Write a class called Triangle with the required constructor and methods to get the following output. Subtasks: 1. Create a class called Triangle. 2. Create the required constructor. Use Encapsulation to protect the variables. [Hint: Assign the variables in private] 3. Create getBase(), getHeight(), setBase and setHeight() method to access variables. 4. Create a method called area to calculate the area of triangles. 5. Handle the operator overloading by using a special method to calculate the radius and area of triangle 3. [You are not allowed to change the code below] Output: First Triangle Base: 10 First Triangle Height: 5 First Triangle area: 25.0 Second Triangle Base: 5 Second Triangle Height: 3 Second Triangle area: 7.5 Third Triangle Base: 5 Third Triangle Height: 2 Third Triangle area: 5.0 # Write your code here for subtasks 1-5 t1 = Triangle(10, 5) print("First Triangle Base:" , t1.getBase()) print("First Triangle Height:" , t1.getHeight()) print("First Triangle area:" ,t1.area()) t2 = Triangle(5, 3) %3D print("Second Triangle Base:" , t2.getBase()) print("Second Triangle Height:" , t2.getHeight()) print("Second Triangle area:" ,t2.area()) t3 = t1 - t2 print("Third Triangle Base:" , t3.getBase()) print("Third Triangle Height:" , t3.getHeight()) print("Third Triangle area:" ,t3.area())
Write a class called Triangle with the required constructor and methods to get the following output. Subtasks: 1. Create a class called Triangle. 2. Create the required constructor. Use Encapsulation to protect the variables. [Hint: Assign the variables in private] 3. Create getBase(), getHeight(), setBase and setHeight() method to access variables. 4. Create a method called area to calculate the area of triangles. 5. Handle the operator overloading by using a special method to calculate the radius and area of triangle 3. [You are not allowed to change the code below] Output: First Triangle Base: 10 First Triangle Height: 5 First Triangle area: 25.0 Second Triangle Base: 5 Second Triangle Height: 3 Second Triangle area: 7.5 Third Triangle Base: 5 Third Triangle Height: 2 Third Triangle area: 5.0 # Write your code here for subtasks 1-5 t1 = Triangle(10, 5) print("First Triangle Base:" , t1.getBase()) print("First Triangle Height:" , t1.getHeight()) print("First Triangle area:" ,t1.area()) t2 = Triangle(5, 3) %3D print("Second Triangle Base:" , t2.getBase()) print("Second Triangle Height:" , t2.getHeight()) print("Second Triangle area:" ,t2.area()) t3 = t1 - t2 print("Third Triangle Base:" , t3.getBase()) print("Third Triangle Height:" , t3.getHeight()) print("Third Triangle area:" ,t3.area())
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter11: More Object-oriented Programming Concepts
Section: Chapter Questions
Problem 9RQ
Related questions
Question
![Write a class called Triangle with the required constructor and methods to get the
following output.
Subtasks:
1. Create a class called Triangle.
2. Create the required constructor. Use Encapsulation to protect the variables. [Hint:
Assign the variables in private]
3. Create getBase(), getHeight(), setBase and setHeight() method to access
variables.
4. Create a method called area to calculate the area of triangles.
5. Handle the operator overloading by using a special method to calculate the radius
and area of triangle 3.
[You are not allowed to change the code below]
Output:
First Triangle Base: 10
First Triangle Height: 5
First Triangle area: 25.0
Second Triangle Base: 5
Second Triangle Height: 3
Second Triangle area: 7.5
Third Triangle Base: 5
Third Triangle Height: 2
Third Triangle area: 5.0
# Write your code here for subtasks 1-5
t1 = Triangle(10, 5)
print("First Triangle Base:" , t1.getBase())
print("First Triangle Height:" , t1.getHeight())
print("First Triangle area:" ,t1.area())
t2 = Triangle(5, 3)
%3D
print("Second Triangle Base:" , t2.getBase())
print("Second Triangle Height:" , t2.getHeight())
print("Second Triangle area:" ,t2.area())
t3 = t1 - t2
print("Third Triangle Base:" , t3.getBase())
print("Third Triangle Height:" , t3.getHeight())
print("Third Triangle area:" ,t3.area())](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e0c9051-3dee-4a62-bd35-9212a8a517fc%2F7e61860d-8297-4ed1-9be6-5f347c64e120%2Fbtf3yam_processed.png&w=3840&q=75)
Transcribed Image Text:Write a class called Triangle with the required constructor and methods to get the
following output.
Subtasks:
1. Create a class called Triangle.
2. Create the required constructor. Use Encapsulation to protect the variables. [Hint:
Assign the variables in private]
3. Create getBase(), getHeight(), setBase and setHeight() method to access
variables.
4. Create a method called area to calculate the area of triangles.
5. Handle the operator overloading by using a special method to calculate the radius
and area of triangle 3.
[You are not allowed to change the code below]
Output:
First Triangle Base: 10
First Triangle Height: 5
First Triangle area: 25.0
Second Triangle Base: 5
Second Triangle Height: 3
Second Triangle area: 7.5
Third Triangle Base: 5
Third Triangle Height: 2
Third Triangle area: 5.0
# Write your code here for subtasks 1-5
t1 = Triangle(10, 5)
print("First Triangle Base:" , t1.getBase())
print("First Triangle Height:" , t1.getHeight())
print("First Triangle area:" ,t1.area())
t2 = Triangle(5, 3)
%3D
print("Second Triangle Base:" , t2.getBase())
print("Second Triangle Height:" , t2.getHeight())
print("Second Triangle area:" ,t2.area())
t3 = t1 - t2
print("Third Triangle Base:" , t3.getBase())
print("Third Triangle Height:" , t3.getHeight())
print("Third Triangle area:" ,t3.area())
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
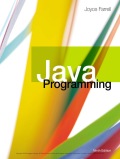
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
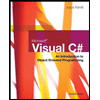
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
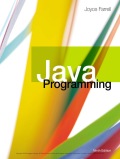
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
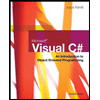
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,