Can you help me with this problem please. Here is my code: #include using namespace std; const double GALLONS_IN_A_CUBIC_FEET = 7.48; class swimmingPool { public: void set(double l = 0, double w = 0, double d = 0, double fi = 0, double fo = 0, double wInPool = 0); void setLength(double l); void setWidth(double w); void setDepth(double d); void setWaterFlowRateIn(double fi); void setWaterFlowRateOut(double fo); void addWater(double time, double fillRate); void drainWater(double time, double drainRate); double poolTotalWaterCapacity(); double getLength(); double getWidth(); double getDepth(); double getWaterFlowRateIn(); double getWaterFlowRateOut(); double getTotalWaterInPool(); int timeToFillThePool(); int timeToDrainThePool(); double waterNeededToFillThePool(); swimmingPool(double l = 0, double w = 0, double d = 0, double fi = 0, double fo = 0, double wInPool = 0); //Constructor private: double length; double width; double depth; double waterFlowInRate; double waterFlowOutRate; double amountOfWaterInPool; }; int main() { swimmingPool pool(20, 30, 10, 5, 2, 0); cout << "Pool dimensions: " << pool.getLength() << "ft x " << pool.getWidth() << "ft x " << pool.getDepth() << "ft" << endl; cout << "Pool water flow in rate: " << pool.getWaterFlowRateIn() << " gallons per minute" << endl; cout << "Pool water flow out rate: " << pool.getWaterFlowRateOut() << " gallons per minute" << endl; cout << "Total water in pool: " << pool.getTotalWaterInPool() << " gallons" << endl; std::cout << "Total water capacity: " << pool.poolTotalWaterCapacity() << " gallons" << endl; pool.addWater(60, 10); cout << "Water added. Total water in pool: " << pool.getTotalWaterInPool() << " gallons" << endl; pool.drainWater(30, 4); cout << "Water drained. Total water in pool: " << pool.getTotalWaterInPool() << " gallons" << endl; int timeToFill = pool.timeToFillThePool(); if (timeToFill >= 0) { cout << "Time to fill the pool completely: " << timeToFill << " minutes" << endl; } else { cout << "Invalid fill rate. Cannot determine time to fill the pool." << endl; } int timeToDrain = pool.timeToDrainThePool(); if (timeToDrain >= 0) { cout << "Time to drain the pool completely: " << timeToDrain << " minutes" << endl; } else { cout << "Invalid drain rate. Cannot determine time to drain the pool." << endl; } double waterNeeded = pool.waterNeededToFillThePool(); cout << "Water needed to fill the pool: " << waterNeeded << " gallons" << endl; return 0; } And here is one of the few issues I am having /root/sandbox6f9a0586/nt-test-126872a3.cpp: In function 'int main(int, char**)': /root/sandbox6f9a0586/nt-test-126872a3.cpp:8:15: error: conflicting declaration of C function 'int main(int, char**)' int main(int argc, char **argv) { ^~~~ In file included from /root/sandbox6f9a0586/nt-test-126872a3.cpp:2:0: /root/sandbox6f9a0586/swimmingPoolImp.cpp:148:5: note: previous declaration 'int main()' int main() { ^~~~ make[2]: *** [CMakeFiles/runTests.dir/nt-test-126872a3.cpp.o] Error 1 make[1]: *** [CMakeFiles/runTests.dir/all] Error 2 make: *** [all] Error 2 I don't know what to do because my actual code works but not in this specific way.
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Can you help me with this problem please. Here is my code:
/root/sandbox6f9a0586/nt-test-126872a3.cpp: In function 'int main(int, char**)': /root/sandbox6f9a0586/nt-test-126872a3.cpp:8:15: error: conflicting declaration of C function 'int main(int, char**)' int main(int argc, char **argv) { ^~~~ In file included from /root/sandbox6f9a0586/nt-test-126872a3.cpp:2:0: /root/sandbox6f9a0586/swimmingPoolImp.cpp:148:5: note: previous declaration 'int main()' int main() { ^~~~ make[2]: *** [CMakeFiles/runTests.dir/nt-test-126872a3.cpp.o] Error 1 make[1]: *** [CMakeFiles/runTests.dir/all] Error 2 make: *** [all] Error 2
I don't know what to do because my actual code works but not in this specific way.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

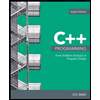
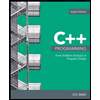