Complete the Kennel class by implementing the following methods: addDog(Dog dog) findYoungestDog() method, which returns the Dog object with the lowest age in the kennel. Assume that no two dogs have the same age. Given classes: Class LabProgram contains the main method for testing the program. Class Kennel represents a kennel, which contains an array of Dog objects as a dog list. (Type your code in here.) Class Dog represents a dog, which has three fields: name, breed, and age. (Hint: getAge() returns a dog's age.) For testing purposes, different dog values will be used. Ex. For the following dogs: Rex Labrador 3.5 Fido Healer 2.0 Snoopy Beagle 3.2 Benji Spaniel 3.9 the output is: Youngest Dog: Fido (Healer) (Age: 2.0)
Complete the Kennel class by implementing the following methods: addDog(Dog dog) findYoungestDog() method, which returns the Dog object with the lowest age in the kennel. Assume that no two dogs have the same age. Given classes: Class LabProgram contains the main method for testing the program. Class Kennel represents a kennel, which contains an array of Dog objects as a dog list. (Type your code in here.) Class Dog represents a dog, which has three fields: name, breed, and age. (Hint: getAge() returns a dog's age.) For testing purposes, different dog values will be used. Ex. For the following dogs: Rex Labrador 3.5 Fido Healer 2.0 Snoopy Beagle 3.2 Benji Spaniel 3.9 the output is: Youngest Dog: Fido (Healer) (Age: 2.0)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Complete the Kennel class by implementing the following methods:
-
addDog(Dog dog)
-
findYoungestDog() method, which returns the Dog object with the lowest age in the kennel.
Assume that no two dogs have the same age.
Given classes:
- Class LabProgram contains the main method for testing the program.
- Class Kennel represents a kennel, which contains an array of Dog objects as a dog list. (Type your code in here.)
- Class Dog represents a dog, which has three fields: name, breed, and age. (Hint: getAge() returns a dog's age.)
For testing purposes, different dog values will be used.
Ex. For the following dogs:
Rex Labrador 3.5
Fido Healer 2.0
Snoopy Beagle 3.2
Benji Spaniel 3.9
the output is:
Youngest Dog: Fido (Healer) (Age: 2.0)
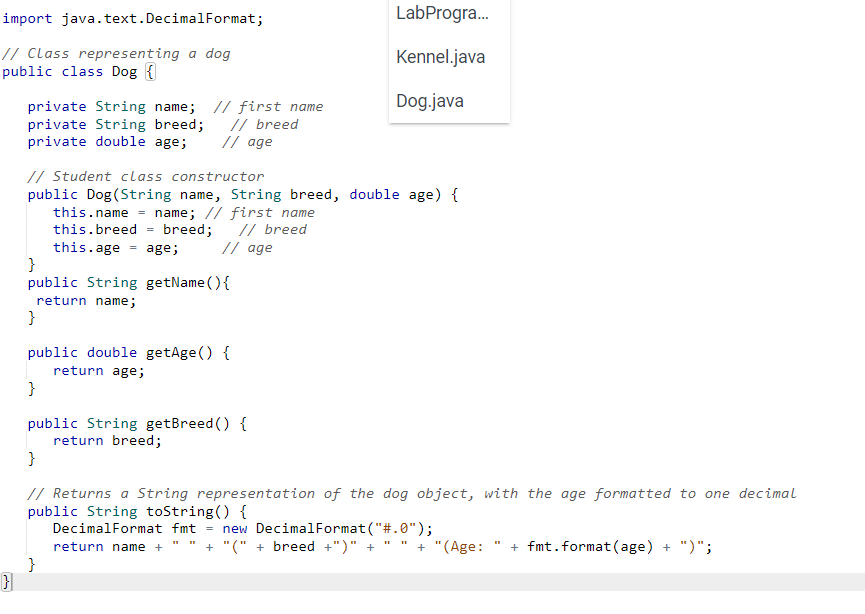
Transcribed Image Text:import java.text.DecimalFormat;
// Class representing a dog
public class Dog {
}
private String name; // first name
private String breed;
private double age;
// breed
=
// age
// Student class constructor
public Dog (String name, String breed, double age) {
this.name = name; // first name
this.breed = breed; // breed
// age
this.age
age;
}
}
public String getName() {
return name;
}
public double getAge() {
return age;
LabProgra...
Kennel.java
Dog.java
public String getBreed() {
return breed;
}
// Returns a String representation of the dog object, with the age formatted to one decimal
public String toString() {
DecimalFormat fmt new DecimalFormat("#.0");
return name +
+ "(" + breed +")" + " " + "(Age: + fmt.format(age) + ")";
"
![import java.util.Scanner;
public class LabProgram {
public static void main(String args[]) {
}
}
Kennel kennel = new Kennel();
int beforeCount;
// Example dogs for testing
kennel.addDog(new Dog("Rex", "Labrador", 3.5));
kennel.addDog(new Dog("Fido", "Healer", 2.0));
kennel.addDog(new Dog("Snoopy", "Beagle", 3.2));
kennel.addDog(new Dog("Benji", "Spaniel", 3.9));
Dog dog
=
kennel.findYoungest Dog();
System.out.println("Youngest
Dog: + dog);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F326c098a-aef3-422a-aa42-2a32e1345aab%2Fe07913fb-114d-4773-9d47-3030825dd3ad%2Frj5geq6_processed.png&w=3840&q=75)
Transcribed Image Text:import java.util.Scanner;
public class LabProgram {
public static void main(String args[]) {
}
}
Kennel kennel = new Kennel();
int beforeCount;
// Example dogs for testing
kennel.addDog(new Dog("Rex", "Labrador", 3.5));
kennel.addDog(new Dog("Fido", "Healer", 2.0));
kennel.addDog(new Dog("Snoopy", "Beagle", 3.2));
kennel.addDog(new Dog("Benji", "Spaniel", 3.9));
Dog dog
=
kennel.findYoungest Dog();
System.out.println("Youngest
Dog: + dog);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
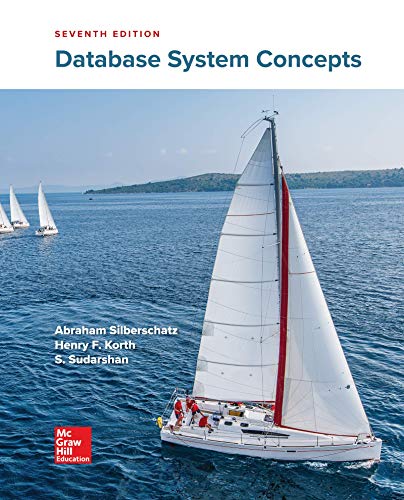
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
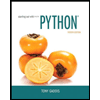
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
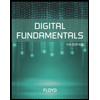
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
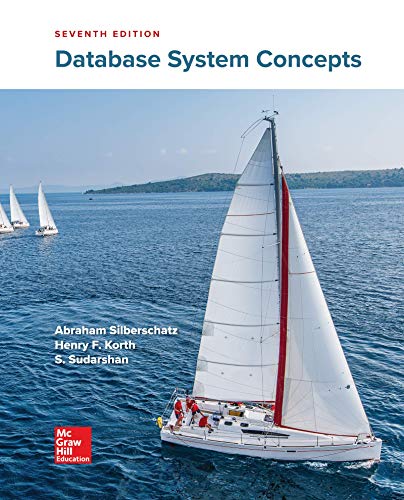
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
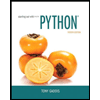
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
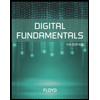
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
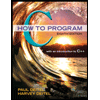
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
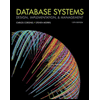
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
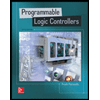
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education