Java Program: There are errors in the lexer and shank file. Please fix those errors and there must be no error in any of the code at all. Below is the lexer, shank, and token files. The shank file is the main method. There is a rubric attached as well.
Java Program:
There are errors in the lexer and shank file. Please fix those errors and there must be no error in any of the code at all. Below is the lexer, shank, and token files. The shank file is the main method. There is a rubric attached as well.
Lexer.java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import mypack.Token.TokenType;
public class Lexer {
private static final int INTEGER_STATE = 1;
private static final int DECIMAL_STATE = 2;
private static final int IDENTIFIER_STATE = 3;
private static final int SYMBOL_STATE = 4;
private static final int ERROR_STATE = 5;
private static final int STRING_STATE = 6;
private static final int CHAR_STATE = 7;
private static final int COMMENT_STATE = 8;
private static final char EOF = (char) -1;
private static String input;
private static int index;
private static char currentChar;
private static int lineNumber = 1;
private static int indentLevel = 0;
private static int lastIndentLevel = 0;
private static HashMap<String, TokenType> keywords = new HashMap<String, TokenType>() {{
put("while", TokenType.WHILE);
put("if", TokenType.IF);
put("else", TokenType.ELSE);
put("print", TokenType.PRINT);
}};
private static HashMap<Character, TokenType> symbols = new HashMap<Character, TokenType>() {{
put('+', TokenType.PLUS);
put('-', TokenType.MINUS);
put('*', TokenType.MULTIPLY);
put('/', TokenType.DIVIDE);
put('=', TokenType.EQUALS);
put(':', TokenType.COLON);
put(';', TokenType.SEMICOLON);
put('(', TokenType.LEFT_PAREN);
put(')', TokenType.RIGHT_PAREN);
put('{', TokenType.LEFT_BRACE);
put('}', TokenType.RIGHT_BRACE);
put('<', TokenType.LESS_THAN);
put('>', TokenType.GREATER_THAN);
}};
public Lexer(String input) {
Lexer.input = input;
index = 0;
currentChar = input.charAt(index);
}
private void nextChar() {
index++;
if (index >= input.length()) {
currentChar = EOF;
} else {
currentChar = input.charAt(index);
}
}
private void skipWhiteSpace() {
while (Character.isWhitespace(currentChar)) {
nextChar();
}
}
private int getIndentLevel() {
int level = 0;
int i = index;
char c = input.charAt(i);
while (c == ' ' || c == '\t') {
if (c == '\t') {
level += 1;
} else if (c == ' ') {
level += 1;
}
i++;
if (i >= input.length()) {
break;
}
Shank.java
package mypack;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
public class Shank {
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Error: Exactly one argument is required.");
System.exit(0);
}
String filename = args[0];
try {
List<String> lines = Files.readAllLines(Paths.get(filename));
for (String line : lines) {
try {
Lexer lexer = new Lexer(line);
List<Token> tokens = lexer.lex(line);
for (Token token : tokens) {
System.out.println(token);
}
} catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
}
}
} catch (IOException e) {
System.out.println("Error: Could not read file '" + filename + "'.");
}
}
}
Token.java
package mypack;
public class Token {
public enum TokenType {
WORD,
NUMBER,
SYMBOL
}
public TokenType tokenType;
private String value;
public Token(TokenType type, String val) {
this.tokenType = type;
this.value = val;
}
public TokenType getTokenType() {
return this.tokenType;
}
public String toString() {
return this.tokenType + ": " + this.value;
}
}
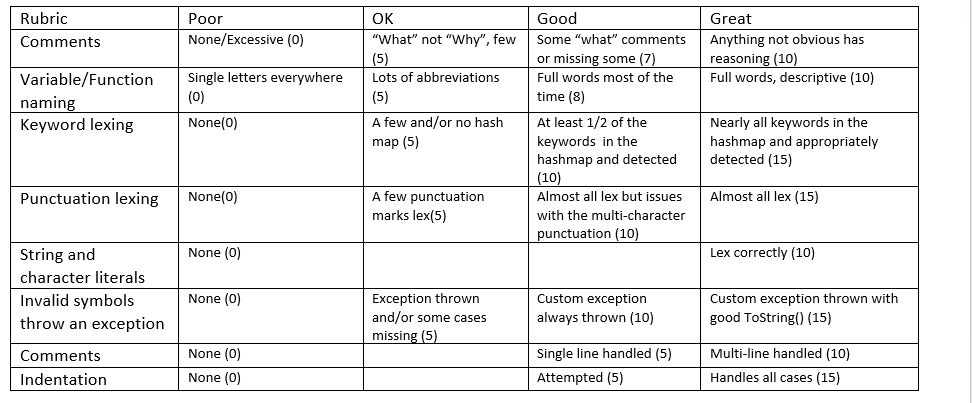

- In the HashMap declaration for the keywords, you can replace the anonymous inner class with a static block.
- In the getIndentLevel method, the variable
level
andi
are never used after being incremented, so there is an infinite loop. - In the same method, you should increment
level
by a specific number of spaces instead of just incrementing by one for each space or tab character. - In the same method, you should break out of the loop when the current character is not a space or tab, not when
i >= input.length()
.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

There are still errors in the lexer and shank files. Please fix those errors and make sure there are no errors in any of the files at all. Attached is an image of the error on the lexer file. There is still an error in the creation of the Lexer object in the main method.
Lexer.java
package mypack;
import java.util.HashMap;
import mypack.Token.TokenType;
public class Lexer {
private static final int INTEGER_STATE = 1;
private static final int DECIMAL_STATE = 2;
private static final int IDENTIFIER_STATE = 3;
private static final int SYMBOL_STATE = 4;
private static final int ERROR_STATE = 5;
private static final int STRING_STATE = 6;
private static final int CHAR_STATE = 7;
private static final int COMMENT_STATE = 8;
private static final char EOF = (char) -1;
private static String input;
private static int index;
private static char currentChar;
private static int lineNumber = 1;
private static int indentLevel = 0;
private static int lastIndentLevel = 0;
private static HashMap<String, TokenType> keywords = new HashMap<>() {{
put("while", TokenType.WHILE);
put("if", TokenType.IF);
put("else", TokenType.ELSE);
put("print", TokenType.PRINT);
}};
private static HashMap<Character, TokenType> symbols = new HashMap<>() {{
put('+', TokenType.PLUS);
put('-', TokenType.MINUS);
put('*', TokenType.MULTIPLY);
put('/', TokenType.DIVIDE);
put('=', TokenType.EQUALS);
put(':', TokenType.COLON);
put(';', TokenType.SEMICOLON);
put('(', TokenType.LEFT_PAREN);
put(')', TokenType.RIGHT_PAREN);
put('{', TokenType.LEFT_BRACE);
put('}', TokenType.RIGHT_BRACE);
put('<', TokenType.LESS_THAN);
put('>', TokenType.GREATER_THAN);
}};
public Lexer(String input) {
Lexer.input = input;
index = 0;
currentChar = input.charAt(index);
}
private void nextChar() {
index++;
if (index >= input.length()) {
currentChar = EOF;
} else {
currentChar = input.charAt(index);
}
}
private void skipWhiteSpace() {
while (Character.isWhitespace(currentChar)) {
nextChar();
}
}
private int getIndentLevel() {
int level = 0;
int i = index;
char c = input.charAt(i);
while (c == ' ' || c == '\t') {
if (c == '\t') {
level += 1;
} else if (c == ' ') {
level += 1;
}
i++;
if (i >= input.length()) {
break;
}
}
return level;
}
}
Shank.java
package mypack;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
public class Shank {
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Error: Exactly one argument is required.");
System.exit(0);
}
String filename = args[0];
try {
List<String> lines = Files.readAllLines(Paths.get(filename));
for (String line : lines) {
try {
Lexer lexer = new Lexer(line);
List<Token> tokens = lexer.lex(line);
for (Token token : tokens) {
System.out.println(token);
}
} catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
}
}
} catch (IOException e) {
System.out.println("Error: Could not read file '" + filename + "'.");
}
}
}
Token.java
package mypack;
public class Token {
public enum TokenType {
WORD,
NUMBER,
SYMBOL
}
public TokenType tokenType;
private String value;
public Token(TokenType type, String val) {
this.tokenType = type;
this.value = val;
}
public TokenType getTokenType() {
return this.tokenType;
}
public String toString() {
return this.tokenType + ": " + this.value;
}
}
![private static final int STRING_STATE = 6;
private static final int CHAR_STATE = 7;
private static final int COMMENT_STATE = 8;
31
private static final char EOF= (char) -1;
32
33 /**The methods below will allow the user to throw an exception and set up the input, index, current
34 *character, line number, indent level, and the last indent level of the lexer file (lines 37-42)
27
28
29
30
35 */
367 38 39 40 44 2 B4U 45 46 F 48 49
41
42
430
44
47
500
51
52
53
54
55
56
57
58
private static String input;
private static int index;
private static char currentChar;
private static int lineNumber = 1;
private static int indentLevel = 0;
private static int lastIndentLevel = 0;
private static HashMap<String, TokenType> keywords = new HashMap<>() {{
put("while", TokenType.WHILE);
put("if", TokenType.IF);
put ("else", TokenType.ELSE);
put("print", TokenType.PRINT);
}};
private static HashMap<Character, TokenType> symbols = new HashMap<>() {{
put('+', TokenType.PLUS);
put('-', TokenType.MINUS);
put('*', TokenType.MULTIPLY);
put('/', TokenType.DIVIDE);
put('=' TokenType. EQUALS);
put(':', TokenType.COLON);
put(';
TokenType.SEMICOLON);
nut('(' Token Tyne LEFT PAREN).
Problems @Javadoc Declaration Console X
<terminated > Shank (1) [Java Application] C:\Users\subri\.p2\pool\plugins\org.eclipse.justj.openjdk.hotspot.jre.full.win32.x86_64_17.0.1.v2021111
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
The method lex (String) is undefined for the type Lexer
at mypack.Shank.main(Shank.java:35)](https://content.bartleby.com/qna-images/question/e7ddc10c-4670-40fd-b02c-6a60c5fcc2f2/906594c1-1100-49dd-9001-9cca0316a52d/olyszi6a_thumbnail.png)
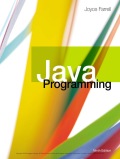
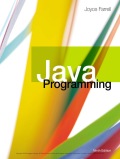