What is a loop?
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Structure of the loop
A loop contains a decision making or a conditional statement and a loop body. The conditional statement contains certain conditions which will direct the sentence present within the curly braces ({ }) to execute till the condition fails.
Purpose of the loops
- Makes the code organized and manageable.
- Repeat the code for certain times.
Types of loops
Based on the position of control or conditional statements in the program, the loops are divided into two different types, they are:
- Entry controlled loop
The conditional statement is checked, and it is essential to execute/perform the body of the loop; thus, the other name for this entry-controlled loop is a pre-checking loop. Example: for and while loop.
2. Exit controlled loop
This checks the loop condition (text expression) after performing the body of the loop. Even if the conditional statement is false, at least for once the block (statement present within the ({…}) curly braces ) will be performed/executed; thus, the other name for this exit-controlled loop is a post-checking loop. Example: do-while loop.
For loop
for loop has the most efficient structure and the syntax as follows,
for (initial statement; test expression; iteration statement)
{ //loop body.
}
Working
The initial statement performs only once to initialize the loop counter value. Evaluate the (condition) test expression until the result is false to conclude the loop. If the result of (condition) test expression becomes true, the block (statement presents within the curly braces ({…})) of the loop gets executed.
Flow chart
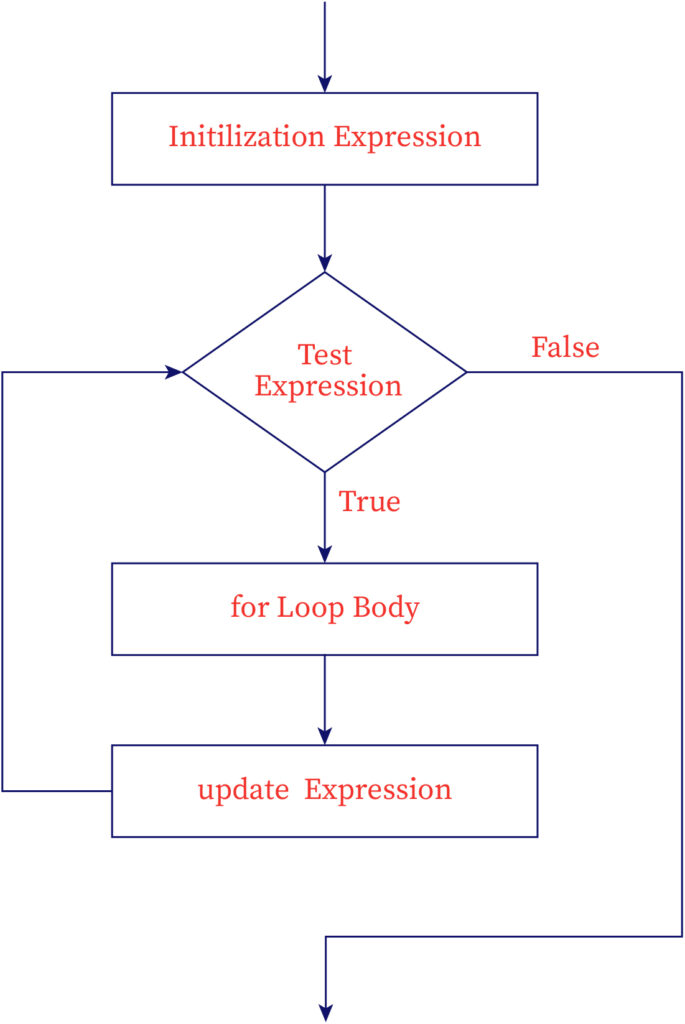
Example
Code
/* Program to display the number starting from 0 - 5 by applying for loop */
#include <stdio.h> //header files
int main() //main function
{
int i; //variable declaration
for (i = 0; i < 6; i++) // initialization of i with 0 to perform for loop
{
printf(“n Printing the number from 0 - 5 n”);
printf(“%d t”, i); //display statement
}
return 0;
}
Output
Printing the number from 0-5
0 1 2 3 4 5
Code explanation
An integer variable i gets initialized as 0. Text expression i < 6 is evaluated and it is true. The block is performed to print 0 ( i value). The statement will get (iteration) increment using i++; i value becomes 1, then the test expression is evaluated again to result as true and the loop gets executed to print 1 ( i value). The text expression i < 6 is checked until the i value becomes 6. If the i = 6, i < 6 results as false and loop gets terminated.
While loop
while loop structure is straightforward and the syntax of the while loop is,
while (test expression)
{
// loop body
}
Working
The test expression present within the parenthesis () is evaluated. If the test expression results as true, the block (statement presents within the ({...}) curly braces) of the loop will be performed and again it evaluates its test expression. The loop process is repeated until the evaluation result is false. Once the result is false, then the loop will get terminated.
Flow chart
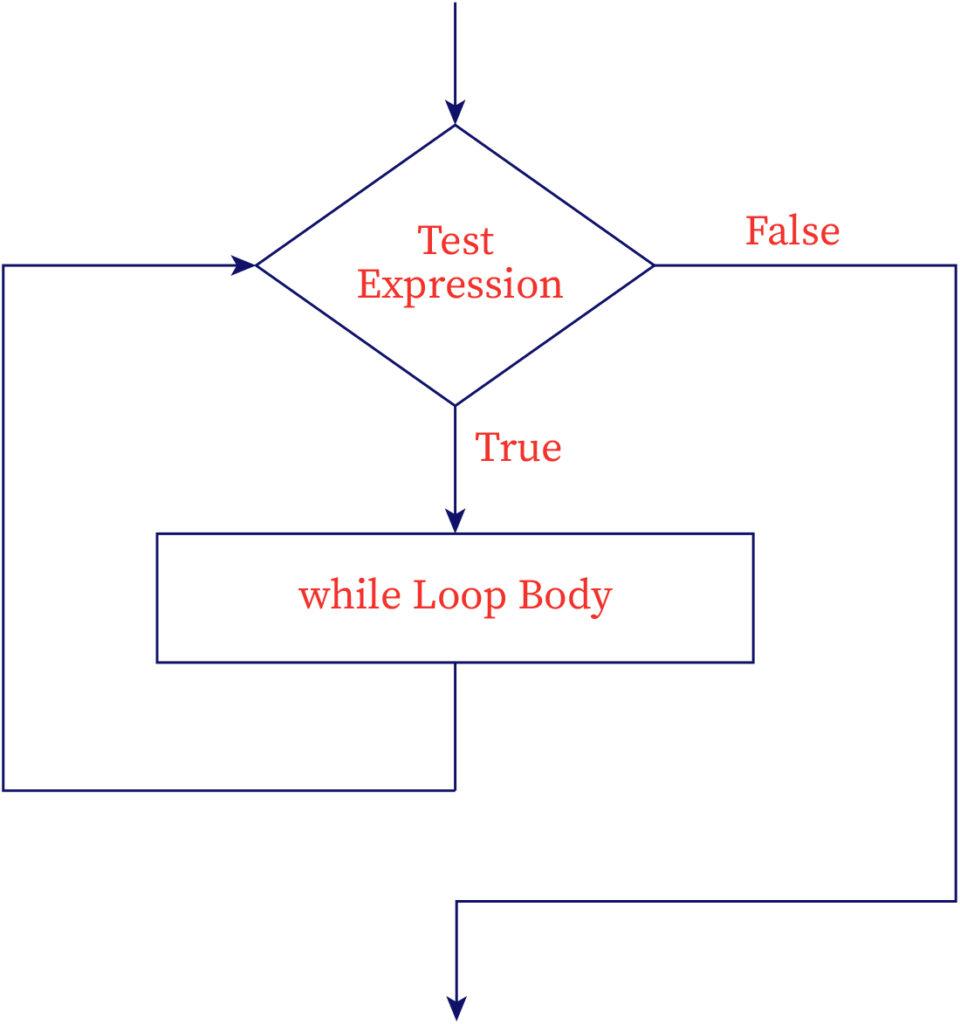
Example
Code
/* Program to print the number starting from 0-5 by applying while loop */
#include <stdio.h> //header files
int main() //main function
{
int i = 0 ; // declaration and initialization of a variable
while (i < 6 )
{
printf(“n Printing the number from 0-5n”);
printf(“%d t”, i); //print statement
i ++; //iteration
}
return 0;
}
Output
Printing the number from 0 - 5
0 1 2 3 4 5
Code explanation
An integer variable i gets initialized as 0. i < 6, the text expression is evaluated and it is true. The loop is performed to print 0 (i value) and increment the i value to 1. Now the i value is 1; i < 6 is evaluated/tested again, astest expression is true, so the loop will be executed to print 1 (i value) and increment the i value to 2. If the i value is 6, then i < 6 is false and ends the loop.
Do-while loop
The do-while loop is similar to the while loop; whereas the condition statement is executed after the block of the do-while loop, and it is also known as an exit-controlled loop. The syntax of the do-while loop is,
do
{
//loop body
} while ( test expression );
Working
Before the evaluation of (condition) test expression, the block (statements which are present within the ({…}) curly braces) will get executed. When the condition (test expression) is true, then the block will be executed again and once more the test expression gets evaluated. The same process of the loop occurs until the (condition) test expression results as false. When the condition result is false, then the loop will get terminated.
Flow chart
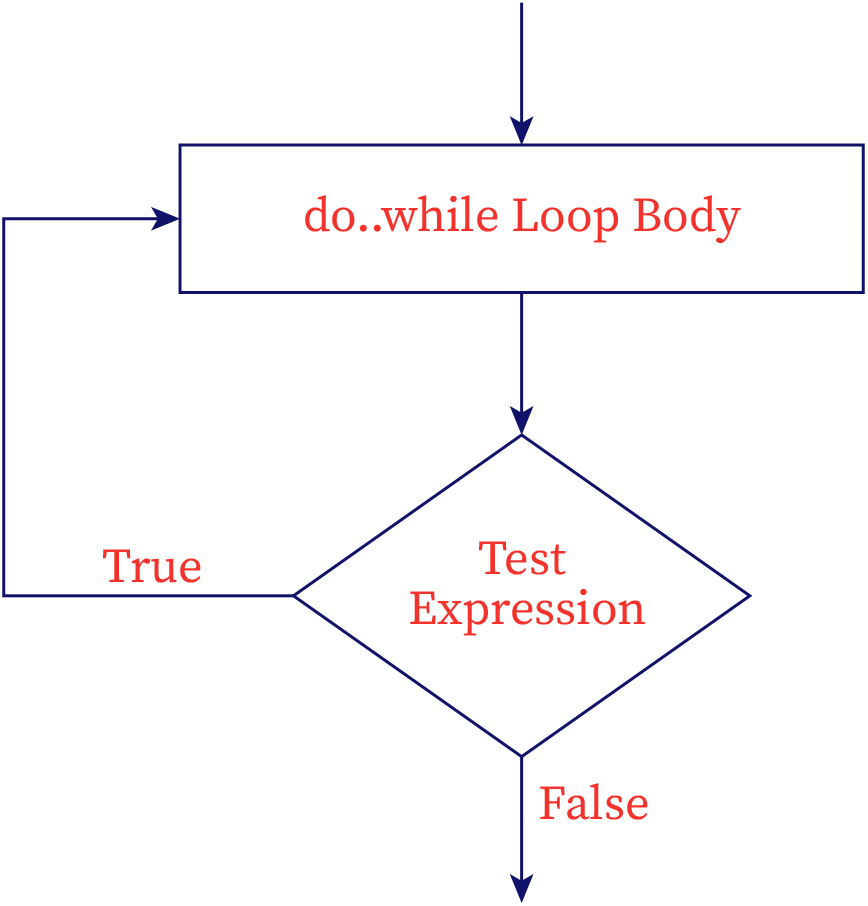
Example
Code
/* Program to display the number starting from 0 to 5 by applying do-while loop */
#include <stdio.h>
int main()
{
int i = 0; // declaration and initialization of a variable
do
{
printf(“n Printing the number from 0 to 5 n ”);
printf(“%d”, i); //display statement
i ++; //iteration
} while (i < 6)
Return 0;
}
Output
Printing the number from 0 - 5
0 1 2 3 4 5
Code explanation
An integer variable i gets initialized as 0. The do-while loop executes the statements before checking the condition. The loop gets executed to print 0 ( i value) and increment the i value to 1. i < 6, text expression gets evaluated and it is true, the block in the loop will execute to print 1 ( i value) and increment the i value. i < 6 text expressions is checked again, as 2 < 6 is true, the loop gets executed to print 2 (i value). If the i value is 6, then i < 6 becomes false and ends the loop.
Nested loops
Nested loops allow the loop statement inside another loop; it allows to define n loops inside another loop; that is no restriction in defining a loop and n refers to the nesting level. Both the inner and outer loop is valid, and it can be for, while, and do-while. The syntax of nested loops is,
Outer-loop
{
Inner-loop
{
//statement for inner-loop
}
//statement for outer-loop
}
Infinite loop
When the loop does not finish its execution and the statement is processed for infinite times, then it is known as an infinite loop and also known as an endless loop. An infinite loop will occur:
- When there is no proper definition for the condition.
- When there is no condition to end the loop.
- When there is no chance to meet the condition.
Context and Applications
This topic is important for postgraduate and undergraduate courses, particularly for, bachelors in computer science engineering and associate of science in computer science.
Practice Problems
Question 1: Which of the following is used to perform certain instructions repeatedly?
a) for
b) while
c) if-else-if
d) both a and b
Answer: Option d is correct.
Explanation: The purpose of the loop is to repeat a set of code/instructions.
Question 2: What will the following code do?
Code:
int main() {
int i = 1;
while (i <= 2)
{
printf( “%d” , i);
}
return 0;
}
a) 12
b) Error
c) infinite loop
d) i
Answer: Option c is correct.
Explanation: In the above code, the while loop will never result in false the output will be an infinite loop.
Question 3: Which of the following loop is implemented in the programming language?
a) For loop
b) While loop
c) do-while loop
d) all the above
Answer: Option d is correct.
Explanation: The programming language makes use of different kinds of loops including for, while, do-while, infinite, and nested loop.
Question 4: The operator ++ in the loop will increment the variable value by ___.
a) 2
b) 1
c) 0
d) depends on the compiler
Answer: Option b is correct.
Explanation: The ++ operator will increment by 1.
Question 5: When will the code block followed by while(i < 10) get executed?
a) when i is greater than ten
b) when i is equal to ten
c) when i is less than ten
d) all the above
Answer: Option c is correct.
Explanation: The block will be executed when i is less than 10.
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Types of Loop Homework Questions from Fellow Students
Browse our recently answered Types of Loop homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.