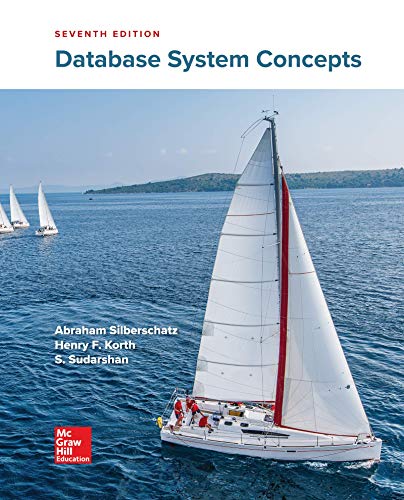
Concept explainers
C++
Question: Make an execution chart like the example below for the code provided.
Example chart:
An execution chart is a text version of the hierarchy. Indentation is used to indicate the
sublevels or calls inside a call. It also contains the data exchange between the components as
designated in the hierarchy chart. Given below is the execution chart that corresponds to the
hierarchy chart of the property tax calculation program
1.0 Main()
2.0 CalculatePropertyTax()
3.0 displayMessage( input string messageToDisplay)
3.1 return double getHomeValue()
3.2 return boolean checkHomeValue()
3.3 return double applyPropertyTax(input double homeValue)
3.4 displayPropertyTax(input homeValue)
3.5 return Boolean queryMoreData()
4.0 displayMessage(input string messageToDisplay)
4.1 return char getYesNo()
4.2 return char convertCase(input char)
3.6 displayErrorMessage()
More exlanation:
example: 1.0 means it's of depth 1, line 0 then 2.0 means it's one call inside a function (aka it's inside another function) then 2.1 means it's of the same depth,
In my code The only functions you call in main are removeDuplicates and displayAccounts, Meaning you only need to list the calls in main, Like 1.0 main() 2.0 displayAccounts() 2.1 removeDuplicates() 2.2 displayAccounts() but add the arguments into the functions as in their definitions
Main.cpp:
#include <iostream>
#include <fstream>
#include <sstream>
#include <
#include "BankAccount.h"
using namespace std;
const int SIZE = 8;
// function declaration
bool removeDuplicates(vector<BankAccount> &accountsVector);
void displayAccounts(vector<BankAccount> accountsVector);
int main()
{
ifstream fin;
fin.exceptions(ifstream::failbit | ifstream::badbit);
try{
fin.open("BankData.dat"); // provide full path to file
vector<BankAccount> accountsVector;
string firstName, lastName;
int accountId;
int accountNumber;
double accountBalance;
string line;
while(!fin.eof())
{
getline(fin,line);
stringstream ss(line);
ss>>firstName>>lastName>>accountId>>accountNumber>>accountBalance;
BankAccount account(firstName+" "+lastName,accountId,accountNumber,accountBalance);
accountsVector.push_back(account);
}
fin.close();
if(accountsVector.size() > 0 )
{
BankAccount largest = accountsVector[0];
BankAccount smallest = accountsVector[0];
displayAccounts(accountsVector);
for(unsigned int i=0;i<accountsVector.size();i++)
{
if(accountsVector[i].getAccountBalance() > largest.getAccountBalance())
largest = accountsVector[i];
if(accountsVector[i].getAccountBalance() < smallest.getAccountBalance())
smallest = accountsVector[i];
}
cout<<"\nLargest Balance : "<<endl;
cout<<largest.toString()<<endl<<endl;
cout<<"Smallest Balance : "<<endl;
cout<<smallest.toString()<<endl<<endl;
cout<<"Using the static count, there are "<<BankAccount::count<<" accounts"<<endl;
cout<<"Using vector size, there are "<<accountsVector.size()<<" accounts"<<endl;
if(removeDuplicates(accountsVector))
{
cout<<"\nDuplicate Account Found : Reprinting List"<<endl;
displayAccounts(accountsVector);
}else
cout<<"\nDuplicate Account Not Found"<<endl;
cout<<"Using the static count, there are "<<BankAccount::count<<" accounts"<<endl;
cout<<"Using vector size, there are "<<accountsVector.size()<<" accounts"<<endl;
BankAccount account1("Amy Machado",387623 ,1244 ,1023.67);
BankAccount account2("Tak Phen",981243 ,1262 ,6423.03);
BankAccount account3("Celia Beatle",465281 ,1276 ,3.56 );
accountsVector.insert(accountsVector.begin()+2,account1);
accountsVector.insert(accountsVector.begin()+4,account2);
accountsVector.insert(accountsVector.begin()+6,account3);
cout<<"\nInserted Three New Accounts : Reprinting List"<<endl;
displayAccounts(accountsVector);
cout<<"Using the static count, there are "<<BankAccount::count<<" accounts"<<endl;
cout<<"Using vector size, there are "<<accountsVector.size()<<" accounts"<<endl;
}
}catch(ifstream::failure &e)
{
cerr<<"Error while opening/reading the input file"<<endl;
}
return 0;
}
// function to remove duplicate accounts from the vector, return true if duplicate records present else false
bool removeDuplicates(vector<BankAccount> &accountsVector)
{
bool duplicate = false;
for(unsigned int i=0;i<accountsVector.size()-1;i++)
{
for(unsigned int j=i+1;j<accountsVector.size();j++)
if(accountsVector[i].equals(accountsVector[j]))
{
duplicate = true;
accountsVector.erase(accountsVector.begin()+j);
}
}
return duplicate;
}
// function to display the accounts
void displayAccounts(vector<BankAccount> accountsVector)
{
cout<<"FAVORITE BANK - CUSTOMER DETAILS"<<endl;
cout<<string(50,'-')<<endl;
for(unsigned int i=0;i<accountsVector.size();i++)
{
cout<<accountsVector[i].toString()<<endl<<endl;
}
}
//end of program


Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- only in c++ please!arrow_forwardC++ Coding Help (Operators: new delete) Assign pointer myGas with a new Gas object. Call myGas's Read() to read the object's fields. Then, call myGas's Print() to output the values of the fields. Finally, delete myGas. Ex: If the input is 14 45, then the output is: Gas's volume: 14 Gas's temperature: 45 Gas with volume 14 and temperature 45 is deallocated. #include <iostream>using namespace std; class Gas { public: Gas(); void Read(); void Print(); ~Gas(); private: int volume; int temperature;};Gas::Gas() { volume = 0; temperature = 0;}void Gas::Read() { cin >> volume; cin >> temperature;} void Gas::Print() { cout << "Gas's volume: " << volume << endl; cout << "Gas's temperature: " << temperature << endl;} Gas::~Gas() { // Covered in section on Destructors. cout << "Gas with volume " << volume << " and temperature " << temperature << " is deallocated."…arrow_forwardWrite your program in C# with Visual Studio, or an online compiler - compile, and execute it. Upload the file with the .cs extension to Canvas for grading. Canvas will not accept any other file type. • There will be a 10% deduction for each weekly last submission. You only get a maximum of two weeks to make up for any late work; Ex: late two weeks = 20% deductions Programming Assignment 3: Chapter 4 Write, compile, and test a program called Credit Test. Write a program that asks a user to enter their Credit Score and Down payment for a loan approval process. Display credit accepted message if the user meets either of the following requirements: A minimum credit score of 675, and a down payment of $35000 or above. A credit score below 675, and a down payment of 85000 and above. A credit rejected message should display if the user doesn't meet any of the qualification criteria.arrow_forward
- C++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template: 1.0 Main()2.0 CalculatePropertyTax()3.0 displayMessage( input string messageToDisplay)3.1 return double getHomeValue()3.2 return boolean checkHomeValue()3.3 return double applyPropertyTax(input double homeValue)3.4 displayPropertyTax(input homeValue)3.5 return Boolean queryMoreData()4.0 displayMessage(input string messageToDisplay)4.1 return char getYesNo()4.2 return char convertCase(input char)3.6 displayErrorMessage() CODE: Maincpp: #include <iostream> #include <fstream> #include "BankAccount.h" using namespace std; const int SIZE = 8; // function declaration for array void fillArray (ifstream &input,BankAccount accountsArray[]); int largest(BankAccount accountsArray[]); int smallest(BankAccount accountsArray[]); void printArray(BankAccount…arrow_forward/* Interface File: Movie.h Declaration of Movie Class (Variables - "Data Members" or "Attributes" AND Functions - "Member Functions" or "Methods") */ #ifndef MOVIE_H // Include Guard or Header Guard -If already defined ignore rest of code #define MOVIE_H // Otherwise, define MOVIE_H #include<string> // Note: Not "using namespace std;" or even "using std::string" class Movie { private: std::string title = ""; // Explict scope used --> std::string int year = 0; public: Movie(std::string title = "", int year = 1888); // Declaring a Default Constructor ~Movie(); // A Destructor used for freeing up resources void set_title(std::string title_param); std::string get_title() const; // "const" safeguards class variable changes within function std::string get_title_upper() const; void set_year(int year_param); int get_year() const; }; //…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
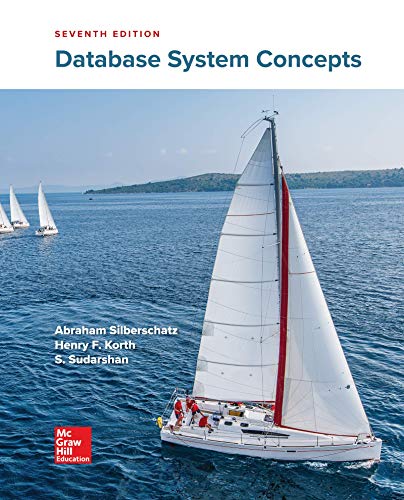
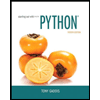
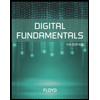
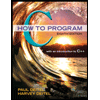
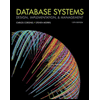
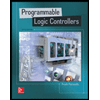