C++ You are required to write a universal calculator that performs DOUBLE UP of different types of data. Especially the calculator must take the following types of input. A decimal number A float point number A binary number which must be prefixed with “0b”(e.g 0b111 ob1010 are valid 01b1010, 0b101, 01010 are invalid) A hexadecimal number which must be prefixed with “0x” (e.g. 0x19, 0xAAare valid. 01x1A, ox1A, 01A are invalid) Note Your program must implement function overloading. You can consider the examples below for your implementation. You are provided with the initial code which can be found. #include #include #include #include using namespace std; /* * IMPLEMENT YOUR OVERLOADED FUNCTIONS HERE */ /* this function takes a string and * returns 1 if the string represents a decimal * returns 2 if the string represents a floating point * returns 3 if the string represents a binary * returns 4 if the string represents a hexadecimal * return 0 if the input string is anything else */ int check_type(char *input) { int res = 1; int len = strlen(input); int i; // check the first char for special sign chars [-,+] and '.' char first = input[0]; char second = input[1]; if (first=='-' && first=='+') { if (second=='.') { res = 2; } } // got a '.' from the beginning, got a float else if(first=='.') { if (second=='.') return 0; res = 2; } //check to see if we got a prefix 0b --> expect a binary else if (first=='0' && second=='b') res = 3; // check to see if we got a prefix 0x --> expect a hexa else if(first=='0' && second=='x') res = 4; else if(first>='0'||first<='9') { if (second=='.') res = 2; } else if ( (first<'0'||first>'9') && (second<'0'||second>'9') ) return 0; for (i=2; i'9')) return 0; } // already expecting a binary, only '0' or '1' else if (res==3) { if (input[i]!='0' && input[i]!='1') return 0; } // already expecting a hexa, only [0-9] or [A-F] else if (res==4) { if ( (input[i]<'0'||input[i]>'9') && (input[i]<'A'||input[i]>'F') ) return 0; } // still seeing integer, could be a float with a '.' else if (res==1) { if (input[i]=='.') res = 2; else if (input[i]<'0' || input[i]>'9') return 0; } } return res; } int main(int argc, char* argv[]) { int type; if (argc!=2) { cerr << "Wrong number of inputs" << endl; return 0; } type = check_type(argv[1]); if (type==1) { doubleup(atoi(argv[1])); } else if (type==2) { doubleup(stof(argv[1])); } else if (type==3) { doubleup(argv[1]); } else if (type==4) { doubleup(string(argv[1])); } else { cerr << "Invalid input" << endl; } return 0; } -The init code has already checked different types of input for you. You only need to implement the overloaded version of the DOUBLEUP function. - No more built in libraries allowed apart from those than have been included in the init code Output A decimal number 12, 24 Floating pint number 12.12, 24.24 A binary number 0b111 0b1110 Hexadecimal number 0xAA 0x154
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
C++ You are required to write a universal calculator that performs DOUBLE UP of different types of data. Especially the calculator must take the following types of input.
-
A decimal number
-
A float point number
-
A binary number which must be prefixed with “0b”(e.g 0b111 ob1010 are valid 01b1010, 0b101, 01010 are invalid)
-
A hexadecimal number which must be prefixed with “0x” (e.g. 0x19, 0xAAare valid. 01x1A, ox1A, 01A are invalid)
Note
Your program must implement function overloading. You can consider the examples below for your implementation. You are provided with the initial code which can be found.
#include <iostream>
#include <string>
#include <string.h>
#include <iomanip>
using namespace std;
/*
* IMPLEMENT YOUR OVERLOADED FUNCTIONS HERE
*/
/* this function takes a string and
* returns 1 if the string represents a decimal
* returns 2 if the string represents a floating point
* returns 3 if the string represents a binary
* returns 4 if the string represents a hexadecimal
* return 0 if the input string is anything else
*/
int check_type(char *input) {
int res = 1;
int len = strlen(input);
int i;
// check the first char for special sign chars [-,+] and '.'
char first = input[0];
char second = input[1];
if (first=='-' && first=='+') {
if (second=='.') {
res = 2;
}
}
// got a '.' from the beginning, got a float
else if(first=='.') {
if (second=='.') return 0;
res = 2;
}
//check to see if we got a prefix 0b --> expect a binary
else if (first=='0' && second=='b')
res = 3;
// check to see if we got a prefix 0x --> expect a hexa
else if(first=='0' && second=='x')
res = 4;
else if(first>='0'||first<='9') {
if (second=='.')
res = 2;
}
else if ( (first<'0'||first>'9') && (second<'0'||second>'9') )
return 0;
for (i=2; i<len; i++) {
// already expecting a float, no more '.'
if (res==2) {
if (input[i]=='.' || (input[i]<'0'||input[i]>'9'))
return 0;
}
// already expecting a binary, only '0' or '1'
else if (res==3) {
if (input[i]!='0' && input[i]!='1')
return 0;
}
// already expecting a hexa, only [0-9] or [A-F]
else if (res==4) {
if ( (input[i]<'0'||input[i]>'9') && (input[i]<'A'||input[i]>'F') )
return 0;
}
// still seeing integer, could be a float with a '.'
else if (res==1) {
if (input[i]=='.') res = 2;
else if (input[i]<'0' || input[i]>'9')
return 0;
}
}
return res;
}
int main(int argc, char* argv[]) {
int type;
if (argc!=2) {
cerr << "Wrong number of inputs" << endl;
return 0;
}
type = check_type(argv[1]);
if (type==1) {
doubleup(atoi(argv[1]));
}
else if (type==2) {
doubleup(stof(argv[1]));
}
else if (type==3) {
doubleup(argv[1]);
}
else if (type==4) {
doubleup(string(argv[1]));
}
else {
cerr << "Invalid input" << endl;
}
return 0;
}
-The init code has already checked different types of input for you. You only need to implement the overloaded version of the DOUBLEUP function.
- No more built in libraries allowed apart from those than have been included in the init code
Output
A decimal number 12, 24
Floating pint number 12.12, 24.24
A binary number 0b111 0b1110
Hexadecimal number 0xAA 0x154

Step by step
Solved in 2 steps

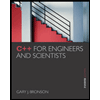
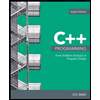
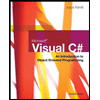
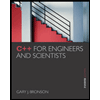
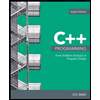
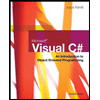