Can you help me with this code because this is a little difficult for me: question that i need help with: The Lights Out puzzle consists of an m x n grid of lights, each of which has two states: on and off. The goal of the puzzle is to turn all the lights off, with the caveat that whenever a light is toggled, its neighbors above, below, to the left, and to the right will be toggled as well. If a light along the edge of the board is toggled, then fewer than four other lights will be affected, as the missing neighbors will be ignored. In this section, you will investigate the behavior of Lights Out puzzles of various sizes by implementing a LightsOutPuzzle class task: A natural representation for this puzzle is a two-dimensional list of Boolean values, where True corresponds to the on state and False corresponds to the off state. In the LightsOutPuzzle class, write an initialization method __init__(self, board) that stores an input board of this form for future use. Also write a method get_board(self) that returns this internal representation. You additionally may wish to store the dimensions of the board as separate internal variables, though this is not required. >>> b = [[True, False], [False, True]] >>> p = LightsOutPuzzle(b) >>> p.get_board() [[True, False], [False, True]] >>> b = [[True, True], [True, True]] >>> p = LightsOutPuzzle(b) >>> p.get_board() [[True, True], [True, True]] Write a top-level function create_puzzle(rows, cols) that returns a new LightsOutPuzzle of the specified dimensions with all lights initialized to the off state. >>> p = create_puzzle(2, 2) >>> p.get_board() [[False, False], [False, False]] >>> p = create_puzzle(2, 3) >>> p.get_board() [[False, False, False], [False, False, False]] In the LightsOutPuzzle class, write a method perform_move(self, row, col) that toggles the light located at the given row and column, as well as the appropriate neighbors. >>> p = create_puzzle(3, 3) >>> p.perform_move(1, 1) >>> p.get_board() [[False, True, False], [True, True, True ], [False, True, False]] >>> p = create_puzzle(3, 3) >>> p.perform_move(0, 0) >>> p.get_board() [[True, True, False], [True, False, False], [False, False, False]] In the LightsOutPuzzle class, write a method scramble(self) which scrambles the puzzle by calling perform_move(self, row, col) with probability 1/2 on each location on the board. This guarantees that the resulting configuration will be solvable, which may not be true if lights are flipped individually. In the LightsOutPuzzle class, write a method is_solved(self) that returns whether all lights on the board are off. >>> b = [[True, False], [False, True]] >>> p = LightsOutPuzzle(b) >>> p.is_solved() False >>> b = [[False, False], [False, False]] >>> p = LightsOutPuzzle(b) >>> p.is_solved() True
Can you help me with this code because this is a little difficult for me:
question that i need help with:
The Lights Out puzzle consists of an m x n grid of lights, each of which has two states: on and off. The goal of the puzzle is to turn all the lights off, with the caveat that whenever a light is toggled, its neighbors above, below, to the left, and to the right will be toggled as well. If a light along the edge of the board is toggled, then fewer than four other lights will be affected, as the missing neighbors will be
ignored. In this section, you will investigate the behavior of Lights Out puzzles of various sizes by implementing a LightsOutPuzzle class
task:
A natural representation for this puzzle is a two-dimensional list of Boolean values, where True corresponds to the on state and False corresponds to the off state. In the LightsOutPuzzle class, write an initialization method __init__(self, board) that stores an input board of this form for future use. Also write a method get_board(self) that returns this internal representation. You additionally may wish to store the dimensions of the board as separate internal variables, though this is not required.
>>> b = [[True, False], [False, True]]
>>> p = LightsOutPuzzle(b)
>>> p.get_board()
[[True, False], [False, True]]
>>> b = [[True, True], [True, True]]
>>> p = LightsOutPuzzle(b)
>>> p.get_board()
[[True, True], [True, True]]
Write a top-level function create_puzzle(rows, cols) that returns a new LightsOutPuzzle of the specified dimensions with all lights initialized to the off state.
>>> p = create_puzzle(2, 2)
>>> p.get_board()
[[False, False], [False, False]]
>>> p = create_puzzle(2, 3)
>>> p.get_board()
[[False, False, False],
[False, False, False]]
In the LightsOutPuzzle class, write a method perform_move(self, row, col) that toggles the light located at the given row and column, as well as the appropriate neighbors.
>>> p = create_puzzle(3, 3)
>>> p.perform_move(1, 1)
>>> p.get_board()
[[False, True, False],
[True, True, True ],
[False, True, False]]
>>> p = create_puzzle(3, 3)
>>> p.perform_move(0, 0)
>>> p.get_board()
[[True, True, False],
[True, False, False],
[False, False, False]]
In the LightsOutPuzzle class, write a method scramble(self) which scrambles the puzzle by calling perform_move(self, row, col) with probability 1/2 on each location on the board. This guarantees that the resulting configuration will be solvable, which may not be true if lights are flipped individually.
In the LightsOutPuzzle class, write a method is_solved(self) that returns whether all lights on the board are off.
>>> b = [[True, False], [False, True]]
>>> p = LightsOutPuzzle(b)
>>> p.is_solved()
False
>>> b = [[False, False], [False, False]]
>>> p = LightsOutPuzzle(b)
>>> p.is_solved()
True

Step by step
Solved in 3 steps with 4 images

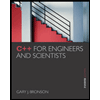
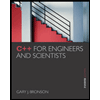