calculate(self) A property method that evaluates the infix expression saved in self.__expr. First convert the expression to postfix, then use a stack to evaluate the output postfix expression. You must use the Stack defined in section 1 in this method, otherwise your code will not receive credit. Input (excluding self) str txt
In python please - all sections (there are 3 in total) except the last 3 pages included in the images, last section included in text below, thank you. Do not change the function names or given starter code in your script. Each class has different requirements, read them carefully and ask so I can comment to clarify. Do not use the exec or eval functions, nor are you allowed to use regular expressions. This includes multiple methods interacting with each other over the course of execution. All methods that output a string must return the string, not print it. If you are unable to complete a method, use the pass statement to avoid syntax errors.
Last 3 pages continued -
calculate(self) A property method that evaluates the infix expression saved in self.__expr. First convert the expression to postfix, then use a stack to evaluate the output postfix expression. You must use the Stack defined in section 1 in this method, otherwise your code will not receive credit. Input (excluding self)
str
txt
The string to check if it represents a number Output
float
The result of the expression
None
None is returned if the input is an invalid expression or if expression can’t be computed
Section 3: The AdvancedCalculator class
The AdvancedCalculator class represents a calculator that supports multiple expressions over many lines, as well as the use of variables. Lines will be split by semicolons (;), and every token will be separated by a single space. Each line will start with a variable name, then an ‘=’ character, then a mathematical expression. The last line will ask to return a mathematical expression too.
You can assume that an expression will not reference variables that have not been defined yet.
You can assume that variable names will be consistent and case sensitive. A valid variable name is a non-empty string of alphanumeric characters, the first of which must be a letter.
You must use a Calculator to evaluate each expression in this class, otherwise, no credit will be given.
Attributes Type Name Description
str
expressions
The expressions this calculator will evaluate
dict
states
A dictionary mapping variable names to their float values
Methods Type Name Description
bool
_isVariable(self, word)
Returns True if word is a valid variable name
str
_replaceVariables(self, expr)
Replaces all variables in an expression with values
dict
calculateExpressions(self)
Calculates all expressions and shows state at each step
>>> C = AdvancedCalculator()
>>> C.setExpression('A = 1;B = A + 9;C = A + B;A = 20;D = A + B + C;return D + 2 * B')
>>> C.states
{}
>>> C.calculateExpressions() # spacing added for readability
{'A = 1': {'A': 1.0},
'B = A + 9': {'A': 1.0, 'B': 10.0},
'C = A + B': {'A': 1.0, 'B': 10.0, 'C': 11.0},
'A = 20': {'A': 20.0, 'B': 10.0, 'C': 11.0},
'D = A + B + C': {'A': 20.0, 'B': 10.0, 'C': 11.0, 'D': 41.0},
'_return_': 61.0}
>>> C.states
{'A': 20.0, 'B': 10.0, 'C': 11.0, 'D': 41.0}
_isVariable(self, word) Determines if the input is a valid variable name (see above for rules for names). The string methods str.isalpha() and str.isalnum() could be helpful here. Input (excluding self)
str
word
The string to check if it is a valid variable name Output
bool
True if word is a variable name, False otherwise
_replaceVariables(self, expr) Replaces all variables in the input expression with the current value of those variables saved in self.states. Input (excluding self)
str
expr
The input expression that may contain variables Output
str
The expression with all variables replaced with their values
None
Nothing is returned if expression has invalid variables or uses a variable that has not been defined
calculateExpressions(self) Evaluates each expression saved in self.expressions. For each line, replace all variables in the expression with their values, then use a Calculator object to evaluate the expression and update self.states. This method returns a dictionary that shows the progression of the calculations, with the key being the line evaluated and the value being the current state of self.states after that line is evaluated. The dictionary must include a key named ‘_return_’ with the return value as its value. Hint: the str.split(sep) method can be helpful for separating lines, as well as separating the variable from the expression (for the lines that are formatted as var = expr). The str.strip() method removes the white space before and after a string. Don’t forget dictionaries are mutable objects! >>> 'hi;there'.split(';') ['hi', 'there'] >>> 'hi=there'.split('=') ['hi', 'there'] >>> ' hi there '.strip() ' hi there' Output
dict
The different states of self.states as the calculation progresses.
None
Nothing is returned if there was an error replacing variables
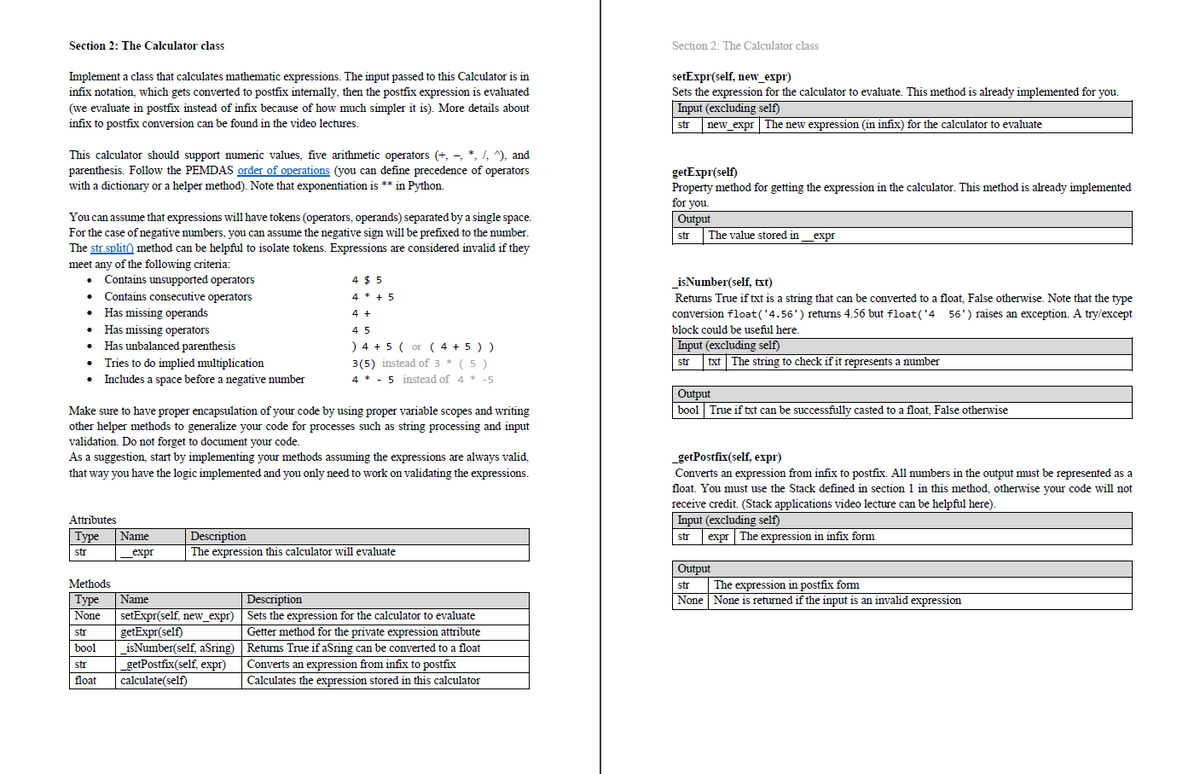
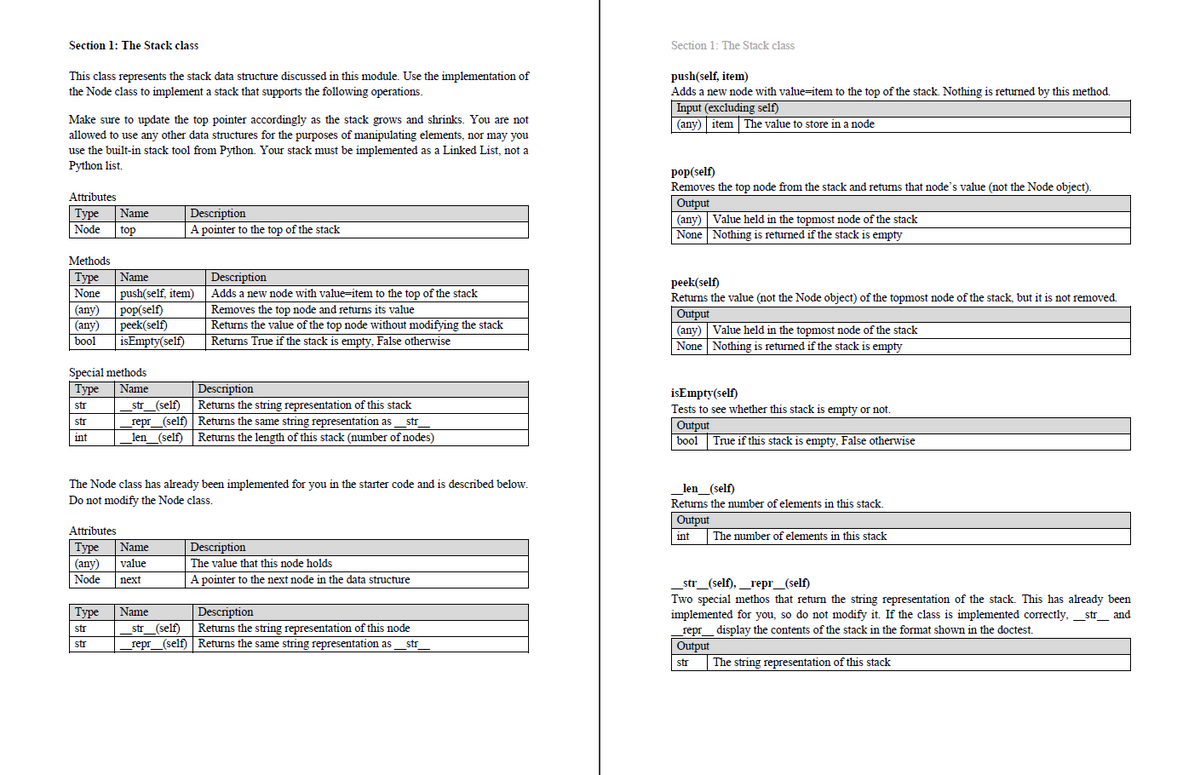

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

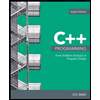
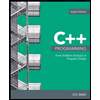