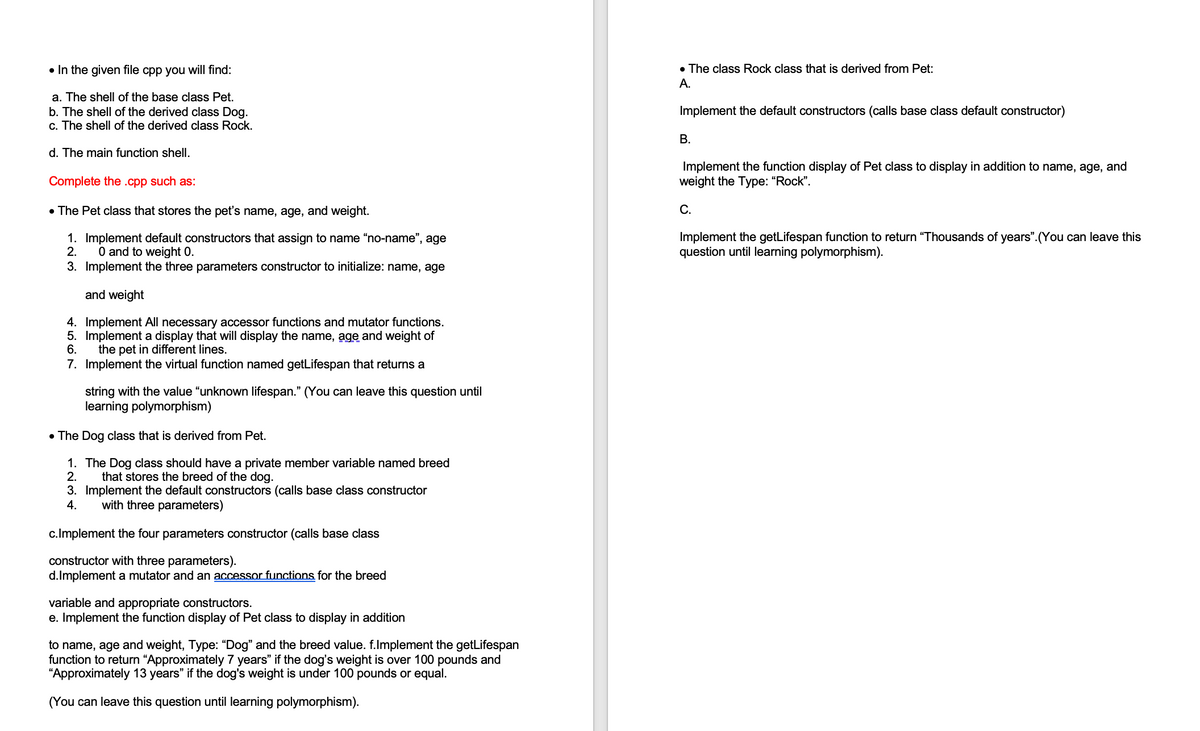

#include <iostream>
#include <string>
using namespace std;
class Pet{
string name;
int age;
int weight;
public:
// constructors
Pet() : name("No name"), age(0), weight(0)
{}
Pet(string n, int a, int w) : name(n), age(a), weight(w)
{}
// setters
void setName(string n) { name = n;}
void setAge(int a) { age = a;}
void setWeight(int w) { weight = w;}
// getters
string getName() { return name;}
int getAge() {return age;}
int getWeight() {return weight;}
// virtual function
virtual string getLifespan()
{
return "Pet Lifespan";
}
// display Pet details
void display()
{
cout<<"Name: " <<name<<endl;
cout<<"Age: "<<age<<endl;
cout<<"Weight: "<<weight<<endl;
}
};
class Dog: public Pet
{
private:
string breed;
public:
// constructors
Dog() : Pet(), breed("No Breed")
{}
Dog(string n, int a, int w, string b) : Pet(n, a, w), breed(b)
{}
// setter
void setBreed(string b) { breed = b;}
// getters
string getBreed() {return breed;}
// overriden getLifeSpan from Pet class
string getLifespan() { return "Dog Lifespan";}
// display details of Dog
void display()
{
cout<<"Name: " <<getName()<<endl;
cout<<"Age: "<<getAge()<<endl;
cout<<"Weight: "<<getBreed()<<endl;
cout<<"Breed: "<<breed<<endl;
}
};
class Rock : public Pet
{
public:
// constructor
Rock(): Pet()
{}
// overriden getLifeSpan from Pet class
string getLifespan() { return "Rock lifespan";}
// display details of Rock
void display()
{
cout<<"Name: " <<getName()<<endl;
cout<<"Age: "<<getAge()<<endl;
cout<<"Weight: "<<getWeight()<<endl;
}
};
int main()
{
// inheritance
cout<<"------ Demonstration of the concept of inheritance ------"<<endl;
// declare static object P1 of class type Pet that holds an object of class type Pet
static Pet P1 = Pet();
// call the function display for the object P1
P1.display();
// declare a static object P2 of class type Pet that holds an object of type Dog
static Pet P2 = Dog("Lily",6,95,"Irish setter");;
// call the function for the object P2
P2.display();
// declare a static object P3 of class type Pet that holds an object of type Rock
static Pet P3 = Rock();;
// change its name to Statue
P3.setName("Statue");
// call the display function for the object P3
P3.display();
// Polymorphic
// Declare a dynamic object P4 of class type Pet that holds an object of class type Pet
Pet *P4 = new Pet();
// Declare a dynamic object P5 of class type Pet that holds an object of class type Dog
Pet *P5 = new Dog("Bella",5, 50, "Dalmatian");
// Declare a dynamic object P6 of class type Pet that holds an object of class type Rock
Pet *P6 = new Rock();
// change its name to Sculpture
P6->setName("Sculpture");
cout<<"\n------------ Demonstration of the concept of Polymorphism -------------"<<endl;
// Display the name and the Life span of P4, P5 and P6
cout<<P4->getName()<<": "<<P4->getLifespan()<<endl;
cout<<P5->getName()<<": "<<P5->getLifespan()<<endl;
cout<<P6->getName()<<": "<<P6->getLifespan()<<endl;
return 0;
}
//end of program
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

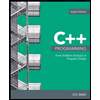
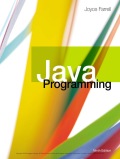
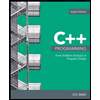
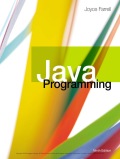