Can you make C++ code of a Multi-level queue (FCFS, Round Robin, and Priority) for a better understanding of the topic? The explanation of how to make it and the output must be in the image below thank you!
Can you make C++ code of a Multi-level queue (FCFS, Round Robin, and Priority) for a better understanding of the topic? The explanation of how to make it and the output must be in the image below thank you!
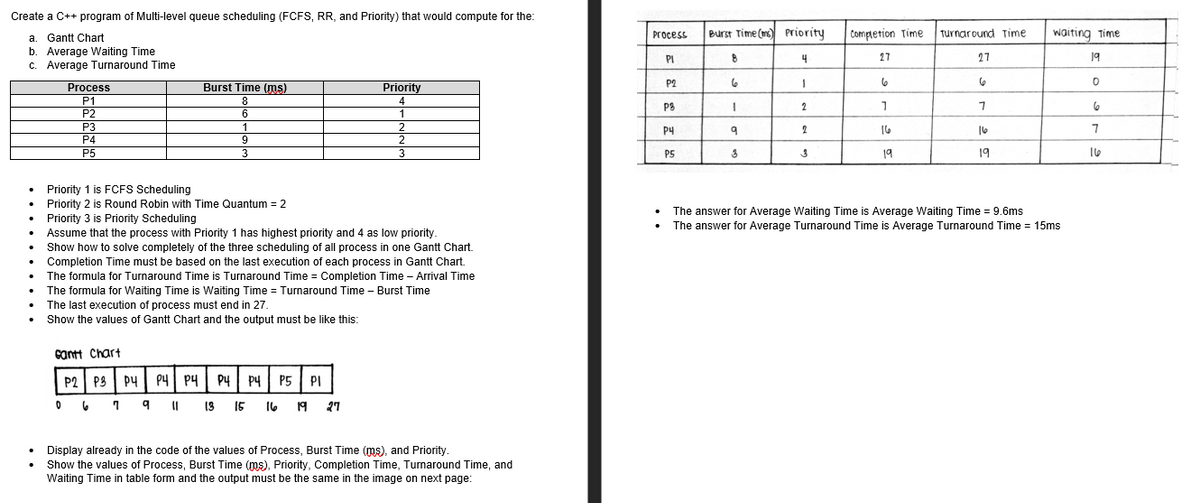

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Can you put the Average Waiting Time and Turnaround Time in the code? Thank you!
Code:
#include <iostream>
#include <queue>
#include <string>
#include <
#include <functional>
struct Process {
int arrivalTime; // Added arrivalTime
int processId;
int burstTime;
int priority;
};
void print_gantt_chart(const std::vector<std::pair<int, int>>& gantt_chart) {
std::cout << "Gantt Chart:" << std::endl;
std::cout << "---------------------------------------------" << std::endl;
std::cout << "| ";
for (const auto& process : gantt_chart) {
std::cout << "P" << process.first << " | ";
}
std::cout << std::endl;
std::cout << "---------------------------------------------" << std::endl;
std::cout << "0";
int currentTime = 0;
for (const auto& process : gantt_chart) {
currentTime += process.second;
if (std::to_string(currentTime).length() == 1)
std::cout << " " << currentTime;
else
std::cout << " " << currentTime;
}
std::cout << std::endl;
}
void fcfsScheduling(std::queue<Process>& fcfsQueue, std::vector<std::pair<int, int>>& gantt_chart) {
while (!fcfsQueue.empty()) {
Process process = fcfsQueue.front();
fcfsQueue.pop();
int executionTime = process.burstTime;
gantt_chart.emplace_back(process.processId, executionTime);
}
}
void rrScheduling(std::queue<Process>& rrQueue, std::vector<std::pair<int, int>>& gantt_chart, int quantumTime) {
while (!rrQueue.empty()) {
Process process = rrQueue.front();
rrQueue.pop();
int executionTime = std::min(quantumTime, process.burstTime);
process.burstTime -= executionTime;
gantt_chart.emplace_back(process.processId, executionTime);
if (process.burstTime > 0)
rrQueue.push(process);
}
}
void priorityScheduling(std::queue<Process>& priorityQueue, std::vector<std::pair<int, int>>& gantt_chart) {
std::priority_queue<Process, std::vector<Process>, std::function<bool(Process, Process)>> pq(
[](const Process& p1, const Process& p2) { return p1.priority > p2.priority; }
);
while (!priorityQueue.empty()) {
pq.push(priorityQueue.front());
priorityQueue.pop();
}
while (!pq.empty()) {
Process process = pq.top();
pq.pop();
int executionTime = process.burstTime;
gantt_chart.emplace_back(process.processId, executionTime);
}
}
void multiLevelQueueScheduling(const std::vector<Process>& processes, int quantumTime) {
std::queue<Process> fcfs;
std::queue<Process> rr;
std::queue<Process> priority;
for (const auto& process : processes) {
if (process.priority == 1)
fcfs.push(process);
else if (process.priority == 2)
rr.push(process);
else if (process.priority == 3 || process.priority == 4)
priority.push(process);
}
std::vector<std::pair<int, int>> gantt_chart;
fcfsScheduling(fcfs, gantt_chart);
rrScheduling(rr, gantt_chart, quantumTime);
priorityScheduling(priority, gantt_chart);
print_gantt_chart(gantt_chart);
std::vector<int> completionTime(processes.size());
std::vector<int> turnaroundTime(processes.size());
std::vector<int> waitingTime(processes.size());
int currentEndTime = 0;
for (const auto& process : gantt_chart) {
int processIndex = process.first - 1;
currentEndTime += process.second;
completionTime[processIndex] = currentEndTime;
turnaroundTime[processIndex] = completionTime[processIndex];
waitingTime[processIndex] = turnaroundTime[processIndex] - processes[processIndex].burstTime;
}
std::cout << "\nProcess\t\tArrival Time\t\tBurst Time\t\tPriority\t\tCompletion Time\t\tTurnaround Time\t\tWaiting Time" << std::endl;
for (const auto& process : processes) {
int processIndex = process.processId - 1;
std::cout << "P" << process.processId << "\t\t" << process.arrivalTime << "\t\t\t" << process.burstTime << "\t\t\t" << process.priority << "\t\t\t"
<< completionTime[processIndex] << "\t\t\t" << turnaroundTime[processIndex] << "\t\t\t" << waitingTime[processIndex] << std::endl;
}
}
int main() {
std::vector<Process> processes = {
{0, 1, 8, 4},
{0, 2, 6, 1},
{0, 3, 1, 2},
{0, 4, 9, 2},
{0, 5, 3, 3}
};
int quantumTime = 2;
multiLevelQueueScheduling(processes, quantumTime);
return 0;
}
Can you put Arrival time in the code if all the arrival time in all processes is 0? Thank you!
Can you align properly the values of Gantt Chart? Thank you!
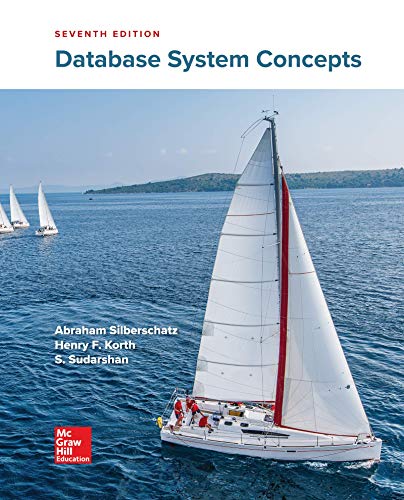
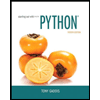
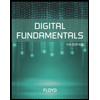
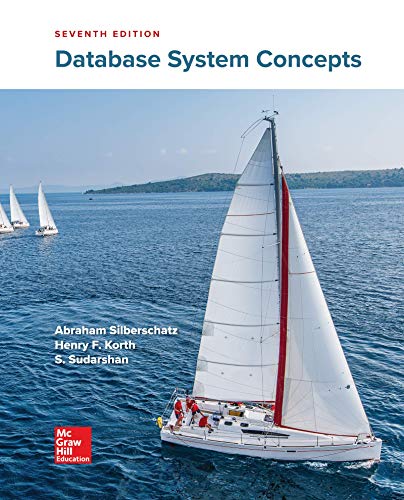
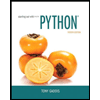
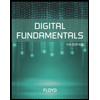
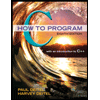
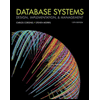
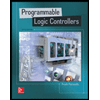