Blackout Math is a math puzzle in which you are given an incorrect arithmetic equation. The goal of the puzzle is to remove two of the digits and/or operators in the equation so that the resulting equation is correct. For example, given the equation 6 - 5 = 15 ^ 4/2 we can remove the digit 5 and the / operator from the right-hand side in order to obtain the correct equality 6 - 5 = 1 ^ 42. Both sides of the equation now equal to 1. Observe how removing an operator between two numbers (4 and 2) causes the digits of the numbers to be concatenated (42). Here is a more complicated example: 288 / 24 x 6 = 18 x 13 x 8 We can remove digits and operators from either side of the equals sign (either both from one side, or one on each side). In this case, we can remove the 2 from the number 24 on the left-hand side and the 1 from the number 13 on the right-hand side to obtain the correct equality 288 / 4 x 6 = 18 x 3 x 8 Both sides of the equation now equal to 432. Here is another puzzle for you to try on your own: 168 / 24 + 8 = 11 x 3 - 16 In this question, we will write Python code to automatically solve a Blackout Math puzzle, and also to create entirely new Blackout Math puzzles. Blackout Math Utility Functions There is a lot to do for this question. Therefore, let us start by creating a module named blackout_utils to store several helper functions which will be useful to us when we implement the full program later on in this question. For full marks, all the following functions must be part of your module. Be careful: functions that take lists as inputs should not modify them. To prevent that from happening, you can create new lists inside the function which are copies of the inputs, and work with those instead. • do_op_for_numbers(op, num1, num2): Takes a string corresponding to a mathematical operation (either '+', '-', 'x', '/' or '^', and two integers, and returns the result of the operation applied to the two numbers as an integer. If the operation is division, the result should be rounded down to the nearest integer. If the operation is division and the result is infinity, return 0 instead. example of how function should be used : >>> do_op_for_numbers('+', 3, 5)
Blackout Math is a math puzzle in which you are given an incorrect arithmetic equation. The goal of the puzzle is to remove two of the digits and/or operators in the equation so that the resulting equation is correct.
For example, given the equation
6 - 5 = 15 ^ 4/2
we can remove the digit 5 and the / operator from the right-hand side in order to obtain the correct
equality
6 - 5 = 1 ^ 42.
Both sides of the equation now equal to 1.
Observe how removing an operator between two numbers (4 and 2) causes the digits of the numbers to be concatenated (42).
Here is a more complicated example:
288 / 24 x 6 = 18 x 13 x 8We can remove digits and operators from either side of the equals sign (either both from one side, or one on each side). In this case, we can remove the 2 from the number 24 on the left-hand side and the 1 from the number 13 on the right-hand side to obtain the correct equality
288 / 4 x 6 = 18 x 3 x 8Both sides of the equation now equal to 432.
Here is another puzzle for you to try on your own:
168 / 24 + 8 = 11 x 3 - 16
In this question, we will write Python code to automatically solve a Blackout Math puzzle, and also to create entirely new Blackout Math puzzles.
Blackout Math Utility Functions
There is a lot to do for this question. Therefore, let us start by creating a module named blackout_utils to store several helper functions which will be useful to us when we implement the full program later on in this question.
For full marks, all the following functions must be part of your module. Be careful: functions that take lists as inputs should not modify them. To prevent that from happening, you can create new lists inside the function which are copies of the inputs, and work with those instead.
• do_op_for_numbers(op, num1, num2): Takes a string corresponding to a mathematical operation (either '+', '-', 'x', '/' or '^', and two integers, and returns the result of the operation applied to the two numbers as an integer. If the operation is division, the result should be rounded down to the nearest integer. If the operation is division and the result is infinity, return 0 instead.
example of how function should be used :
>>> do_op_for_numbers('+', 3, 5)
8

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

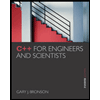
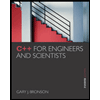