can you fix any errors in this code, it does not producethedesired output import sys import json from collections import deque class Process: def __init__(self, name, runtime, arrival_time, io_frequency): self.name = name self.runtime = runtime self.arrival_time = arrival_time self.io_frequency = io_frequency self.remaining_time = runtime self.last_io_time = 0 def execute(self, current_time): return self.name def io_interrupt(self, current_time): return f'!{self.name}' def MLFQ(data_set): time = 0 queues = [deque() for _ in range(3)] # Three queues for different priority levels schedule = [] while data_set or any(queues): for proc in data_set[:]: if proc.arrival_time <= time: queues[0].append(proc) data_set.remove(proc) for queue in queues: if queue: executed_process = queue.popleft() current_proc_name = executed_process.execute(time) schedule.append(current_proc_name) executed_process.remaining_time -= 1 if executed_process.io_frequency and (time - executed_process.last_io_time) % executed_process.io_frequency == 0: io_interrupt = executed_process.io_interrupt(time) schedule.append(io_interrupt) executed_process.last_io_time = time if executed_process.remaining_time > 0: queues[0].append(executed_process) time += 1 return ' '.join(schedule) def main(): try: with open('config.json', 'r') as config_file: config = json.load(config_file) except FileNotFoundError: print('Error opening the configuration file.') return 1 if len(sys.argv) != 2: print('Usage: python your_script.py input_file_name') return 1 input_file_name = f"Process_List/{config['dataset']}/{sys.argv[1]}" num_processes = 0 data_set = [] try: with open(input_file_name, "r") as file: # Read the number of processes from the file num_processes = int(file.readline().strip()) # Read process data from the file and populate the data_set list for _ in range(num_processes): line = file.readline().strip() name, duration, arrival_time, io_frequency = line.split(',') process = Process(name, int(duration), int(arrival_time), int(io_frequency)) data_set.append(process) except FileNotFoundError: print('Error opening the input file.') return 1 output = MLFQ(data_set) try: output_path = f"Schedulers/template/{config['dataset']}/template_out_{sys.argv[1].split('_')[1]}" with open(output_path, 'w') as output_file: output_file.write(output) except IOError: print('Error writing to the output file.') return 1 return 0 if __name__ == '__main__': exit_code = main()
can you fix any errors in this code, it does not producethedesired output import sys import json from collections import deque class Process: def __init__(self, name, runtime, arrival_time, io_frequency): self.name = name self.runtime = runtime self.arrival_time = arrival_time self.io_frequency = io_frequency self.remaining_time = runtime self.last_io_time = 0 def execute(self, current_time): return self.name def io_interrupt(self, current_time): return f'!{self.name}' def MLFQ(data_set): time = 0 queues = [deque() for _ in range(3)] # Three queues for different priority levels schedule = [] while data_set or any(queues): for proc in data_set[:]: if proc.arrival_time <= time: queues[0].append(proc) data_set.remove(proc) for queue in queues: if queue: executed_process = queue.popleft() current_proc_name = executed_process.execute(time) schedule.append(current_proc_name) executed_process.remaining_time -= 1 if executed_process.io_frequency and (time - executed_process.last_io_time) % executed_process.io_frequency == 0: io_interrupt = executed_process.io_interrupt(time) schedule.append(io_interrupt) executed_process.last_io_time = time if executed_process.remaining_time > 0: queues[0].append(executed_process) time += 1 return ' '.join(schedule) def main(): try: with open('config.json', 'r') as config_file: config = json.load(config_file) except FileNotFoundError: print('Error opening the configuration file.') return 1 if len(sys.argv) != 2: print('Usage: python your_script.py input_file_name') return 1 input_file_name = f"Process_List/{config['dataset']}/{sys.argv[1]}" num_processes = 0 data_set = [] try: with open(input_file_name, "r") as file: # Read the number of processes from the file num_processes = int(file.readline().strip()) # Read process data from the file and populate the data_set list for _ in range(num_processes): line = file.readline().strip() name, duration, arrival_time, io_frequency = line.split(',') process = Process(name, int(duration), int(arrival_time), int(io_frequency)) data_set.append(process) except FileNotFoundError: print('Error opening the input file.') return 1 output = MLFQ(data_set) try: output_path = f"Schedulers/template/{config['dataset']}/template_out_{sys.argv[1].split('_')[1]}" with open(output_path, 'w') as output_file: output_file.write(output) except IOError: print('Error writing to the output file.') return 1 return 0 if __name__ == '__main__': exit_code = main()
Chapter14: Files And Streams
Section: Chapter Questions
Problem 2CP: In Chapter 11, you created the most recent version of the MarshallsRevenue program, which prompts...
Related questions
Question
can you fix any errors in this code, it does not producethedesired output
import sys
import json
from collections import deque
class Process:
def __init__(self, name, runtime, arrival_time, io_frequency):
self.name = name
self.runtime = runtime
self.arrival_time = arrival_time
self.io_frequency = io_frequency
self.remaining_time = runtime
self.last_io_time = 0
def execute(self, current_time):
return self.name
def io_interrupt(self, current_time):
return f'!{self.name}'
def MLFQ(data_set):
time = 0
queues = [deque() for _ in range(3)] # Three queues for different priority levels
schedule = []
while data_set or any(queues):
for proc in data_set[:]:
if proc.arrival_time <= time:
queues[0].append(proc)
data_set.remove(proc)
for queue in queues:
if queue:
executed_process = queue.popleft()
current_proc_name = executed_process.execute(time)
schedule.append(current_proc_name)
executed_process.remaining_time -= 1
if executed_process.io_frequency and (time - executed_process.last_io_time) % executed_process.io_frequency == 0:
io_interrupt = executed_process.io_interrupt(time)
schedule.append(io_interrupt)
executed_process.last_io_time = time
if executed_process.remaining_time > 0:
queues[0].append(executed_process)
time += 1
return ' '.join(schedule)
def main():
try:
with open('config.json', 'r') as config_file:
config = json.load(config_file)
except FileNotFoundError:
print('Error opening the configuration file.')
return 1
if len(sys.argv) != 2:
print('Usage: python your_script.py input_file_name')
return 1
input_file_name = f"Process_List/{config['dataset']}/{sys.argv[1]}"
num_processes = 0
data_set = []
try:
with open(input_file_name, "r") as file:
# Read the number of processes from the file
num_processes = int(file.readline().strip())
# Read process data from the file and populate the data_set list
for _ in range(num_processes):
line = file.readline().strip()
name, duration, arrival_time, io_frequency = line.split(',')
process = Process(name, int(duration), int(arrival_time), int(io_frequency))
data_set.append(process)
except FileNotFoundError:
print('Error opening the input file.')
return 1
output = MLFQ(data_set)
try:
output_path = f"Schedulers/template/{config['dataset']}/template_out_{sys.argv[1].split('_')[1]}"
with open(output_path, 'w') as output_file:
output_file.write(output)
except IOError:
print('Error writing to the output file.')
return 1
return 0
if __name__ == '__main__':
exit_code = main()
sys.exit(exit_code)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
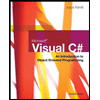
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
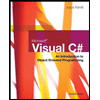
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,