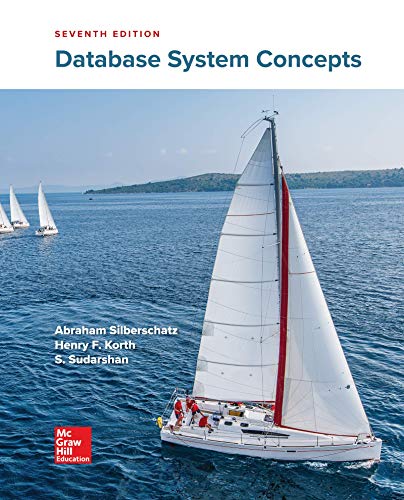
Concept explainers
In C++
Using the code provided below
Do the Following:
- Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools
- Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array)
USE THE FOLLOWING CODE and MODIFY IT:
#define _SECURE_SCL_DEPRECATE 0
#include <iostream>
#include <string>
#include <cstdlib>
using namespace std;
class GChar
{
public:
static const int DEFAULT_CAPACITY = 5;
//constructor
GChar(string name = "john", int capacity = DEFAULT_CAPACITY);
//copy constructor
GChar(const GChar& source);
//Overload Assignment
GChar& operator=(const GChar& source);
//Destructor
~GChar();
//Insert a New Tool
void insert(const std::string& toolName);
private:
//data members
string name;
int capacity;
int used;
string* toolHolder;
};
//constructor
GChar::GChar(string n, int cap)
{
name = n;
capacity = cap;
used = 0;
toolHolder = new string[cap];
}
//copy constructor
GChar::GChar(const GChar& source)
{
cout << "Copy Constructor Called." << endl;
name = source.name;
capacity = source.capacity;
used = source.used;
toolHolder = new string[source.capacity];
copy(source.toolHolder, source.toolHolder + used, toolHolder);
}
//Overload Assignment
GChar& GChar::operator=(const GChar& source)
{
cout << "Overload Assignment Called." << endl;
//Check for self assignment
//c1=c1
if (this == &source)
{
return *this;
}
this->name = source.name;
this->capacity = source.capacity;
used = source.used;
copy(source.toolHolder, source.toolHolder + used, this->toolHolder);
}
//Destructor
GChar::~GChar()
{
cout << "Destructor called for " << this->name << " @ this memory location " << this << endl;
delete[] toolHolder;
}
void GChar::insert(const string& toolName)
{
if (used == capacity)
{
cout << " The tool holder is full, Cannot add any additional tools" << endl;
}
else
{
toolHolder[used] = toolName;
used++;
}
}
int main()
{
GChar gc1;
gc1.insert("potion");
gc1.insert("crossbow");
gc1.insert("candle");
gc1.insert("cloak");
gc1.insert("sword");
gc1.insert("book of spells");
GChar gc2("Bob", 5);
GChar gc3 = gc2;
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- >> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forwardC++ programming task. main.cc file: #include <iostream>#include <map>#include <vector> #include "plane.h" int main() { std::vector<double> weights{3.2, 4.7, 2.1, 5.5, 9.8, 7.4, 1.6, 9.3}; std::cout << "Printing out all the weights: " << std::endl; // ======= YOUR CODE HERE ======== // 1. Using an iterator, print out all the elements in // the weights vector on one line, separated by spaces. // Hint: see the README for the for loop syntax using // an iterator, .begin(), and .end() // ========================== std::cout << std::endl; std::map<std::string, std::string> abbrevs{{"AL", "Alabama"}, {"CA", "California"}, {"GA", "Georgia"}, {"TX", "Texas"}}; std::map<std::string, double> populations{ {"CA", 39.2}, {"GA", 10.8}, {"AL", 5.1}, {"TX", 29.5}}; std::cout <<…arrow_forwardC++ This program does not compile! Spot the error and give the line number. Presume that the header file is error free and // Implementation file for the Rectangle class.1. #include "Rectangle.h" // Needed for the Rectangle class2. #include <iostream> // Needed for cout3. #include <cstdlib> // Needed for the exit function4. using namespace std; //***********************************************************// setWidth sets the value of the member variable width. *//*********************************************************** 5. void Rectangle::setWidth(double w){6. if (w >= 0)7. width = w;8. else{9. cout << "Invalid width\n";10. exit(EXIT_FAILURE);}} //***********************************************************// setLength sets the value of the member variable length. *//*********************************************************** 11. void Rectangle::setLength(double len){12. if (len >= 0)13. length = len;14. else{15. cout << "Invalid length\n";16.…arrow_forward
- Using C++ Programming language: Assume you define a vector in the following way: vector<int> vec; Assign the value 10 to the first element of this vector. What is the statement you would use?arrow_forwardC PROGRAMMING HELP I need help with an old script of mine. I'm having trouble getting math involving accessing an array to work properly, I'm getting an error message that's puzzling me. I'm pretty sure the solution is simple and right in front of me but it's stumping me. Below is the script: #include <stdio.h>#include <math.h>void value(float x){ printf("what is the value of x?"); scanf("%f",&x); }void power(float i,int p,int x,float arr[100]){ float equation,butt; printf("what is the highest power in the series?"); scanf("%d",&p); for (i = 0; i < p; ++i) equation = pow(x,i); //how the hell do i get the counter to do what i want butt = 1+equation^arr[i];}void shortcut(int x){ shortcut = 1/(1-x)}int main(){ printf("Here is the result!:") printf("%d %f", p, f); printf("If we had used shortcut, value would be: %d", shortcut); int diff; diff = shortcut - butt; printf("The difference is only %0.2f!",diff);…arrow_forwardC++ Program: #include <iostream>#include <string> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","LAX"}; int main(){ // define stack (or queue ) here string origin; string dest; string citypair; cout << "Loading the CONTAINER ..." << endl; // LOAD THE STACK ( or queue) HERE // Create all the possible Airport combinations that could exist from the list provided. // i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ... // DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached program (AirportCombos.cpp), create a list of strings to process and place on a STL STACK container. The provided code is meant to be generic. Using the provided 3 char airport codes, create a 6 character string that is the origin &…arrow_forward
- C++ Student Data Write a program that uses two structures Name and Student to store the following information for multiple students: Create a nameType structure that consists of First Name, Middle Initial, and Last Name Create a studentType structure that contains student information (Include the nameType structure within the Student information structure): Name ID email GPA Program (an enum type named programType containing programs such as CSCI, DBMS, INFM, SDEV) Suppose that a class has at most 20 students. Use an array of 20 components of type studentType. You will read in the names, id, and email from classroster.txt. The first line of the file will tell you how many students are in the class. To get the the GPA and program for each student you will need to read from studentlist.txt. The second file will have the id, program, and GPA for all of the students in the department. That means that not every student from studentlist.txt will be in classroster.txt. You will…arrow_forwardC++arrow_forwardC++ Visual Studio 2019 Complete #7 Customer Accounts and #8 Search Function for Customer Accounts Program. Create the array with only 2 elements. 7.Customer Accounts Write a program that uses a structure to store the following data about a customer account: Name Address City, State, and ZIP Telephone Number Account Balance Date of Last Payment The program should use an array of at least 10 structures. It should let the user enter data into the array, change the contents of any element, and display all the data stored in the array. The program should have a menu-driven user interface. Input Validation: When the data for a new account is entered, be sure the user enters data for all the fields. No negative account balances should be entered. 8. Search Function for Customer Accounts Program Add a function to Programming Challenge 7 (Customer Accounts) that allows the user to search the structure array for a particular customer's account. It should accept part of the customer's name as an…arrow_forward
- use c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forwardFind errors / syntax error. Write line numberarrow_forwardDeclare a vector of integers in C++ named vec and initialize the vector to 10, 20, 30, 40, and 50. Modify the first element of the vector to be 100 and modify the last element to be 1000. The resulting vector should then be 100, 20, 30, 40, and 1000. Using cout statements, display the resulting vector to the terminal.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
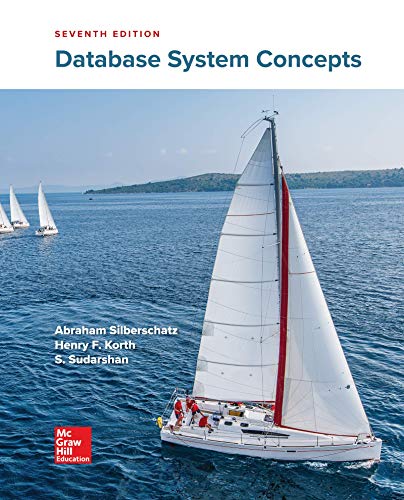
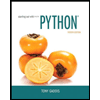
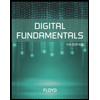
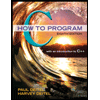
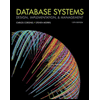
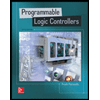