gets a linked list of ints, and reverses it
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a function that gets a linked list of ints, and reverses it. For example
- on input 1 -> 2 -> 3 -> 4, after the function finishes the execution, the list
becomes 4 -> 3 -> 2 -> 1
- If the list has one element, then it doesn’t change
- If the list is empty, then it doesn’t change
You may use the data fields in the struct and the functions provided in LL.h and LL.c.
// reverses a linked list
void LL_reverse(LL_t* list);
Test for the Function:
void test_q3() {
LL_t* lst = LLcreate();
LL_add_to_tail(lst, 1);
LL_add_to_tail(lst, 3);
LL_add_to_tail(lst, 8);
LL_add_to_tail(lst, 4);
LL_add_to_tail(lst, 3);
LL_reverse(lst);
intcorrect[] = {3,4,8,3,1};
inti;
node_t* n = lst->head;
for(i=0;i<5;i++) {
if (n==NULL) {
printf("Q3 ERROR: node %d==NULL unexpected\n", i);
return;
}
if (n->data != correct[i]) {
printf("Q3 ERROR: node%d->data==%d, expected %d\n", i, n->data, correct[i]);
return;
}
n = n->next;
}
if (n==NULL)
printf("Q3 ok\n");
}
Support file named LL.c:
// Creates and returns a new, empty list
LL_t* LLcreate() {
LL_t* ret = malloc(sizeof(LL_t));
if (ret == NULL)
returnNULL;
ret->size = 0;
ret->head = NULL;
ret->tail = NULL;
returnret;
}
// Adds the element to the head of the list
void LL_add_to_head(LL_t* list, int value) {
// create a new node
node_t* newNode = (node_t*) malloc(sizeof(node_t));
if (newNode == NULL)
return;
// set the data in the new node
newNode->data = value;
newNode->next = list->head;
if (list->size == 0) { // empty list
list->head = newNode;
list->tail = newNode;
}
else {
list->head = newNode;
}
list->size++; // update size
}
// Adds a new element to the tail of a list
void LL_add_to_tail(LL_t* list, int value) {
// create a new node
node_t* newNode = (node_t*) malloc(sizeof(node_t));
if (newNode == NULL)
return;
// set the data in the new node
newNode->data = value;
newNode->next = NULL;
if (list->size == 0) {
list->head = newNode;
list->tail = newNode;
}
else {
list->tail->next = newNode;
list->tail = newNode;
}
list->size++; // update size
}
// assumption: list is not empty
int LL_remove_from_head(LL_t* list) {
node_t* prev_head = list->head;
intret = prev_head->data;
list->head = list->head->next;
(list->size)--;
if ((list->size)==0)
list->tail = NULL;
free(prev_head);
returnret;
}
// assumption: list is not empty
int LL_remove_from_tail(LL_t* list) {
intret = list->tail->data;
// take care of the case when list size is 1
if (list->head == list->tail) {
free(list->tail);
list->head = NULL;
list->tail = NULL;
}
else {
node_t* cur = list->head;
while (cur->next != list->tail)
cur = cur->next;
// here cur->next = list->tail;
// update cur->next to be null, and update the tail
free(list->tail);
cur->next = NULL; // this is the new last element
list->tail = cur;
}
(list->size)--;
returnret;
}
// Returns the size of the list
int LL_size(const LL_t* list) {
returnlist->size;
}
// Prints the elements in the list from head to tail
void LL_print(const LL_t* list) {
node_t* current = list->head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// releases the memore used by list
void LL_free(LL_t* list) {
node_t* cur;
while (list->head != NULL) {
cur = list->head;
list->head = cur->next;
free(cur);
}
free(list);
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
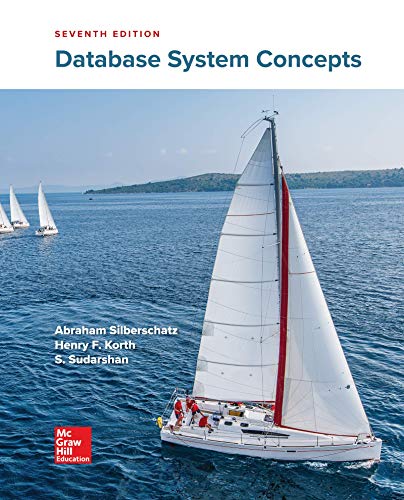
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
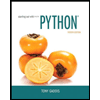
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
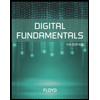
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
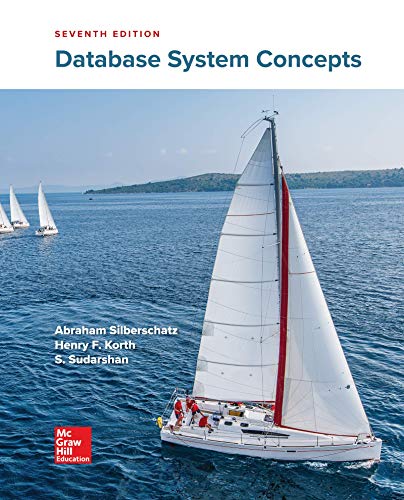
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
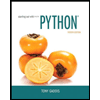
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
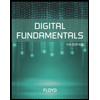
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
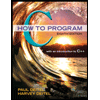
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
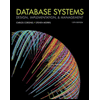
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
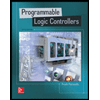
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education