Code language Java 1, Add a print statement to say “Multiplication Table” and then prompt the user to enter an integer. Your program should then prints “times table” for the numbers from 1 to the given integer (inclusive). That is, for input n, you would print a table of n rows (lines) each with n columns, where the cell at row i and column j contains the value i × j. You do need separate rows, but don’t worry about the columns lining up nicely yet. 2. Add a print statement to say “Prime Testing”. Using the integer entered by the user above, print out all the prime numbers between 2 and the number squared. E.g., if the user types 12 for the multiplication table part, you should print all the prime numbers that are not larger than 144. All integers are either prime or composite. A prime number is one that has no integer factors other than itself or one. Examples are 2, 3, 5, 7, 11, 13, . . .. A composite number, by contrast, has multiple factors. Examples are 4, 6, 12, 15, 18, 21, . . .. Testing whether or not a number is prime is an important application of computing power. One very simple method is to simply check every possible factor from 2 up to the number in question. For example, to test whether or not the value 35 is prime, you can test if 35 % 2 == 0, then if 35 % 3 == 0, then is 35 % 4 == 0, and so on, up until you find a number that evenly divides 35 or confirm that no such number exists. You must write this yourself, and cannot use any library methods for primality testing. 3, Add another print statement that says “Infinite Series Test”. Consider the infinite series This time you should prompt the user to enter a second integer k, and then calculates and prints the sum of the first k terms of the sequence. Do not use Math.pow. Instead, compute the value of 2k incrementally in your loop. Try your program for different values of k. Do you notice a trend?
Code language Java
1, Add a print statement to say “Multiplication Table” and then prompt the
user to enter an integer. Your program should then prints “times table” for the
numbers from 1 to the given integer (inclusive). That is, for input n, you would print
a table of n rows (lines) each with n columns, where the cell at row i and column
j contains the value i × j. You do need separate rows, but don’t worry about the
columns lining up nicely yet.
2. Add a print statement to say “Prime Testing”.
Using the integer entered by the user above, print out all the prime numbers between
2 and the number squared. E.g., if the user types 12 for the multiplication table part,
you should print all the prime numbers that are not larger than 144.
All integers are either prime or composite. A prime number is one that has no integer
factors other than itself or one. Examples are 2, 3, 5, 7, 11, 13, . . .. A composite
number, by contrast, has multiple factors. Examples are 4, 6, 12, 15, 18, 21, . . ..
Testing whether or not a number is prime is an important application of computing
power. One very simple method is to simply check every possible factor from 2 up to
the number in question.
For example, to test whether or not the value 35 is prime, you can test if 35 % 2 ==
0, then if 35 % 3 == 0, then is 35 % 4 == 0, and so on, up until you find a number
that evenly divides 35 or confirm that no such number exists. You must write this
yourself, and cannot use any library methods for primality testing.
3, Add another print statement that says “Infinite Series Test”.
Consider the infinite series
This time you should prompt the user to enter a second integer k, and then calculates
and prints the sum of the first k terms of the sequence. Do not use Math.pow. Instead,
compute the value of 2k
incrementally in your loop. Try your program for different
values of k. Do you notice a trend?

Lets see the solution in the next steps
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

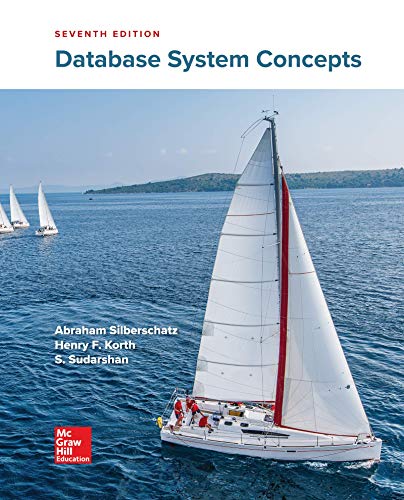
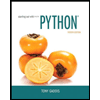
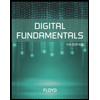
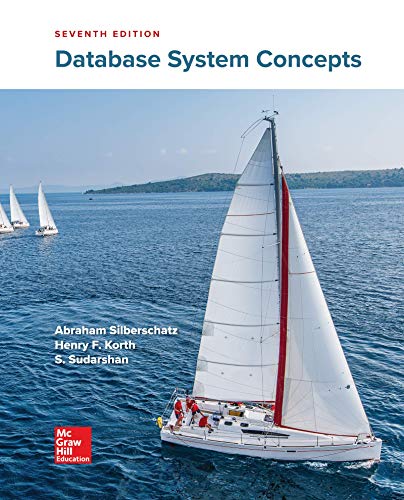
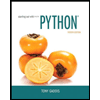
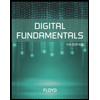
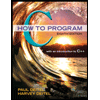
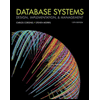
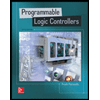