) Convert your structure into a class. For this exercise, you can leave the data as public (otherwise you would have to change the input and output functions). 2) Write a member function show_all that prints all the information for one record - name, cost, markup, and the three inventory numbers. 3) Add a user option S that lets the user see all the information for all the items in the inventory, using the show_all member function. Print a header so the user knows what each column means, and format the output to appear in columns. Hint: do a setwidth() before *each* cout. Pick widths that make sense for name, cost, markup, and the three inventory numbers.
Previous Code:
#include <iostream>
using namespace std;
// function to prompt for the re-assigning
int placement(int REDApplesCounter, int REDApplesCounterOnShelf, int AssortedBouquetsCounter, int CamembertCheeseCounter)
{
// prompt for values
cout<<"Enter the values in (P)lacement \n "<<endl;
cout<<"Items Exists are: Red Delicious Apples, Assorted bouquets, Camembert Cheese"<<endl;
cout<<"Enter the Red Apples Count: "; cin>>REDApplesCounter;
cout<<"Enter the Red Apples on shelf Count: "; cin>>REDApplesCounterOnShelf;
cout<<"Enter the Assorted bouquets Count: "; cin>>AssortedBouquetsCounter;
cout<<"Enter the Camembert Cheese Count: "; cin>>CamembertCheeseCounter;
return (REDApplesCounter, REDApplesCounterOnShelf, AssortedBouquetsCounter, CamembertCheeseCounter);
}
// main function
int main()
{
// prompt for the values
cout<<"Enter values "<<endl;
int REDApplesCounter, REDApplesCounterOnShelf, AssortedBouquetsCounter, CamembertCheeseCounter;
cout<<"Items Exists are: Red Delicious Apples, Assorted bouquets, Camembert Cheese"<<endl;
cout<<"Enter the Red Apples Count: "; cin>>REDApplesCounter;
cout<<"Enter the Red Apples on shelf Count: "; cin>>REDApplesCounterOnShelf;
cout<<"Enter the Assorted bouquets Count: "; cin>>AssortedBouquetsCounter;
cout<<"Enter the Camembert Cheese Count: "; cin>>CamembertCheeseCounter;
cout<<"\n***********************************"<<endl;
// prompt for choice
char choice;
cout<<"You have 3 \n(P)lacement\t(W)arning\te(X)it: ";cin>>choice;
// defining the switch cases
switch(choice)
{
// for Placement
case 'P':
{
cout<<endl;
cout<<"\nThe Red Apples Counts are: "<<REDApplesCounter;
cout<<"\nThe Red Apples on shelf Counts are: "<<REDApplesCounterOnShelf;
cout<<"\nThe Assorted bouquets Counts are: "<<AssortedBouquetsCounter;
cout<<"\nThe Camembert Cheese Counts are: "<<CamembertCheeseCounter;
cout<<"\nIf you want replacement then enter 'Y' otherwise 'N': ";
bool ch;
cin>>ch;
// if user wants to again assign the values
if(ch=='Y')
{
placement(REDApplesCounter, REDApplesCounterOnShelf, AssortedBouquetsCounter, CamembertCheeseCounter);
}
break;
}
// For warnings
case 'W':
{
cout<<endl;
if(REDApplesCounter<10)
cout<<"Warning: Red delicious Apples counter: "<<REDApplesCounter<<endl;
if(REDApplesCounterOnShelf<10)
cout<<"Warning: Red delicious Apples shelf: "<<REDApplesCounterOnShelf<<endl;
if(AssortedBouquetsCounter<10)
cout<<"Warning: Assorted bouquets warehouse: "<< AssortedBouquetsCounter<<endl;
if(CamembertCheeseCounter<10)
cout<<"Warning: Camembert Cheese warehouse: "<< CamembertCheeseCounter<<endl;
break;
}
// Exit from the program
case 'X':
exit(0);
default:
cout<<"Wrong Input: "<<endl;
}
return 0;

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

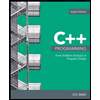
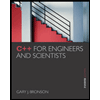
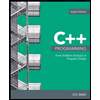
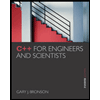