Python’s list method sort includes the keyword argument reverse, whose default value is False. The programmer can override this value to sort a list in descending order. Modify the selectionSort function so that it allows the programmer to supply this additional argument to redirect the sort. selectionSort function: def selectionSort(lyst, profiler): i = 0 while i < len(lyst) - 1: minIndex = i j = i + 1 while j < len(lyst): profiler.comparison() # Count if lyst[j] < lyst[minIndex]: minIndex = j j += 1 if minIndex != i: swap(lyst, minIndex, i, profiler) i += 1 def swap(lyst, i, j, profiler): """Exchanges the elements at positions i and j.""" profiler.exchange() # Count temp = lyst[i] lyst[i] = lyst[j] lyst[j] = temp Use this template in Python to modify the function above: def selection_sort(input_list, reverse): sorted_list = [] #TODO: Your work here # Return sorted_list return sorted_list if __name__ == "__main__": my_list = [1, 2, 3, 4, 5] print(selection_sort(my_list, True)) # Correct Output: [5, 4, 3, 2, 1]
Python’s list method sort includes the keyword argument reverse, whose default
value is False. The programmer can override this value to sort a list in descending
order. Modify the selectionSort function so that it allows
the programmer to supply this additional argument to redirect the sort.
selectionSort function:
def selectionSort(lyst, profiler):
i = 0
while i < len(lyst) - 1:
minIndex = i
j = i + 1
while j < len(lyst):
profiler.comparison() # Count
if lyst[j] < lyst[minIndex]:
minIndex = j
j += 1
if minIndex != i:
swap(lyst, minIndex, i, profiler)
i += 1
def swap(lyst, i, j, profiler):
"""Exchanges the elements at positions i and j."""
profiler.exchange() # Count
temp = lyst[i]
lyst[i] = lyst[j]
lyst[j] = temp
Use this template in Python to modify the function above:
def selection_sort(input_list, reverse):
sorted_list = []
#TODO: Your work here
# Return sorted_list
return sorted_list
if __name__ == "__main__":
my_list = [1, 2, 3, 4, 5]
print(selection_sort(my_list, True)) # Correct Output: [5, 4, 3, 2, 1]

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Can u explain the thought process behind the code ?
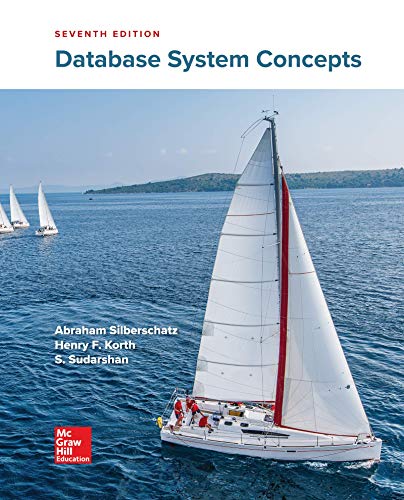
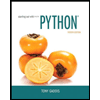
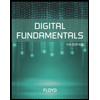
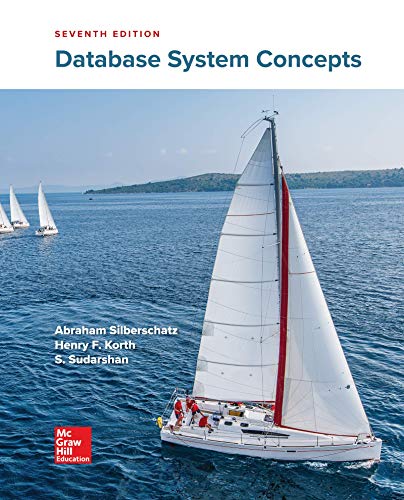
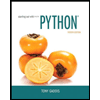
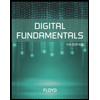
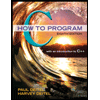
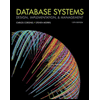
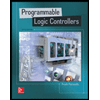