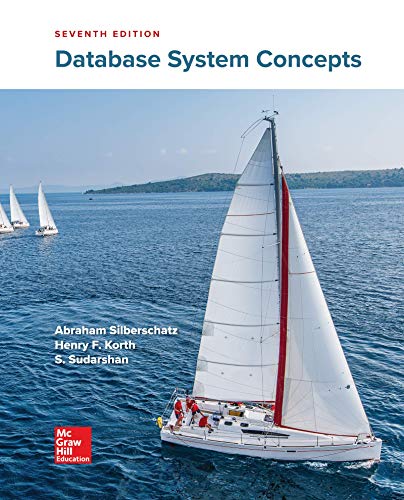
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
Implement in C
6.6.3: Function with loop: Shampoo.
Write a function PrintShampooInstructions(), with int parameter numCycles, and void return type. If numCycles is less than 1, print "Too few.". If more than 4, print "Too many.". Else, print "N: Lather and rinse." numCycles times, where N is the cycle number, followed by "Done.". End with a newline. Example output with input 2:1: Lather and rinse. 2: Lather and rinse. Done. Hint: Declare and use a loop variable.
#include <stdio.h>
/* Your solution goes here */
int main(void) {
int userCycles;
scanf("%d", &userCycles);
PrintShampooInstructions(userCycles);
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Using c++ In main.cpp, complete the function RollSpecificNumber() that takes in three parameters: a GVDie object, an integer representing a desired face number of a die, and an integer representing the goal amount of times to roll the desired face number. The function RollSpecificNumber() then rolls the die until the desired face number is rolled the goal amount of times and returns the number of rolls required. Note: For testing purposes, the GVDie objects are created in the main() function using a pseudo-random number generator with a fixed seed value. The program used during development uses a seed value of 15, but when submitted, different seed values will be used for each test case. Refer to the textbook section on random numbers to learn more about pseudo-random numbers. Ex: If the GVDie objects are created with a seed value of 15 and the input of the program is: 3 20 the output is: It took 140 rolls to get a "3" 20 times. #include <iostream>#include "GVDie.h"using…arrow_forwardWrite a Python function round_up that, given an integer, rounds it up the next multiple of 100. If the given number is already a multiple of 100, it is just returned. For example, round_up(234) returns 300 round_up(465) returns 500 round_up(400) returns 400 The round_up function does not read input or print output.arrow_forwardwrite a program in R that allows a user to do some basic math functions and return the results Instructions For this assignment, you need to create a program that allows the user to do some basicmath functions.First, ask the user if they would like to find out sqrt, log or factorial of a number, and return the results.arrow_forward
- Write a Python function to run a Parking Lot (PL) simulation, called PLSimulation, which takes four integers as parameters: • The first parameter (minArriving) is the minimum number of cars that can park in the PL during one hour. • The second parameter (maxArriving) is the maximum that can park during one hour. • The third parameter (maxLeaving) is the maximum number of cars that may leave the PL during one hour. • The fourth parameter (hours) is the number of hours to run the simulation. Your function should simulate parking and leaving cars for the specified number of hours. In each hour, the following occurs in this order: 1. A random number of cars arrive, ready to park (between the minimum and maximum). That number is added to a count of the number waiting to park. 2. The maximum number of cars that may leave, allowing the same number of cars to park, so that number is subtracted from the count of the number waiting to park (if there are that many waiting to park). Initially, the…arrow_forward13. Implement function triangleArea(a,b,c) that takes as input the lengths of the 3 sides of a triangle and returns the area of the triangle. By Heron's formula, the area of atriangle with side lengths a, b, and c is √ s ( s−a )( s−b)( s−c ) , where s=(a+b+ c )/ 2 .>>> triangleArea(2,2,2)1.7320508075688772arrow_forwardlllustrating your answer using the buggy code below, explain in your own words what it means for variables to be defined “as locally as possible”, and why it is important. This code is intended to print one of two messages depending on whether an integer input is less than a secret number or not. The function, inputInt, correctly prints the given String and then returns a number input by the user.arrow_forward
- Please write a full code in JAVA for this: A function foo has k integers as input arguments, i.e., foo(int n1, int n2, ..., int nk). Each argument may belong to a different equivalence class, which are stored in an Eq.txt file. In the file, the nth row describes the nth input. Take the second row for example. The data 1, 10; 11, 20; 21, 30 indicates that n2 has three equivalence classes separated by the semi-colons. There is an internal method “int check(int n)” that returns the equivalence class n is in. The result of check(n2 = 3) will be 1 and check(n2 = 25) will be 3. Regarding the function foo, it computes the sum of the returned values by the check function for all input arguments.Follow the Eq.txt file to automatically create test cases for Strong Normal Equivalence class testing. The input argument values are randomly generated. Store your prepared test cases to a test.txt file. Each test case comes with an expected output at the end. All values are delimited by a comma.…arrow_forwardThis is in C++ given main(), define the Team class (in files Team.h and Team.cpp). For class member function GetWinPercentage(), the formula is: teamWins / (teamWins + teamLosses) Use casting to prevent integer division. Ex: If the input is: Ravens 13 3 where Ravens is the team's name, 13 is number of team wins, and 3 is the number of team losses, the output is: Congratulations! Team Ravens has a winning average! If the input is Angels 80 82, the output is: Team Angels has a losing average. The template provided is this: #include <iostream>#include <string>#include "Team.h"using namespace std; int main(int argc, const char* argv[]) {string name;int wins;int losses;Team team; cin >> name;cin >> wins;cin >> losses; team.SetTeamName(name);team.SetTeamWins(wins);team.SetTeamLosses(losses); if (team.GetWinPercentage() >= 0.5) {cout << "Congratulations, Team " << team.GetTeamName() <<" has a winning average!" << endl;}else {cout…arrow_forwardWrite a program called p3.py that contains a function called reveal_recursive() that takes a word (string) and the length of the word (int) as input and has the following functionality: 1. prints the word where all characters are replaced by underscores 2. continue to print the word revealing one character at a time. i.e., the second line printed should print the first character followed by “_”’s representing the rest of the word. (see example below) 3. the function should end after printing the entire word once. 4. This function should be recursive Example: #the word is kangaroo ________ k_______ ka______ kan_____ kang____ kanga___ kangar__ kangaro_ kangarooarrow_forward
- Implement a function rollsToRepeat in Python that simulates a dice game in which a pair of dice are rolled repeatedly until some total on the dice occurs the specified number of times. Details: accepts one integer argument, n , the number of repeats required before the game ends rolls a pair of dice repeatedly until some dice total has repeated n times returns the total number of times the pair of dice was rolled For example, using a random seed of 85 , the sequence of rolled pairs will be : (2, 6), (5, 1),(3, 2), (2, 4), (3, 5), (2, 6), (4, 5), (5, 2), (6, 5), (5, 5), ... If you runrollsToRepeat with n=1 , only 1 repeat is required. On the first roll, the total 8=2+6 is repeated once (as wouldany first roll). So the simulation stops and the function returns 1. This always happens whenn=1 . n=2 , some total must repeat twice. This first occurs on the 4throll, when the total of 6occurs for the 2ndtime. n=3 , some total must repeat three times. This first occurs on the 6throll, when…arrow_forward53. Write a class with two instance variables, representing an old password and a new password. Write a recursive method that returns the number of places where the two passwords have different characters. The passwords can have different lengths. Write another, nonrecursive method returning whether the two passwords are sufficiently different. The method takes an int parameter indicating the minimum number of differences that qualify the passwords as being sufficiently different. Your program should include a client class to test your class.arrow_forwardImplement the following function: Code should be written in python.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
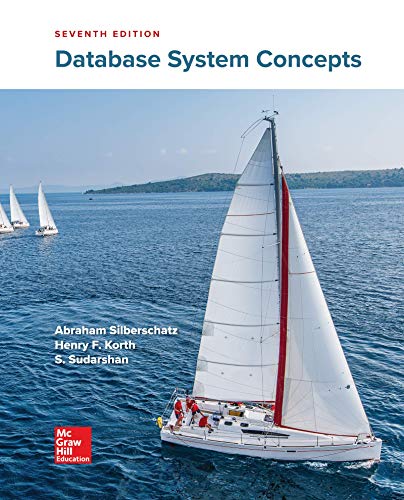
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
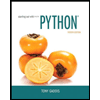
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
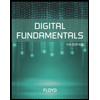
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
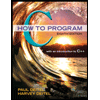
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
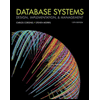
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
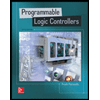
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education