C++ Two stacks of the same type are the same if they have the same number of elements and their elements at the corresponding positions are the same. Overload the relational operator == for the class linkedStackType that returns true if two stacks of the same type are the same; it returns false otherwise. Also, write the definition of the function template to overload this operator. Write a program to test the various functions and operators of the class linkedStackType.
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
C++
Two stacks of the same type are the same if they have the same number of elements and their elements at the corresponding positions are the same. Overload the relational operator == for the class linkedStackType that returns true if two stacks of the same type are the same; it returns false otherwise. Also, write the definition of the function template to overload this operator. Write a
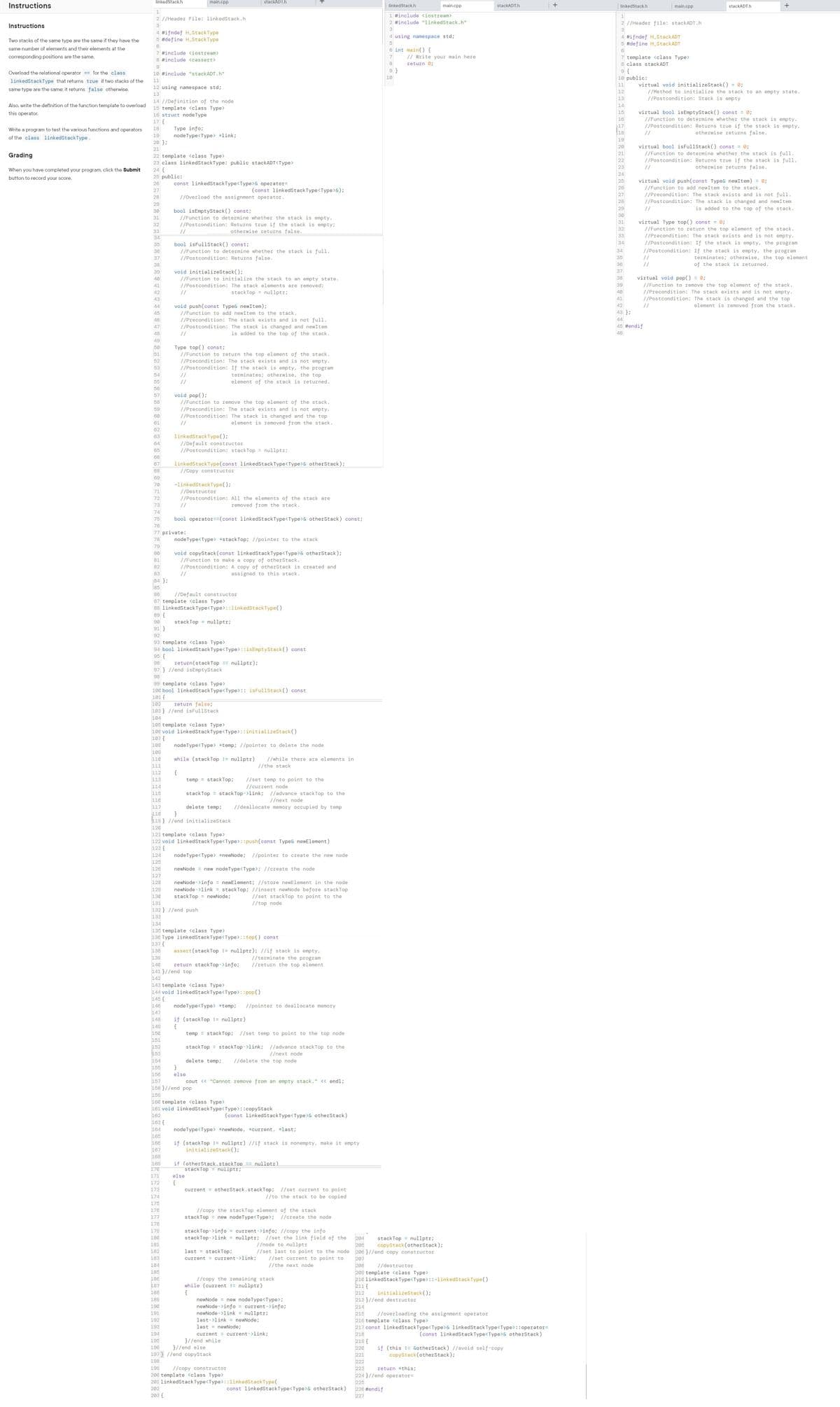

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

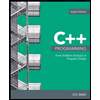
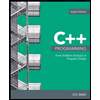