c# Now, modify the program so that the major functions appear in the following individual methods: GetMonth - This method prompts for and returns the month GetNumMurals - This method prompts for and returns the number of murals scheduled and is called twice -- once for interior murals and once for exterior murals ComputeRevenue - This method accepts the number of interior and exterior murals scheduled, accepts the month they are scheduled, displays the interior and exterior prices, and then returns the total expected revenue DataEntry - This method fills an array with customer names and mural codes and is called twice -- once to fill the array of interior murals and once to fill the array of exterior murals GetSelectedMurals - This method continuously prompts for mural codes and displays jobs of the corresponding type until a sentinel value is entered
c# Now, modify the program so that the major functions appear in the following individual methods: GetMonth - This method prompts for and returns the month GetNumMurals - This method prompts for and returns the number of murals scheduled and is called twice -- once for interior murals and once for exterior murals ComputeRevenue - This method accepts the number of interior and exterior murals scheduled, accepts the month they are scheduled, displays the interior and exterior prices, and then returns the total expected revenue DataEntry - This method fills an array with customer names and mural codes and is called twice -- once to fill the array of interior murals and once to fill the array of exterior murals GetSelectedMurals - This method continuously prompts for mural codes and displays jobs of the corresponding type until a sentinel value is entered
Chapter7: Using Methods
Section: Chapter Questions
Problem 2CP
Related questions
Question
c# Now, modify the program so that the major functions appear in the following individual methods:
- GetMonth - This method prompts for and returns the month
- GetNumMurals - This method prompts for and returns the number of murals scheduled and is called twice -- once for interior murals and once for exterior murals
- ComputeRevenue - This method accepts the number of interior and exterior murals scheduled, accepts the month they are scheduled, displays the interior and exterior prices, and then returns the total expected revenue
- DataEntry - This method fills an array with customer names and mural codes and is called twice -- once to fill the array of interior murals and once to fill the array of exterior murals
- GetSelectedMurals - This method continuously prompts for mural codes and displays jobs of the corresponding type until a sentinel value is entered
using systeml
using static System.Console;
using System.Globalization;
class MarshallsRevenue
{
staticvoidMain()
{
// Your code here
constint INTERIOR_PRICE =500;
constint EXTERIOR_PRICE =750;
constint DISCOUNT_INTERIOR_PRICE =450;
constint DISCOUNT_EXTERIOR_PRICE =699;
constint MIN_MURALS =0;
constint MAX_MURALS =30;
string entryString;
int month;
int numInterior;
int numExterior;
int revenueInterior;
int revenueExterior;
int total;
bool isInteriorGreater;
string[] interiorCustomers = new string[MAX_MURALS];
string[] exteriorCustomers = new string[MAX_MURALS];
char[] muralCodes = {'L','S','A','C','O'};
string[] muralCodeStrings = {"Landscape", "Seascape", "Abstract", "Children's", "Other"};
char[] interiorCodes =newchar[MAX_MURALS];
char[] exteriorCodes =newchar[MAX_MURALS];
int x;
int pos =0;
bool isValid;
bool found;
int[] interiorCounts = {0,0,0,0,0};
int[] exteriorCounts = {0,0,0,0,0};
char option;
constchar QUIT ='Z';
Write("Enter the month >> ");
entryString = ReadLine();
month = Convert.ToInt32(entryString);
while(month <1|| month >12)
{
WriteLine("Invalid month. Enter the month >>");
entryString = ReadLine();
month = Convert.ToInt32(entryString);
}
Write("Enter number of interior murals scheduled >> ");
entryString = ReadLine();
numInterior = Convert.ToInt32(entryString);
while(numInterior < MIN_MURALS || numInterior > MAX_MURALS)
{
WriteLine("Number must be between {0} and {1} inclusive", MIN_MURALS, MAX_MURALS);
Write("Enter number of interior murals scheduled >> ");
entryString = ReadLine();
numInterior = Convert.ToInt32(entryString);
}
Write("Enter number of exterior murals scheduled >> ");
entryString = ReadLine();
numExterior = Convert.ToInt32(entryString);
while(numExterior < MIN_MURALS || numExterior > MAX_MURALS)
{
WriteLine("Number must be between {0} and {1} inclusive", MIN_MURALS, MAX_MURALS);
Write("Enter number of exterior murals scheduled >> ");
entryString = ReadLine();
numExterior = Convert.ToInt32(entryString);
}
if(month ==12|| month ==1|| month ==2)
numExterior = 0;
if(month ==4|| month ==5|| month ==9|| month ==10)
revenueExterior = numExterior * DISCOUNT_EXTERIOR_PRICE;
else
revenueExterior = numExterior * EXTERIOR_PRICE;
if(month ==7|| month ==8)
revenueInterior = numInterior * DISCOUNT_INTERIOR_PRICE;
else
revenueInterior = numInterior * INTERIOR_PRICE;
total = revenueInterior + revenueExterior;
isInteriorGreater = numInterior > numExterior;
WriteLine("{0} interior murals are scheduled at {1} each for a total of {2}",
numInterior, INTERIOR_PRICE.ToString("C", CultureInfo.GetCultureInfo("en-US")), revenueInterior.ToString("C",
CultureInfo.GetCultureInfo("en-US")));
WriteLine("{0} exterior murals are scheduled at {1} each for a total of {2}",
numExterior, EXTERIOR_PRICE.ToString("C", CultureInfo.GetCultureInfo("en-US")), revenueExterior.ToString("C",
CultureInfo.GetCultureInfo("en-US")));
WriteLine("Total revenue expected is {0}", total.ToString("C", CultureInfo.GetCultureInfo("en-US")));
WriteLine("It is {0} that there are more interior murals scheduled than exterior ones", isInteriorGreater);
WriteLine("Enter interior jobs:");
x = 0;
while(x < numInterior)
{
Write("Enter customer name >> ");
interiorCustomers[x] = ReadLine();
WriteLine("Mural options are: ");
for(int y =0; y < muralCodes.Length; ++y)
WriteLine(" {0} {1}", muralCodes[y], muralCodeStrings[y]);
Write(" Enter mural style code >> ");
interiorCodes[x] = Convert.ToChar(ReadLine());
isValid = false;
while(!isValid)
{
for(int z =0; z < muralCodes.Length; ++z)
{
if(interiorCodes[x] == muralCodes[z])
{
isValid = true;
++interiorCounts[z];
}
}
if(!isValid)
{
WriteLine("{0} is not a valid code", interiorCodes[x]);
Write(" Enter mural style code >> ");
interiorCodes[x] = Convert.ToChar(ReadLine());
}
}
++x;
}
WriteLine("Enter exterior job: ");
x = 0;
while(x < numExterior)
{
Write("Enter customer name >> ");
exteriorCustomers[x] = ReadLine();
WriteLine("Mural options are: ");
for(int y =0; y < muralCodes.Length; ++y)
WriteLine(" {0} {1}", muralCodes[y], muralCodeStrings[y]);
Write(" Enter mural style code >> ");
exteriorCodes[x] = Convert.ToChar(ReadLine());
isValid = false;
while(!isValid)
{
for(int z =0; z < muralCodes.Length; ++z)
{
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
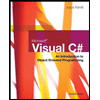
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
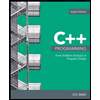
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
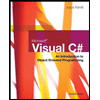
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
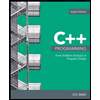
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning