Complete the implementation of an autocorrect function. Given a search query string, the function should return all words which are anagrams. Given 2 arrays, words[n], and queries[q], for each query, return an array of the strings that are anagrams, sorted alphabetically ascending. Note: An anagram is any string that can be formed by rearranging the letters of a string. Example n=4, q=2 words = ["duel", "speed", "dule", "cars"], queries = ["spede","deul"). The only anagram of "spede" is "speed". Both "duel" and "dule" are anagrams of "deul". Return [["speed"], ["duel", "dule"]]. Function Description Complete the function getSearchResults in the editor below. getSearch Results takes the following arguments: string words[n]: the list of words to search string queries[q]: the words to search for Returns string[q][]: the results for each search query Constraints 1 ≤ n, q ≤ 5000 • 1 s length of words[i], length of queries[i] ≤ 100 • It is guaranteed that each query word has at least one anagram in the words list.
Complete the implementation of an autocorrect function. Given a search query string, the function should return all words which are anagrams. Given 2 arrays, words[n], and queries[q], for each query, return an array of the strings that are anagrams, sorted alphabetically ascending. Note: An anagram is any string that can be formed by rearranging the letters of a string. Example n=4, q=2 words = ["duel", "speed", "dule", "cars"], queries = ["spede","deul"). The only anagram of "spede" is "speed". Both "duel" and "dule" are anagrams of "deul". Return [["speed"], ["duel", "dule"]]. Function Description Complete the function getSearchResults in the editor below. getSearch Results takes the following arguments: string words[n]: the list of words to search string queries[q]: the words to search for Returns string[q][]: the results for each search query Constraints 1 ≤ n, q ≤ 5000 • 1 s length of words[i], length of queries[i] ≤ 100 • It is guaranteed that each query word has at least one anagram in the words list.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
![Complete the implementation of an autocorrect function. Given a search query string, the function
should return all words which are anagrams.
Given 2 arrays, words[n], and queries[q], for each query, return an array of the strings that are
anagrams, sorted alphabetically ascending.
Note: An anagram is any string that can be formed by rearranging the letters of a string.
Example
n = 4, q=2
words = ["duel", "speed", "dule", "cars"],
queries=["spede", "deul"].
The only anagram of "spede" is "speed".
Both "duel" and "dule" are anagrams of "deul".
Return [["speed"], ["duel", "dule"]].
Function Description
Complete the function getSearchResults in the editor below.
getSearchResults takes the following arguments:
string words[n]: the list of words to search
string queries[q]: the words to search for
Returns
string[q]: the results for each search query
Constraints
• 1 ≤n, q≤ 5000
• 1 s length of words[i], length of queries[i] ≤ 100
• It is guaranteed that each query word has at least one anagram in the words list.
▼ Input Format For Custom Testing
The first line contains an integer, n, the number of elements in words.
Each of the next n lines contains a string, words[i].
The next line contains an integer, q, the number of elements in queries.
Each of the next n lines contains a string, queries[i].
▾ Sample Case 0
Sample Input For Custom Testing
STDIN
6
allot →
cat
peach
dusty
act
cheap
3
tac
study
peahc
Sample Output
act cat
dusty
cheap peach
Explanation
STDIN
5
emits
items
baker
times
break
2
FUNCTION
The anagrams of "tac" in alphabetical order are "act" and "cat". The only anagram of "study" is
"dusty". The anagrams of "peahc" are "cheap" and "peach".
mites
brake
‒‒‒‒‒‒‒‒
▾ Sample Case 1
Sample Input For Custom Testing
FUNCTION
words [] size n = 6
words = ["allot", "cat", "peach", "dusty", "act", "cheap"]
→
queries] size q 3
queries= ["tac", "study", "peahc"]
→
words [] size n = 5
→ words = ["emits", "items", "baker", "times", "break"]
queries] size q = 2
queries = ["mites", "brake"]
Sample Output
emits items times.
baker break
Explanation
The anagrams of "mites" are "emits", "items", and "times". The anagrams of "brake" are "baker" and
"break".](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F008f31aa-ffde-472d-81b9-49fb75f4a0c2%2F35a93d21-8481-4b8b-993f-6fd5eecbb507%2Fa37qogs_processed.png&w=3840&q=75)
Transcribed Image Text:Complete the implementation of an autocorrect function. Given a search query string, the function
should return all words which are anagrams.
Given 2 arrays, words[n], and queries[q], for each query, return an array of the strings that are
anagrams, sorted alphabetically ascending.
Note: An anagram is any string that can be formed by rearranging the letters of a string.
Example
n = 4, q=2
words = ["duel", "speed", "dule", "cars"],
queries=["spede", "deul"].
The only anagram of "spede" is "speed".
Both "duel" and "dule" are anagrams of "deul".
Return [["speed"], ["duel", "dule"]].
Function Description
Complete the function getSearchResults in the editor below.
getSearchResults takes the following arguments:
string words[n]: the list of words to search
string queries[q]: the words to search for
Returns
string[q]: the results for each search query
Constraints
• 1 ≤n, q≤ 5000
• 1 s length of words[i], length of queries[i] ≤ 100
• It is guaranteed that each query word has at least one anagram in the words list.
▼ Input Format For Custom Testing
The first line contains an integer, n, the number of elements in words.
Each of the next n lines contains a string, words[i].
The next line contains an integer, q, the number of elements in queries.
Each of the next n lines contains a string, queries[i].
▾ Sample Case 0
Sample Input For Custom Testing
STDIN
6
allot →
cat
peach
dusty
act
cheap
3
tac
study
peahc
Sample Output
act cat
dusty
cheap peach
Explanation
STDIN
5
emits
items
baker
times
break
2
FUNCTION
The anagrams of "tac" in alphabetical order are "act" and "cat". The only anagram of "study" is
"dusty". The anagrams of "peahc" are "cheap" and "peach".
mites
brake
‒‒‒‒‒‒‒‒
▾ Sample Case 1
Sample Input For Custom Testing
FUNCTION
words [] size n = 6
words = ["allot", "cat", "peach", "dusty", "act", "cheap"]
→
queries] size q 3
queries= ["tac", "study", "peahc"]
→
words [] size n = 5
→ words = ["emits", "items", "baker", "times", "break"]
queries] size q = 2
queries = ["mites", "brake"]
Sample Output
emits items times.
baker break
Explanation
The anagrams of "mites" are "emits", "items", and "times". The anagrams of "brake" are "baker" and
"break".
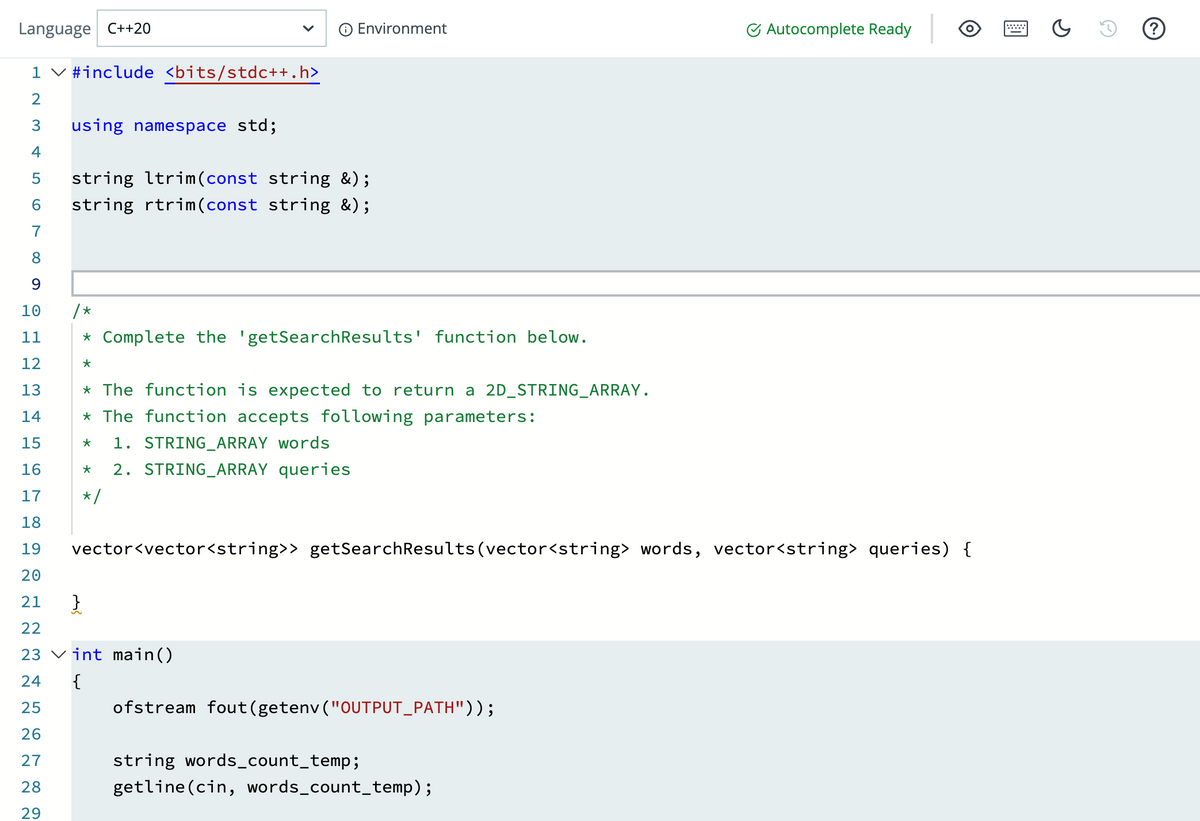
Transcribed Image Text:Language C++20
1 ✓ #include <bits/stdc++.h>
2
3 using namespace std;
4
string ltrim(const string &);
string rtrim(const string &);
✪ Environment
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19 vector<vector<string>> getSearchResults(vector<string> words, vector<string> queries) {
20
21
22
23
24
25
26
27
28
29
/*
* Complete the 'getSearch Results' function below.
*
}
* The function is expected to return a 2D_STRING_ARRAY.
* The function accepts following parameters:
★ 1. STRING ARRAY words
★ 2. STRING_ARRAY queries
*/
int main()
{
ofstream fout (getenv ("OUTPUT_PATH"));
Autocomplete Ready
string words_count_temp;
getline (cin, words_count_temp);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
failed 12 out of 15 tests including time limit tests, if you have to use a different language or change the whole code to attain the 2 second time limit I dont mind, I gave examples of a failed below
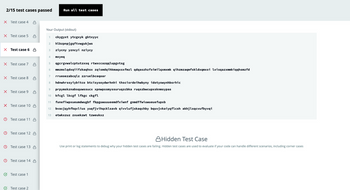
Transcribed Image Text:2/15 test cases passed
X Test case 4 8
X Test case 5 8
X Test case 6 8
X Test case 7
X Test case 8
X Test case 10
X Test case 9 8
8
8
Test case 11 8
Test case 12 A
Test case 13 A
Test case 1
Test case 14 8
Test case 2
Run all test cases
Your Output (stdout)
1 ckygyxt ytcgxyk gktxyyc
2 hlbzpnpjgqftvwgukjws
3 ziycny yzncyi nziycy
4 mxyeq
5
qgcrgvwalcptutzxsq rtwxccazqqlupgvtsg
6 mmzmslqdxqiifzkaqhsx zqismdqihkmaqxsxfmzl qdqaszhzfsimilqxmxmk qihzmzaqmfskldxqmxsi lxisqazxmmkiqqhsmzfd
7 rrueoezabcqlz zzruelbceqoar
8 hdnwhrssyiybitco btcisysoydwrhnhi thsci srdoihwbyny idotyswynhbsrhic
9 prpymskznabsquwssucx xpnwpssmyssuruqzcbka ruqszbwcupxsknmsypas
10 kfcgl lkcgf lfkgc ckgfl
11 funefiwpxueumdwugbf fbpguwuuueemd fxiwnf gnmdffwi uwueuefupxb
12 bvacjqyhfkqvlius yuqfjvihqcklsavb qivvlufjskaqchby bquvjvkaiyqflcsh akhjlsqcvufbyvqi
13 etwkxzuz zxuekzwt tzweukxz
Hidden Test Case
Use print or log statements to debug why your hidden test cases are failing. Hidden test cases are used to evaluate if your code can handle different scenarios, including corner cases
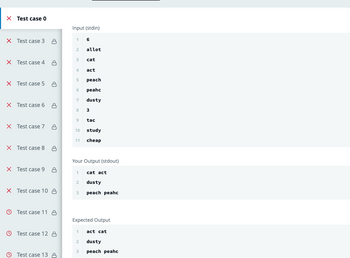
Transcribed Image Text:X Test case 0
X Test case 3
X Test case 4 8
X Test case 5 8
X Test case 6
X
X
(
Test case 7 8
X Test case 9 8
X Test case 10
O
8
Test case 8 8
Test case 11 8
Test case 12
Test case 13 A
Input (stdin)
- NM in 6000 a
16
2
3
4
5
7
allot
cat
act
peach
peahc
dusty
8 3
9 tac
10 study
11 cheap
Your Output (stdout)
1
2 dusty
3 peach peahc
cat act
Expected Output
1 act cat
2 dusty
3 peach peahc
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
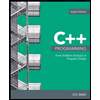
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
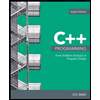
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning