code required for python: For this question, you will be required to use the binary search to find the root of some function f(x)f(x) on the domain x∈[a,b]x∈[a,b] by continuously bisecting the domain. In our case, the root of the function can be defined as the x-values where the function will return 0, i.e. f(x)=0f(x)=0 For example, for the function: f(x)=sin2(x)x2−2f(x)=sin2(x)x2−2 on the domain [0,2][0,2], the root can be found at x≈1.43x≈1.43 Constraints Stopping criteria: ∣∣f(root)∣∣<0.0001|f(root)|<0.0001 or you reach a maximum of 1000 iterations. Round your answer to two decimal places. Function specifications Argument(s): f (function) →→ mathematical expression in the form of a lambda function. domain (tuple) →→ the domain of the function given a set of two integers. MAX (int) →→ the maximum number of iterations that will be performed by the function. Return: root (float) →→ return the root (rounded to two decimals) of the given function. my code below , however as per attached image some tests do not return the correct result. ### START FUNCTION def binary_search(f,domain, MAX = 1000): start ,end = domain while start < end and MAX: mid = (start + end)/ 2 if abs(f(mid)) < 0.0001: return round(mid,2) if f(mid) < 0: start = mid else: end = mid MAX -= 1 return round(mid,2) ### END FUNCTION f = lambda x:(np.sin(x)**2)*(x**2)-2 domain = (0,2) x=binary_search(f,domain) print (binary_search(lambda x:(np.sin(x)**2)*(x**2)-2,(0,2))==1.43) Expected output binary_search(lambda x:(np.sin(x)**2)*(x**2)-2,(0,2))==1.43
code required for python:
For this question, you will be required to use the binary search to find the root of some function f(x)f(x) on the domain x∈[a,b]x∈[a,b] by continuously bisecting the domain. In our case, the root of the function can be defined as the x-values where the function will return 0, i.e. f(x)=0f(x)=0
For example, for the function: f(x)=sin2(x)x2−2f(x)=sin2(x)x2−2 on the domain [0,2][0,2], the root can be found at x≈1.43x≈1.43
Constraints
- Stopping criteria: ∣∣f(root)∣∣<0.0001|f(root)|<0.0001 or you reach a maximum of 1000 iterations.
- Round your answer to two decimal places.
Function specifications
Argument(s):
- f (function) →→ mathematical expression in the form of a lambda function.
- domain (tuple) →→ the domain of the function given a set of two integers.
- MAX (int) →→ the maximum number of iterations that will be performed by the function.
Return:
- root (float) →→ return the root (rounded to two decimals) of the given function.
my code below , however as per attached image some tests do not return the correct result.
### START FUNCTION
def binary_search(f,domain, MAX = 1000):
start ,end = domain
while start < end and MAX:
mid = (start + end)/ 2
if abs(f(mid)) < 0.0001:
return round(mid,2)
if f(mid) < 0:
start = mid
else:
end = mid
MAX -= 1
return round(mid,2)
### END FUNCTION
f = lambda x:(np.sin(x)**2)*(x**2)-2
domain = (0,2)
x=binary_search(f,domain)
print (binary_search(lambda x:(np.sin(x)**2)*(x**2)-2,(0,2))==1.43)
Expected output
binary_search(lambda x:(np.sin(x)**2)*(x**2)-2,(0,2))==1.43
![binary_search
binary_search
Inputs: [<function <lambda> at ex7ff83dfdf6a8>, (2, 3)]
PASSED
FAILED
assert 2.0 == 2.56
your output:
expected output: 2.56
binary_search
Inputs: [<function <lambda> at 0x7ff83deea158>, (-2,
2.0
FAILED
-1)]
assert -2.0 == -1.43
your output:
expected output: -1.43
binary_search
binary_search
Inputs: [<function <lambda> at ex7ff83deea268>, (-2,
-2.0
PASSED
FAILED
-1)]
assert -1.0 == -1.68
your output:
expected output: -1.68
-1.0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9a80abd1-07f7-4db7-81d4-5dae04875d32%2F1fa91736-842b-44a9-a2fb-bf527066ab2e%2F57gqofc_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

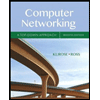
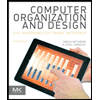
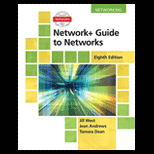
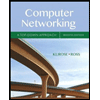
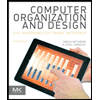
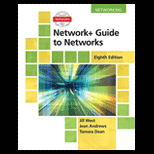
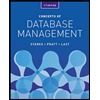
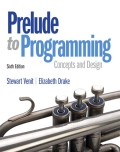
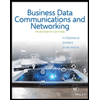