C++ Write a program that simulates the minute-by-minute operation of a checkout line, such as one you might find in a retail store. Use the following parameters: Customers arrive at the checkout line and stand in line until the cashier is free. When they reach the front of the line, they occupy the cashier for some period of time (referred to as ServiceTime) measured in minutes. After the cashier is free, the next customer is served immediately. Customers arrive at the checkout line at ArrivalRate per minute. Use the function included below (customerArrived()) to return the number of customers arriving in a given minute, determined randomly. The line can only hold so many people, MaxLineSize, until new arriving customers get frustrated and leave the store without purchasing anything. The overall time of the simulation is SimulationTime, measured in minutes. The program should take 5 inputs (to be read from a text file named simtest.txt, one per line): SimulationName - a string name for the simulation. SimulationTime - total number of minutes to run the simulation (whole number). ArrivalRate - per-minute arrival rate of customers (a floating point number greater than 0 and less than 1). This number is the "percent chance" that a customer will arrive in a given minute. For example, if it is 0.4, there is a 40% chance a customer will arrive in that minute. ServiceTime - the amount each customer requires at the register (the same for all customers). MaxLineSize - the maximum size of the line. If a new customer arrives and the line has this many customers waiting, the new customer leaves the store (is "dropped"). At the end of each simulation, the program should output: The total number of customers serviced The average time per customer spent in line The average length of the line during the simulation The total number of customers who were dropped (found the line too long, then left the store). You are free to use any STL templates as needed (queue, vector, etc.). Note that you will not need a priority queue for this lab (only a regular queue, since all customers are of equal urgency). Example Run The output should look similar to this. This example is for the first test case in the sample file. Your output may vary somewhat because of the randomness in the simulation. In the below case, with ArrivalRate set to 0.1, we would expect about 200 people (0.1 * 2000) to arrive. If we add the number of customers serviced (125) with the customers leaving (97) that gives us a number (222) which is close enough to 200 to be possible for one run of the simulation. Simulation name: Short Lines -------------------------------------- Simulation time: 2000 Arrival rate: 0.1 Service time: 15 Max line size: 5 Hints Simulation Loop To run each simulation, you will want some kind of loop that runs based on the passing of time. A simple integer counter will work. Start the counter at 0 and run until the counter hits SimulationTime. This counter can also serve as your representation of the current time of the simulation at any given point. Representing Customers You will need a data structure to represent a customer. What information do you want to save when a customer enters the line? If you save a timestamp at the entry time, you will be able to calculate the amount of time they waited by comparing this timestamp to the current time when they are ready to go to the cashier. Random Functions The provided function customerArrived() returns true if a customer arrived at that minute, false otherwise. The input variable 'prob' should be expressed as a decimal percentage, for example, .80 if there is an 80% chance of a customer arriving. This comes from the input parameter 'ArrivalRate'. bool customerArrived(double prob) { double rv = rand() / (double(RAND_MAX) + 1); return (rv < prob); } Before calling customerArrived() be sure to seed the random number generator (you can do this in main()): srand(time(0)); Customers served: 125 Average wait time: 66.688 Average line length: 4.246 Total dropped customers: 97
C++
Write a
- Customers arrive at the checkout line and stand in line until the cashier is free.
- When they reach the front of the line, they occupy the cashier for some period of time (referred to as ServiceTime) measured in minutes.
- After the cashier is free, the next customer is served immediately.
- Customers arrive at the checkout line at ArrivalRate per minute. Use the function included below (customerArrived()) to return the number of customers arriving in a given minute, determined randomly.
- The line can only hold so many people, MaxLineSize, until new arriving customers get frustrated and leave the store without purchasing anything.
- The overall time of the simulation is SimulationTime, measured in minutes.
The program should take 5 inputs (to be read from a text file named simtest.txt, one per line):
- SimulationName - a string name for the simulation.
- SimulationTime - total number of minutes to run the simulation (whole number).
- ArrivalRate - per-minute arrival rate of customers (a floating point number greater than 0 and less than 1). This number is the "percent chance" that a customer will arrive in a given minute. For example, if it is 0.4, there is a 40% chance a customer will arrive in that minute.
- ServiceTime - the amount each customer requires at the register (the same for all customers).
- MaxLineSize - the maximum size of the line. If a new customer arrives and the line has this many customers waiting, the new customer leaves the store (is "dropped").
At the end of each simulation, the program should output:
- The total number of customers serviced
- The average time per customer spent in line
- The average length of the line during the simulation
- The total number of customers who were dropped (found the line too long, then left the store).
You are free to use any STL templates as needed (queue,
Example Run
The output should look similar to this. This example is for the first test case in the sample file. Your output may vary somewhat because of the randomness in the simulation. In the below case, with ArrivalRate set to 0.1, we would expect about 200 people (0.1 * 2000) to arrive. If we add the number of customers serviced (125) with the customers leaving (97) that gives us a number (222) which is close enough to 200 to be possible for one run of the simulation.
Simulation name: Short Lines -------------------------------------- Simulation time: 2000 Arrival rate: 0.1 Service time: 15 Max line size: 5
Hints
Simulation Loop
To run each simulation, you will want some kind of loop that runs based on the passing of time. A simple integer counter will work. Start the counter at 0 and run until the counter hits SimulationTime. This counter can also serve as your representation of the current time of the simulation at any given point.
Representing Customers
You will need a data structure to represent a customer. What information do you want to save when a customer enters the line? If you save a timestamp at the entry time, you will be able to calculate the amount of time they waited by comparing this timestamp to the current time when they are ready to go to the cashier.
Random Functions
The provided function customerArrived() returns true if a customer arrived at that minute, false otherwise. The input variable 'prob' should be expressed as a decimal percentage, for example, .80 if there is an 80% chance of a customer arriving. This comes from the input parameter 'ArrivalRate'.
bool customerArrived(double prob) { double rv = rand() / (double(RAND_MAX) + 1); return (rv < prob); }
Before calling customerArrived() be sure to seed the random number generator (you can do this in main()):
srand(time(0)); Customers served: 125 Average wait time: 66.688 Average line length: 4.246 Total dropped customers: 97

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

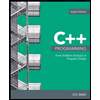
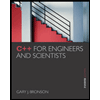
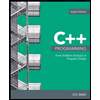
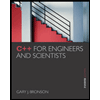