Section 1: The Stack class This class represents the stack data structure discussed in this module. Use the implementation of the Node class to implement a stack that supports the following operations. Make sure to update the top pointer accordingly as the stack grows and shrinks. You are not allowed to use any other data structures for the purposes of manipulating elements, nor may you use the built-in stack tool from Python. Your stack must be implemented as a Linked List, not a Python list. Attributes Type Name Description Node top A pointer to the top of the stack Methods Type Name Description None push(self, item) Adds a new node with value=item to the top of the stack (any) pop(self) Removes the top node and returns its value (any) peek(self) Returns the value of the top node without modifying the stack bool isEmpty(self) Returns True if the stack is empty, False otherwise Special methods Type Name Description str __str__(self) Returns the string representation of this stack str __repr__(self) Returns the same string representation as __str__ int __len__(self) Returns the length of this stack (number of nodes) The Node class has already been implemented for you in the starter code and is described below. Do not modify the Node class. Attributes Type Name Description (any) value The value that this node holds Node next A pointer to the next node in the data structure Type Name Description str __str__(self) Returns the string representation of this node str __repr__(self) Returns the same string representation as __str__3 push(self, item) Adds a new node with value=item to the top of the stack. Nothing is returned by this method. Input (excluding self) (any) item The value to store in a node pop(self) Removes the top node from the stack and returns that node’s value (not the Node object). Output (any) Value held in the topmost node of the stack None Nothing is returned if the stack is empty peek(self) Returns the value (not the Node object) of the topmost node of the stack, but it is not removed. Output (any) Value held in the topmost node of the stack None Nothing is returned if the stack is empty isEmpty(self) Tests to see whether this stack is empty or not. Output bool True if this stack is empty, False otherwise __len__(self) Returns the number of elements in this stack. Output int The number of elements in this stack __str__(self), __repr__(self) Two special methos that return the string representation of the stack. This has already been implemented for you, so do not modify it. If the class is implemented correctly, __str__ and __repr__ display the contents of the stack in the format shown in the doctest. Output str The string representation of this stack
Section 1: The Stack class This class represents the stack data structure discussed in this module. Use the implementation of the Node class to implement a stack that supports the following operations. Make sure to update the top pointer accordingly as the stack grows and shrinks. You are not allowed to use any other data structures for the purposes of manipulating elements, nor may you use the built-in stack tool from Python. Your stack must be implemented as a Linked List, not a Python list. Attributes Type Name Description Node top A pointer to the top of the stack Methods Type Name Description None push(self, item) Adds a new node with value=item to the top of the stack (any) pop(self) Removes the top node and returns its value (any) peek(self) Returns the value of the top node without modifying the stack bool isEmpty(self) Returns True if the stack is empty, False otherwise Special methods Type Name Description str __str__(self) Returns the string representation of this stack str __repr__(self) Returns the same string representation as __str__ int __len__(self) Returns the length of this stack (number of nodes) The Node class has already been implemented for you in the starter code and is described below. Do not modify the Node class. Attributes Type Name Description (any) value The value that this node holds Node next A pointer to the next node in the data structure Type Name Description str __str__(self) Returns the string representation of this node str __repr__(self) Returns the same string representation as __str__3 push(self, item) Adds a new node with value=item to the top of the stack. Nothing is returned by this method. Input (excluding self) (any) item The value to store in a node pop(self) Removes the top node from the stack and returns that node’s value (not the Node object). Output (any) Value held in the topmost node of the stack None Nothing is returned if the stack is empty peek(self) Returns the value (not the Node object) of the topmost node of the stack, but it is not removed. Output (any) Value held in the topmost node of the stack None Nothing is returned if the stack is empty isEmpty(self) Tests to see whether this stack is empty or not. Output bool True if this stack is empty, False otherwise __len__(self) Returns the number of elements in this stack. Output int The number of elements in this stack __str__(self), __repr__(self) Two special methos that return the string representation of the stack. This has already been implemented for you, so do not modify it. If the class is implemented correctly, __str__ and __repr__ display the contents of the stack in the format shown in the doctest. Output str The string representation of this stack
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In python please help - Second and third class will be posted in different questions, thank you. Do not change the function names or given starter code in the script. Each class has different requirements, read them carefully and ask questions if you need clarification. Do not use the exec or eval functions, nor are you allowed to use regular expressions (re module). All methods that output a string must return the string, not print it. If you are unable to complete a method, use the pass statement to avoid syntax errors.
Section 1: The Stack class
This class represents the stack data structure discussed in this module. Use the implementation of
the Node class to implement a stack that supports the following operations.
Make sure to update the top pointer accordingly as the stack grows and shrinks. You are not
allowed to use any other data structures for the purposes of manipulating elements, nor may you
use the built-in stack tool from Python. Your stack must be implemented as a Linked List, not a
Python list.
Attributes
Type Name Description
Node top A pointer to the top of the stack
Methods
Type Name Description
None push(self, item) Adds a new node with value=item to the top of the stack
(any) pop(self) Removes the top node and returns its value
(any) peek(self) Returns the value of the top node without modifying the stack
bool isEmpty(self) Returns True if the stack is empty, False otherwise
Special methods
Type Name Description
str __str__(self) Returns the string representation of this stack
str __repr__(self) Returns the same string representation as __str__
int __len__(self) Returns the length of this stack (number of nodes)
The Node class has already been implemented for you in the starter code and is described below.
Do not modify the Node class.
Attributes
Type Name Description
(any) value The value that this node holds
Node next A pointer to the next node in the data structure
Type Name Description
str __str__(self) Returns the string representation of this node
str __repr__(self) Returns the same string representation as __str__3
push(self, item)
Adds a new node with value=item to the top of the stack. Nothing is returned by this method.
Input (excluding self)
(any) item The value to store in a node
pop(self)
Removes the top node from the stack and returns that node’s value (not the Node object).
Output
(any) Value held in the topmost node of the stack
None Nothing is returned if the stack is empty
peek(self)
Returns the value (not the Node object) of the topmost node of the stack, but it is not removed.
Output
(any) Value held in the topmost node of the stack
None Nothing is returned if the stack is empty
isEmpty(self)
Tests to see whether this stack is empty or not.
Output
bool True if this stack is empty, False otherwise
__len__(self)
Returns the number of elements in this stack.
Output
int The number of elements in this stack
__str__(self), __repr__(self)
Two special methos that return the string representation of the stack. This has already been
implemented for you, so do not modify it. If the class is implemented correctly, __str__ and
__repr__ display the contents of the stack in the format shown in the doctest.
Output
str The string representation of this stack
This class represents the stack data structure discussed in this module. Use the implementation of
the Node class to implement a stack that supports the following operations.
Make sure to update the top pointer accordingly as the stack grows and shrinks. You are not
allowed to use any other data structures for the purposes of manipulating elements, nor may you
use the built-in stack tool from Python. Your stack must be implemented as a Linked List, not a
Python list.
Attributes
Type Name Description
Node top A pointer to the top of the stack
Methods
Type Name Description
None push(self, item) Adds a new node with value=item to the top of the stack
(any) pop(self) Removes the top node and returns its value
(any) peek(self) Returns the value of the top node without modifying the stack
bool isEmpty(self) Returns True if the stack is empty, False otherwise
Special methods
Type Name Description
str __str__(self) Returns the string representation of this stack
str __repr__(self) Returns the same string representation as __str__
int __len__(self) Returns the length of this stack (number of nodes)
The Node class has already been implemented for you in the starter code and is described below.
Do not modify the Node class.
Attributes
Type Name Description
(any) value The value that this node holds
Node next A pointer to the next node in the data structure
Type Name Description
str __str__(self) Returns the string representation of this node
str __repr__(self) Returns the same string representation as __str__3
push(self, item)
Adds a new node with value=item to the top of the stack. Nothing is returned by this method.
Input (excluding self)
(any) item The value to store in a node
pop(self)
Removes the top node from the stack and returns that node’s value (not the Node object).
Output
(any) Value held in the topmost node of the stack
None Nothing is returned if the stack is empty
peek(self)
Returns the value (not the Node object) of the topmost node of the stack, but it is not removed.
Output
(any) Value held in the topmost node of the stack
None Nothing is returned if the stack is empty
isEmpty(self)
Tests to see whether this stack is empty or not.
Output
bool True if this stack is empty, False otherwise
__len__(self)
Returns the number of elements in this stack.
Output
int The number of elements in this stack
__str__(self), __repr__(self)
Two special methos that return the string representation of the stack. This has already been
implemented for you, so do not modify it. If the class is implemented correctly, __str__ and
__repr__ display the contents of the stack in the format shown in the doctest.
Output
str The string representation of this stack
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Recommended textbooks for you
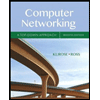
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
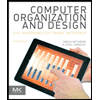
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
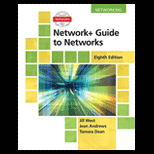
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
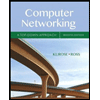
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
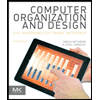
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
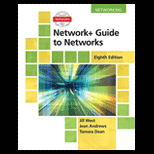
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
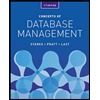
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
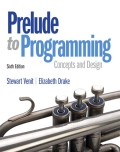
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
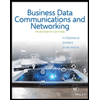
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY