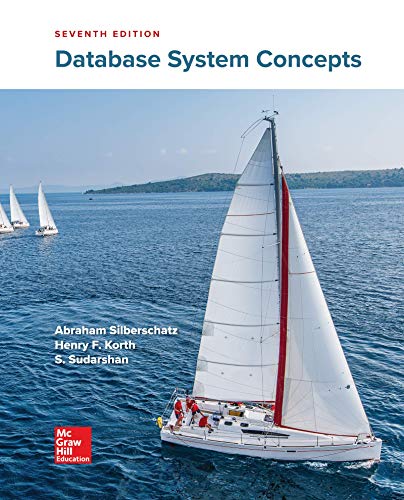
Concept explainers
Please help me with this code, Im am confused adn dont know where to start.
Develop a high-quality, menu-driven object-oriented C++ program that creates a small
The C++ object-oriented program must use the BinarySearchTree class When designing and implementing the program, apply good software engineering principles.
Your program must include:
- a Film class that stores all the data for a Film and provides appropriate methods to support good software engineering principles,
- a FilmDatabase class that stores the binary search tree and provides appropriate methods in support of database queries and reporting using good software engineering principles,
- an application that interacts with the end-user. The design is up to you and may include any number of classes in support of the given application. A Menu class is strongly recommended.
Note that the binary search tree must store Film objects, and the >, <, and == operators must be defined for that class because the BinarySearchTree class uses those overloaded operators.
The downloaded files include the Billionaire class (Billionaire.h and Billionaire.cpp), the BillionaireDatabase class (BillionaireDS.h and BillionaireDS.cpp), main.cpp, and makefile for you to use as an example to help you begin your program. The program compiles, links, and executes if you Make it using NetBeans.
Data Details:
The database contains data pertaining to the 100 highest grossing films of 2017. A comma delimited file named Films2017.csv contains the initial data. Each record is stored on one line of the file in the following format:
Data | Data type |
Rank | int |
Film Title (key) | string |
Studio | string |
Total Gross | double |
Total Theaters | int |
Opening Gross | double |
Opening Theaters | int |
Opening Date | string |
Each of the data fields is separated in the file using the comma (,) character as a delimiter. There is no comma (,) character after the last field on the line. The data is considered clean; There are no errors in the input file.
When storing the data in the binary search tree, use the data types shown above. The Film Title will serve as the key field. Therefore, an inorder traversal of the BST will produce the Films in order of title.
Menu Details:
Your application must be menu driven. The menu system consists of a main menu and sub-menus. All menu choices are selected by entering the letter of the desired choice. After a selection is processed, the current menu should be re-displayed. Do NOT use recursion to do this; use a loop. The current menu continues until the X option (return to main menu or exit) is selected.
When the application begins, the following main menu should be displayed:
MAIN MENU
A - About the Application
R - Reports
S - Search the Database
X - Exit the Program
Enter Selection ->
A - About the Application
If the end-user chooses About the Application, the program provides a detailed description for the user, explaining what the application is doing and how it works. Note that this method does NOT substitute for javadoc-style comments. The audience for this method consists of non-technical users that have no information at all about the assignment.
R - Reports
If the end-user chooses Reports from the MAIN MENU, the program displays the following sub-menu:
REPORTS MENU
T - Order by Film Title report
R - Order by Rank report
X - Return to main menu
Enter Selection ->
If the end-user chooses Order by Film Title report from the REPORTS MENU, the program should do the following:
- Display a report containing all 100 Films in order by Film Title. This report should contain all the data for each record stored in the binary search tree. The data should be formatted; Dollar figures and numeric data should include $ signs and commas for readability.
- Identify the report and label all the data displayed appropriately.
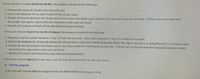
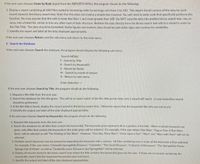

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- code in ada with Ada.Numerics.Generic_Elementary_Functions; package body Number_Theory is -- Instantiate the library for floating point math using Floating_Type. package Floating_Functions is new Ada.Numerics.Generic_Elementary_Functions(Floating_Type); use Floating_Functions; function Factorial(N : in Factorial_Argument_Type) return Positive is begin -- TODO: Finish me! -- -- 0! is 1 -- N! is N * (N-1) * (N-2) * ... * 1 return 1; end Factorial; function Is_Prime(N : in Prime_Argument_Type) return Boolean is Upper_Bound : Prime_Argument_Type; Current_Divisor : Prime_Argument_Type; begin -- Handle 2 as a special case. if N = 2 then return True; end if; Upper_Bound := N - 1; Current_Divisor := 2; while Current_Divisor < Upper_Bound loop if N rem Current_Divisor = 0 then return False; end if; Upper_Bound := N / Current_Divisor; end loop;…arrow_forwardLanguage is C++ Lab14A: The Architect. Buildings can be built in many ways. Usually, the architect of the building draws up maps and schematics of a building specifying the building’s characteristics such as how tall it is, how many stories it has etc. Then the actual building itself is built based on the schematics (also known as blueprints). Now it is safe to assume that the actual building is based off the blueprint but is not the blueprint itself and vice versa. The idea of a classes and objects follows a similar ideology. The class file can be considered the blueprint and the object is the building following the analogy mentioned above. The class file contains the details of the object i.e., the object’s attributes (variables) and behavior (methods). Please keep in mind that a class is a template of an eventual object. Although the class has variables, these variables lack an assigned value since each object will have a unique value for that variable. Think of a form that you…arrow_forwardPlease explain this C++ program, it doesn't have to be long as long as you explain the important parts of the code do. You can explain it line by line and I will give you a good rating. Thank you Activity name: Trinode Restructuring #include "node.h"#include <iostream>using namespace std;class BSTree { node* root; int size; node* create_node(int num, node* parent) { node* n = (node*) malloc( sizeof(node) ); n->element = num; n->parent = parent; n->right = NULL; n->left = NULL; return n; } bool search(node* curr, int num) { if (curr == NULL) { return false; } if (num == curr->element) { return true; } if (num < curr->element) { return search(curr->left, num); } return search(curr->right, num); } node* search_node(node* curr, int num) { if (num == curr->element) { return curr;…arrow_forward
- the code is in ada with Ada.Numerics.Generic_Elementary_Functions; package body Number_Theory is -- Instantiate the library for floating point math using Floating_Type. package Floating_Functions is new Ada.Numerics.Generic_Elementary_Functions(Floating_Type); use Floating_Functions; function Factorial(N : in Factorial_Argument_Type) return Positive is begin -- TODO: Finish me! -- -- 0! is 1 -- N! is N * (N-1) * (N-2) * ... * 1 return 1; end Factorial; function Is_Prime(N : in Prime_Argument_Type) return Boolean is Upper_Bound : Prime_Argument_Type; Current_Divisor : Prime_Argument_Type; begin -- Handle 2 as a special case. if N = 2 then return True; end if; Upper_Bound := N - 1; Current_Divisor := 2; while Current_Divisor < Upper_Bound loop if N rem Current_Divisor = 0 then return False; end if; Upper_Bound := N / Current_Divisor; end loop;…arrow_forwardEvery data structure that we use in computer science has its weaknesses and strengthsHaving a full understanding of each will help make us better programmers!For this experiment, let's work with STL vectors and STL dequesFull requirements descriptions are found in the source code file Part 1Work with inserting elements at the front of a vector and a deque (30%) Part 2Work with inserting elements at the back of a vector and a deque (30%) Part 3Work with inserting elements in the middle, and removing elements from, a vector and a deque (40%) Please make sure to put your code specifically where it is asked for, and no where elseDo not modify any of the code you already see in the template file This C++ source code file is required to complete this problemarrow_forwardIncorporate some data structures in this code.arrow_forward
- In C++ programming. Below is the UAH_sample.txt The University of Alabama in Huntsville was founded in 1950 and became an autonomous campus with The University of Alabama System in 1969. Since that time, it has grown into one of the nation's premiere research universities, offering a challenging hands on curriculum that ensures our graduates are prepared to become tomorrow's leaders. Why GO to UAHuntsville? Our beautiful 400 acre campus is in the heart of Huntsville, Alabama. Also known as the Rocket City, Huntsville has all the perks of nearby big cities like Nashville and Atlanta but with less traffic and a lower cost of living. Not to mention, we enjoy a mild climate perfect for year round outdoor activities and entertainment. And right across the street from campus are a number of major federal and industry employers like Redstone Arsenal, NASA, and Cummings Research Park. So it's no wonder Huntsville was recently ranked in the top ten of Money Magazine's list of "Where the jobs…arrow_forwardYou are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. The program has to be in c++, and have to use the already started code below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in…arrow_forwardHow to use C# for Program 1’s language. This program will prompt the user for a movie title and the category it will be stored in within the access database. For Program 2, how to use C++. This program will query the database to search for a film within a specified category and then display the results. The categories consist of Action, Mafia, Comedy, Drama, Documentary, and Horror. Planning to use Access as the database for connecting both languages. And cannot figure out how to connect the database.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
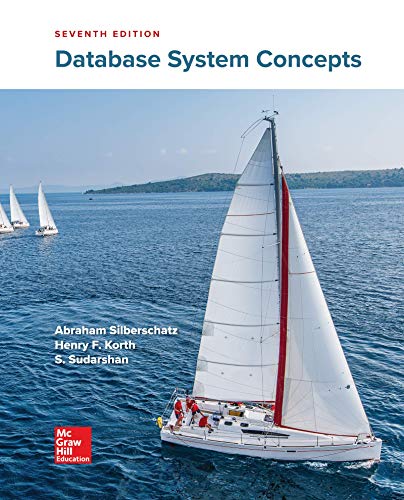
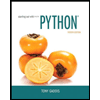
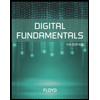
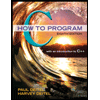
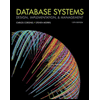
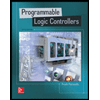