java Singly linked list need help with numbers 5 only 1 to 5 is done but they connected 5 is in the picture 1. Create a class called Citizen with the following attributes/variables: a. String citizenID b. String citizenName c. String citizenSurname d. String citizenCellNumber e. int registrationDay f. int registrationMonth g. int registrationYear 2. Create a class called Node with the following attributes/variables: a. Citizen citizen b. Node nextNode 3. Create a class called CitizenRegister with the following attributes/variables: a. Node headNode b. int totalRegisteredCitizens 4. Add and complete the following methods in CitizenRegister: a. head() i. Returns the first citizen object in the linked list b. tail() i. Returns the last citizen object in the linked list c. size() i. Returns the totalRegisteredCitizen d. isEmpty() i. Returns the boolean of whether the linked list is empty or not e. addCitizenAtHead(Node newNode) i. Adds a new node object containing the citizen object information before the headNode f. addCitizenAtTail(Node newNode) i. Adds a new node object containing the citizen object information at the end of the linked list g. addCitizenBefore(String citizenID, Node newNode) i. Adds a new node object containing the citizen object information before the node with the matching citizenID ii. If such citizen object isn’t found display “Citizen has not registered for vaccine” and add the new node at the end of the linked list h. addCitizenAfter(String citizenID, Node newNode) i. Adds a new node object containing the citizen object information after the node with the matching citizenID ii. If such citizen object isn’t found display “Citizen has not registered for vaccine” and add the new node at the end of the linked list i. removeCitizen(String citizenID) i. Deletes the node object containing the citizen object with the matching citizenID ii. If such citizen object isn’t found display “Citizen has not registered for vaccine” j. removeLastCitizen() i. Deletes the last node object containing the citizen object at the end of the linked list k. removeFirstCitizen() i. Deletes the first node object containing the citizen object in the linked list l. displayAllCitizens() Print the name and surname of all the citizens in the linked list in neatly formatted output: citizenNumber 007 citizenName citizenSurname James Bond
java Singly linked list
need help with numbers 5 only 1 to 5 is done but they connected 5 is in the picture
1. Create a class called Citizen with the following attributes/variables:
a. String citizenID
b. String citizenName
c. String citizenSurname
d. String citizenCellNumber
e. int registrationDay
f. int registrationMonth
g. int registrationYear
2. Create a class called Node with the following attributes/variables:
a. Citizen citizen
b. Node nextNode
3. Create a class called CitizenRegister with the following attributes/variables:
a. Node headNode
b. int totalRegisteredCitizens
4. Add and complete the following methods in CitizenRegister:
a. head()
i. Returns the first citizen object in the linked list
b. tail()
i. Returns the last citizen object in the linked list
c. size()
i. Returns the totalRegisteredCitizen
d. isEmpty()
i. Returns the boolean of whether the linked list is empty or not
e. addCitizenAtHead(Node newNode)
i. Adds a new node object containing the citizen object information
before the headNode
f. addCitizenAtTail(Node newNode)
i. Adds a new node object containing the citizen object information at
the end of the linked list
g. addCitizenBefore(String citizenID, Node newNode)
i. Adds a new node object containing the citizen object information
before the node with the matching citizenID
ii. If such citizen object isn’t found display “Citizen has not registered for
vaccine” and add the new node at the end of the linked list
h. addCitizenAfter(String citizenID, Node newNode)
i. Adds a new node object containing the citizen object information
after the node with the matching citizenID
ii. If such citizen object isn’t found display “Citizen has not registered for
vaccine” and add the new node at the end of the linked list
i. removeCitizen(String citizenID)
i. Deletes the node object containing the citizen object with the
matching citizenID
ii. If such citizen object isn’t found display “Citizen has not registered for
vaccine”
j. removeLastCitizen()
i. Deletes the last node object containing the citizen object at the end
of the linked list
k. removeFirstCitizen()
i. Deletes the first node object containing the citizen object in the linked
list
l. displayAllCitizens()
Print the name and surname of all the citizens in the linked list in
neatly formatted output:
citizenNumber 007
citizenName citizenSurname James Bond
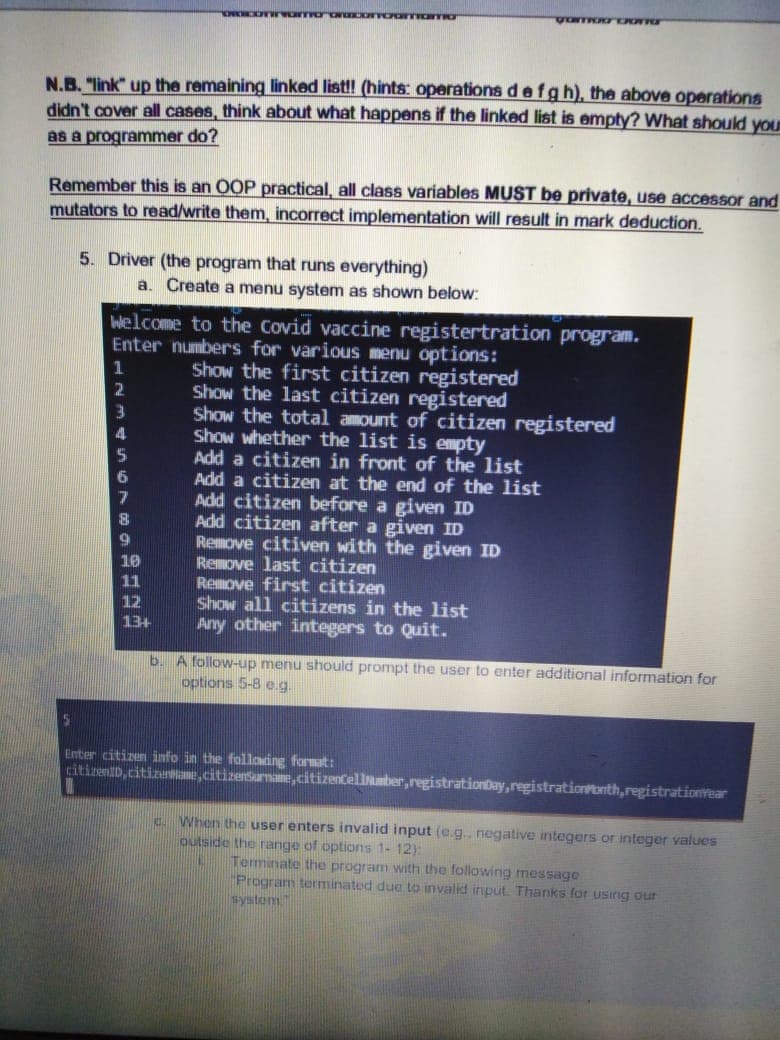

Step by step
Solved in 3 steps with 3 images

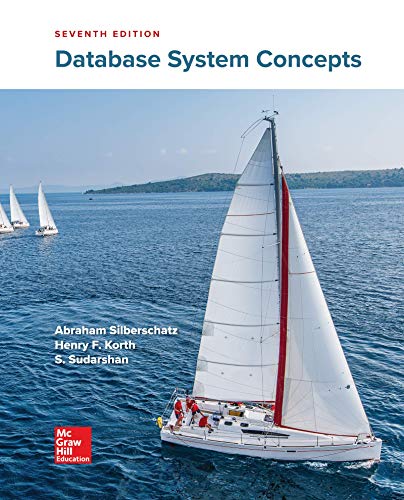
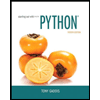
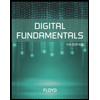
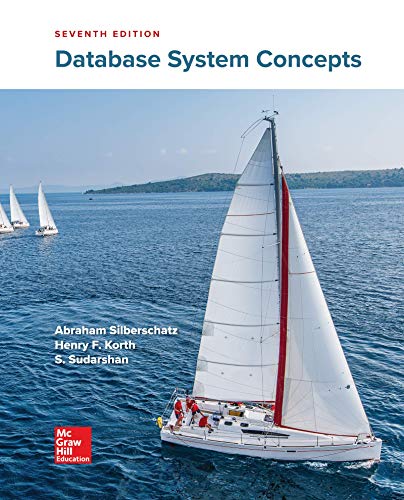
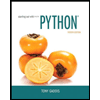
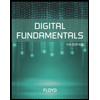
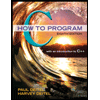
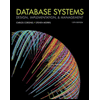
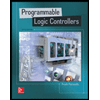