can you produce a lot of logical assumptions for this c programming code?? only words!! #include #include #include void create_inventory(); void update_vacc_qty(); int search_vaccine(); void display_vaccine(); int main() { create_inventory(); display_vaccine(); search_vaccine(); return 0; } void create_inventory() { int option = 1; char vaccinename[15]; char vaccinecode[2]; char country[15]; int dosage; float populaion; FILE* infile; infile = fopen("Vaccine.txt","w"); if (infile == NULL) { printf("Vaccine.txt file unfamiliar\n"); } while (option != 0) { printf("Enter Vaccine Name : "); scanf("%s", vaccinename); printf("Enter Vaccine Code : "); scanf("%s", vaccinecode); printf("Enter Counry Name : "); scanf("%s", country); printf("Enter Dosage Required : "); scanf("%d", &dosage); printf("Enter Population Covered : "); scanf("%f", &populaion); fprintf(infile, "%s %s %s %d %3.2f\n",vaccinename, vaccinecode, country, dosage, populaion); printf("\n press 1 to pursue and 0 to exit : "); scanf("%d", &option); if (option == 0) fclose(infile); } } void display_vaccine() { char vaccinename[15]; char vaccinecode[2]; char country[15]; int dosage; float populaion; FILE* infile; infile = fopen("Vaccine.txt", "r"); if (infile == NULL) { printf("Vaccine.txt file unfamiliar\n"); } printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", "Vaccine Code", "Country", "Dosage", "Population"); while (fscanf(infile, "%s %s %s %d %f\n", vaccinename, vaccinecode, country, &dosage, &populaion) != EOF) { printf("%15s\t%13s\t%15s\t%d\t%3.2f\n", vaccinename, vaccinecode, country, dosage, populaion); } fclose(infile); } int search_vaccine() { char vaccinename[15]; char vaccinecode[2]; char country[15]; int dosage; float populaion; FILE* infile; char vcode[2]; char temp[2]; int value; infile = fopen("Vaccine.txt", "r"); printf("Enter Vaccine Code to explore : "); scanf("%s", vcode); if (infile == NULL) { printf("Vaccine.txt file unfamiliar\n"); } strcpy(temp, vcode); while (fscanf(infile, "%s %s %s %d %f\n", vaccinename, vaccinecode, country, &dosage, &populaion) != EOF) { if (vaccinecode[0] == temp[0] && vaccinecode[1] == temp[1]) { printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", " Vaccine Code", "Country", "Dosage", "Population"); printf("%15s\t%13s\t%15s\t%d\t%3.2f\n", vaccinename, vaccinecode, country, dosage, populaion); } } fclose(infile); }
can you produce a lot of logical assumptions for this c programming code?? only words!!
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void create_inventory();
void update_vacc_qty();
int search_vaccine();
void display_vaccine();
int main()
{
create_inventory();
display_vaccine();
search_vaccine();
return 0;
}
void create_inventory()
{
int option = 1;
char vaccinename[15];
char vaccinecode[2];
char country[15];
int dosage;
float populaion;
FILE* infile;
infile = fopen("Vaccine.txt","w");
if (infile == NULL)
{
printf("Vaccine.txt file unfamiliar\n");
}
while (option != 0)
{
printf("Enter Vaccine Name : ");
scanf("%s", vaccinename);
printf("Enter Vaccine Code : ");
scanf("%s", vaccinecode);
printf("Enter Counry Name : ");
scanf("%s", country);
printf("Enter Dosage Required : ");
scanf("%d", &dosage);
printf("Enter Population Covered : ");
scanf("%f", &populaion);
fprintf(infile, "%s %s %s %d %3.2f\n",vaccinename, vaccinecode, country, dosage, populaion);
printf("\n press 1 to pursue and 0 to exit : ");
scanf("%d", &option);
if (option == 0)
fclose(infile);
}
}
void display_vaccine()
{
char vaccinename[15];
char vaccinecode[2];
char country[15];
int dosage;
float populaion;
FILE* infile;
infile = fopen("Vaccine.txt", "r");
if (infile == NULL)
{
printf("Vaccine.txt file unfamiliar\n");
}
printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", "Vaccine Code", "Country", "Dosage", "Population");
while (fscanf(infile, "%s %s %s %d %f\n", vaccinename, vaccinecode, country, &dosage, &populaion) != EOF)
{
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n", vaccinename, vaccinecode, country, dosage, populaion);
}
fclose(infile);
}
int search_vaccine()
{
char vaccinename[15];
char vaccinecode[2];
char country[15];
int dosage;
float populaion;
FILE* infile;
char vcode[2];
char temp[2];
int value;
infile = fopen("Vaccine.txt", "r");
printf("Enter Vaccine Code to explore : ");
scanf("%s", vcode);
if (infile == NULL)
{
printf("Vaccine.txt file unfamiliar\n");
}
strcpy(temp, vcode);
while (fscanf(infile, "%s %s %s %d %f\n", vaccinename, vaccinecode, country, &dosage, &populaion) != EOF)
{
if (vaccinecode[0] == temp[0] && vaccinecode[1] == temp[1])
{
printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", " Vaccine Code", "Country", "Dosage", "Population");
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n", vaccinename, vaccinecode, country, dosage, populaion);
}
}
fclose(infile);
}

Step by step
Solved in 2 steps

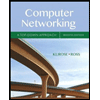
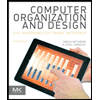
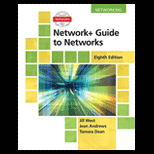
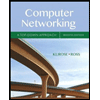
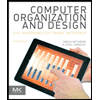
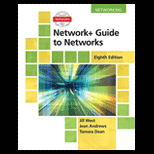
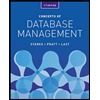
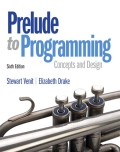
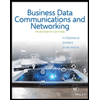