Consider the Bubble Sort algorithm we developed in class. It works, but it may waste some activity. Starting with the code from class, make the following modifications, to make Bubble Sort more efficient. 1. After the first pass, the largest number is guaranteed to be in the highest numbered array index; after the second pass, the two highest numbers are in place; and so on. Modify the code so the sorting algorithm doesn’t bother to make comparisons with these already-in- place elements. For an array of size n, in the first pass n-1 comparisons are required; on the second pass n-2 comparisons are required, etc. 2. What if the array is already sorted? We may be doing all these comparisons, and never changing anything in the array. Modify the sorting algorithm to check at the end of each pass whether any swaps have been made. If no swaps have been made, the array must already be sorted, so you should end the sort. If at least one swap has been made, then at least one more pass through the array is required.
Consider the Bubble Sort algorithm we developed in class. It works, but it may waste some activity. Starting with the code from class, make the following modifications, to make Bubble Sort more efficient. 1. After the first pass, the largest number is guaranteed to be in the highest numbered array index; after the second pass, the two highest numbers are in place; and so on. Modify the code so the sorting algorithm doesn’t bother to make comparisons with these already-in- place elements. For an array of size n, in the first pass n-1 comparisons are required; on the second pass n-2 comparisons are required, etc. 2. What if the array is already sorted? We may be doing all these comparisons, and never changing anything in the array. Modify the sorting algorithm to check at the end of each pass whether any swaps have been made. If no swaps have been made, the array must already be sorted, so you should end the sort. If at least one swap has been made, then at least one more pass through the array is required.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Modify the given code according to what it ask you to do
Show your modified code in a picture
![8 #include <stdio.h>
9
#define SIZE 10
// function main begins program execution
int main(void){
// initialize a
int a[sIZE]
14 puts ( "Data items in original order");
// output original array
for (size_t i = 0; i < SIZE; ++i) {
10
11
12
13
{2, 6, 4, 8, 10, 12, 89, 68, 45, 37};
%3D
15
16
printf( "%4d", ali]);
17
18
}
// bubble sort
// loop to control number of passes
19
20
for (unsigned int pass = 1; pass < SIZE; ++pass) {
// loop to control number of comparisons per pass
for (size_t i = 0; i < SIZE -
// compare adjacent elements and swap them if first
21
22
23
1; ++i) {
24
25
// element is greater than second element
if (a[i] > a[i + 1]) {
int hold = a[i];
ali] = a[i + 1];
ali+ 1] = hold;
26
27
28
29
30
}
}
puts ("\nData items in ascending order");
// output sorted array
for (size_t i = 0; i < SIZE; ++i) {
printf( "%4d", a[i]);
}
31
32
33
34
35
36
37
puts ("");
38
39 }
Data items in original order
2 6 4
8 10
12
89
68
45 37
Data items in ascending order
2 4 6 8 10
12 37
45
68
89
Program ended with exit code: 0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffe556c26-ee28-41c4-9070-fc792c89c4b4%2Fae9895b6-92ac-40b6-bd18-3def94f909cb%2F5k7waf_processed.jpeg&w=3840&q=75)
Transcribed Image Text:8 #include <stdio.h>
9
#define SIZE 10
// function main begins program execution
int main(void){
// initialize a
int a[sIZE]
14 puts ( "Data items in original order");
// output original array
for (size_t i = 0; i < SIZE; ++i) {
10
11
12
13
{2, 6, 4, 8, 10, 12, 89, 68, 45, 37};
%3D
15
16
printf( "%4d", ali]);
17
18
}
// bubble sort
// loop to control number of passes
19
20
for (unsigned int pass = 1; pass < SIZE; ++pass) {
// loop to control number of comparisons per pass
for (size_t i = 0; i < SIZE -
// compare adjacent elements and swap them if first
21
22
23
1; ++i) {
24
25
// element is greater than second element
if (a[i] > a[i + 1]) {
int hold = a[i];
ali] = a[i + 1];
ali+ 1] = hold;
26
27
28
29
30
}
}
puts ("\nData items in ascending order");
// output sorted array
for (size_t i = 0; i < SIZE; ++i) {
printf( "%4d", a[i]);
}
31
32
33
34
35
36
37
puts ("");
38
39 }
Data items in original order
2 6 4
8 10
12
89
68
45 37
Data items in ascending order
2 4 6 8 10
12 37
45
68
89
Program ended with exit code: 0
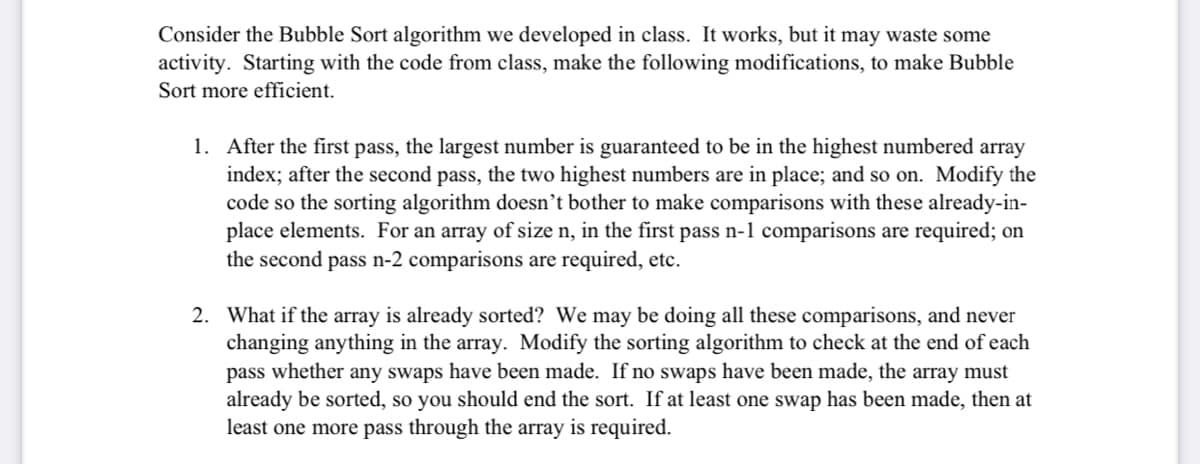
Transcribed Image Text:Consider the Bubble Sort algorithm we developed in class. It works, but it may waste some
activity. Starting with the code from class, make the following modifications, to make Bubble
Sort more efficient.
1. After the first pass, the largest number is guaranteed to be in the highest numbered array
index; after the second pass, the two highest numbers are in place; and so on. Modify the
code so the sorting algorithm doesn't bother to make comparisons with these already-in-
place elements. For an array of size n, in the first pass n-1 comparisons are required; on
the second pass n-2 comparisons are required, etc.
2. What if the array is already sorted? We may be doing all these comparisons, and never
changing anything in the array. Modify the sorting algorithm to check at the end of each
pass whether any swaps have been made. If no swaps have been made, the array must
already be sorted, so you should end the sort. If at least one swap has been made, then at
least one more pass through the array is required.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
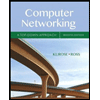
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
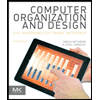
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
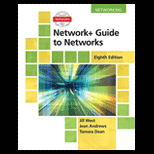
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
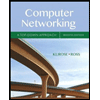
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
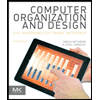
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
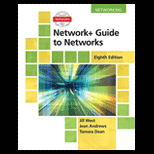
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
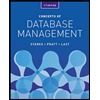
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
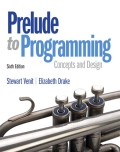
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
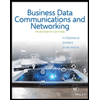
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY