Can you help me with this code because i am struggling and I don't know what to do with this part: he Eight Puzzle consists of a 3 x 3 board of sliding tiles with a single empty space. For each configuration, the only possible moves are to swap the empty tile with one of its neighboring tiles. The goal state for the puzzle consists of tiles 1-3 in the top row, tiles 4-6 in the middle row, and tiles 7 and 8 in the bottom row, with the empty space in the lower-right corner. In this section, you will develop two solvers for a generalized version of the Eight Puzzle, in which the board can have any number of rows and columns. We have suggested an approach similar to the one used to create a Lights Out solver in Homework 2, and indeed, you may find that this pattern can be abstracted to cover a wide range of puzzles. If you wish to use the provided GUI for testing, described in more detail at the end of the section, then your implementation must adhere to the recommended interface. However, this is not required, and no penalty will imposed for using a different approach. A natural representation for this puzzle is a two-dimensional list of integer values between 0 and r · c -1 (inclusive), where r and c are the number of rows and columns in the board, respectively. In this problem, we will adhere to the convention that the 0-tile represents the empty space. this part is the only parts I need help with: In the TilePuzzle class, write a method find_solution_a_star(self) that returns an optimal solution to the current board, represented as a list of direction strings. If multiple optimal solutions exist, any of them may be returned. Your solver should be implemented as an A* search using the Manhattan distance heuristic, which is reviewed below. You may assume that the board is solvable. During your search, you should take care not to add positions to the queue that have already been visited. It is recommended that you use the PriorityQueue class from the Queue module. Recall that the Manhattan distance between two locations (r_1, c_1) and (r_2, c_2) on a board is defined to be the sum of the component wise distances: |r_1 - r_2| + |c_1 - c_2|. The Manhattan distance heuristic for an entire puzzle is then the sum of the Manhattan distances between each tile and its solved location. >>> b = [[4,1,2], [0,5,3], [7,8,6]] >>> p = TilePuzzle(b) >>> p.find_solution_a_star() ['up', 'right', 'right', 'down', 'down'] >>> b = [[1,2,3], [4,0,5], [6,7,8]] >>> p = TilePuzzle(b) >>> p.find_solution_a_star() ['right', 'down', 'left', 'left', 'up', 'right', 'down', 'right', 'up', 'left', 'left', 'down', 'right', 'right']
Can you help me with this code because i am struggling and I don't know what to do with this part:
he Eight Puzzle consists of a 3 x 3 board of sliding tiles with a single empty space. For each configuration, the only possible moves are to swap the empty tile with one of its neighboring tiles. The goal state for the puzzle consists of tiles 1-3 in the top row, tiles 4-6 in the middle row, and tiles 7 and 8 in the bottom row, with the empty space in the lower-right corner.
In this section, you will develop two solvers for a generalized version of the Eight Puzzle, in which the board can have any number of rows and columns. We have suggested an approach similar to the one used to create a Lights Out solver in Homework 2, and indeed, you may find that this pattern can be abstracted to cover a wide range of puzzles. If you wish to use the provided GUI for testing, described in more detail at the end of the section, then your implementation must adhere to the recommended interface. However, this is not required, and no penalty will imposed for using a different approach.
A natural representation for this puzzle is a two-dimensional list of integer values between 0 and r · c -1 (inclusive), where r and c are the number of rows and columns in the board, respectively. In this problem, we will adhere to the convention that the 0-tile represents the empty space.
this part is the only parts I need help with:
In the TilePuzzle class, write a method find_solution_a_star(self) that returns an optimal solution to the current board, represented as a list of direction strings. If multiple optimal solutions exist, any of them may be returned. Your solver should be implemented as an A* search using the Manhattan distance heuristic, which is reviewed below. You may assume that the board is solvable. During your search, you should take care not to add positions to the queue that have already been visited. It is recommended that you use the PriorityQueue class from the Queue module.
Recall that the Manhattan distance between two locations (r_1, c_1) and (r_2, c_2) on a board is defined to be the sum of the component wise distances: |r_1 - r_2| + |c_1 - c_2|. The Manhattan distance heuristic for an entire puzzle is then the sum of the Manhattan distances between each tile and its solved location.
>>> b = [[4,1,2], [0,5,3], [7,8,6]]
>>> p = TilePuzzle(b)
>>> p.find_solution_a_star()
['up', 'right', 'right', 'down', 'down']
>>> b = [[1,2,3], [4,0,5], [6,7,8]]
>>> p = TilePuzzle(b)
>>> p.find_solution_a_star()
['right', 'down', 'left', 'left', 'up', 'right', 'down', 'right', 'up', 'left', 'left', 'down', 'right', 'right']

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

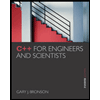
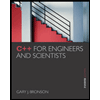