Create a C++ program that produces a two-dimensional board of bombs & numbers that could be used as a data structure inside a minesweeper game such as the one that comes with Microsoft Windows. Display of the board will be text-based & appear in the format shown in the example-program-output.txt file with each digit or character printed in a field width of three. A blank square shall appear as an underscore character, a bomb square shall appear as a '#' character, & a number square shall appear as the number. The game board data structure shall consist of a two-dimensional integer array. A blank square shall be stored as a zero, a bomb shall be stored as a -1, & a number (i.e., clue) square shall be stored as the respective number. Program shall follow this basic algorithm: Create a game board as a two-dimensional integer array with MAX_ROWS and MAX_COLUMNS Randomly fill squares on the game board with bombs or zeros Go through board, square by square, looking for each bomb. When one is found, increment (by one) the values in the number squares surrounding a bomb Display game board on screen Program shall have no interaction with users except for starting the program. Implement the design described below: int main(void) void fillTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS]) Set up a double for loop that will iterate through each row and column of the board in order to fill the squares. Be sure to use the MAX_ROWS and MAX_COLUMNS constants in the 'for loop' source code instead of literal numbers given for the those constants in the skeleton file. Inside the innermost 'for' loop, store a BOMB_DIGIT in a square when the following probability formula is true: (rand() % (MAX_ROWS - 3) == 0), otherwise, put an EMPTY_SQUARE_DIGIT in the square. Use BOMB_DIGIT and EMPTY_SQUARE_DIGIT constants in your source code. void displayTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS]) Set up a double for loop that will iterate through each row & column of the board in order to print (in width of 3) the contents of each square as shown in example-program-output.txt file. Inside inner for loop, use an if-else structure to do the following. If a square contains the BOMB_DIGIT, then print the BOMB_SYMBOL. If, instead, a square contains EMPTY_SQUARE_DIGIT then, print EMPTY_SQUARE_SYMBOL; otherwise, print numeric value stored in the square. void insertTheMineClues(int board[MAX_ROWS][MAX_COLUMNS]) Set up a double for loop that will iterate through each row and column of board. Do following inside inner for loop. If a square contains the BOMB_DIGIT then call the incrementTheNeighborSquares function and pass it the board, the current row value, & the current column value. void incrementTheNeighborSquares(int board[MAX_ROWS][MAX_COLUMNS], int bombRow, int bombColumn) The algorithm checks all of neighbor (i.e., adjacent) squares surrounding a bomb square. If neighbor square does not contain BOMB_DIGIT, then value in the square is incremented by one. A double for loop is used to iterate through the row & column location of each neighbor square surrounding the bomb square. Because C++ does no range checking when an array is indexed, the algorithm checks that, also. This function definition is already finished. Make no changes to it. Files used: example-program-output Skeleton15.cpp (copied below) #include #include #include using namespace std; const int MAX_ROWS = 10; const int MAX_COLUMNS = 10; const int EMPTY_SQUARE_DIGIT = 0; const char EMPTY_SQUARE_SYMBOL = '_'; const int BOMB_DIGIT = -1; const char BOMB_SYMBOL = '#'; void fillTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS]); void displayTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS]); void insertTheMineClues(int board[MAX_ROWS][MAX_COLUMNS]); void incrementTheNeighborSquares(int board[MAX_ROWS][MAX_COLUMNS], int row, int column); int main(void) { int gameBoard[MAX_ROWS][MAX_COLUMNS]; srand(time(NULL)); fillTheGameBoard(gameBoard); insertTheMineClues(gameBoard); displayTheGameBoard(gameBoard); system("pause"); return 0; } void fillTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS]) { } void displayTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS]) { } void insertTheMineClues(int board[MAX_ROWS][MAX_COLUMNS]) { } // The function definition below is finished. Make no changes to it. void incrementTheNeighborSquares(int board[MAX_ROWS][MAX_COLUMNS], int bombRow, int bombColumn) { for (int row = bombRow - 1; row <= bombRow + 1; row++) { if ( (row >= 0) && (row < MAX_ROWS) ) { for (int column = bombColumn - 1; column <= bombColumn + 1; column++) { if ( (column >= 0) && (column < MAX_COLUMNS) && (board[row][column] != BOMB_DIGIT) ) board[row][column]++; } } } }
Create a C++
Display of the board will be text-based & appear in the format shown in the example-program-output.txt file with each digit or character printed in a field width of three. A blank square shall appear as an underscore character, a bomb square shall appear as a '#' character, & a number square shall appear as the number.
The game board data structure shall consist of a two-dimensional integer array. A blank square shall be stored as a zero, a bomb shall be stored as a -1, & a number (i.e., clue) square shall be stored as the respective number.
Program shall follow this basic
Create a game board as a two-dimensional integer array with MAX_ROWS and MAX_COLUMNS
Randomly fill squares on the game board with bombs or zeros
Go through board, square by square, looking for each bomb. When one is found, increment (by one) the values in the number squares surrounding a bomb
Display game board on screen
Program shall have no interaction with users except for starting the program.
Implement the design described below:
int main(void)
void fillTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS])
Set up a double for loop that will iterate through each row and column of the board in order to fill the squares. Be sure to use the MAX_ROWS and MAX_COLUMNS constants in the 'for loop' source code instead of literal numbers given for the those constants in the skeleton file.
Inside the innermost 'for' loop, store a BOMB_DIGIT in a square when the following probability formula is true: (rand() % (MAX_ROWS - 3) == 0), otherwise, put an EMPTY_SQUARE_DIGIT in the square. Use BOMB_DIGIT and EMPTY_SQUARE_DIGIT constants in your source code.
void displayTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS])
Set up a double for loop that will iterate through each row & column of the board in order to print (in width of 3) the contents of each square as shown in example-program-output.txt file. Inside inner for loop, use an if-else structure to do the following. If a square contains the BOMB_DIGIT, then print the BOMB_SYMBOL. If, instead, a square contains EMPTY_SQUARE_DIGIT then, print EMPTY_SQUARE_SYMBOL; otherwise, print numeric value stored in the square.
void insertTheMineClues(int board[MAX_ROWS][MAX_COLUMNS])
Set up a double for loop that will iterate through each row and column of board. Do following inside inner for loop. If a square contains the BOMB_DIGIT then call the incrementTheNeighborSquares function and pass it the board, the current row value, & the current column value.
void incrementTheNeighborSquares(int board[MAX_ROWS][MAX_COLUMNS], int bombRow, int bombColumn)
The algorithm checks all of neighbor (i.e., adjacent) squares surrounding a bomb square. If neighbor square does not contain BOMB_DIGIT, then value in the square is incremented by one. A double for loop is used to iterate through the row & column location of each neighbor square surrounding the bomb square. Because C++ does no range checking when an array is indexed, the algorithm checks that, also.
This function definition is already finished. Make no changes to it.
Files used:
example-program-output
Skeleton15.cpp (copied below)
#include <iostream>
#include <iomanip>
#include <time.h>
using namespace std;
const int MAX_ROWS = 10;
const int MAX_COLUMNS = 10;
const int EMPTY_SQUARE_DIGIT = 0;
const char EMPTY_SQUARE_SYMBOL = '_';
const int BOMB_DIGIT = -1;
const char BOMB_SYMBOL = '#';
void fillTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS]);
void displayTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS]);
void insertTheMineClues(int board[MAX_ROWS][MAX_COLUMNS]);
void incrementTheNeighborSquares(int board[MAX_ROWS][MAX_COLUMNS], int row, int column);
int main(void)
{
int gameBoard[MAX_ROWS][MAX_COLUMNS];
srand(time(NULL));
fillTheGameBoard(gameBoard);
insertTheMineClues(gameBoard);
displayTheGameBoard(gameBoard);
system("pause");
return 0;
}
void fillTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS])
{
} void displayTheGameBoard(int board[MAX_ROWS][MAX_COLUMNS])
{
}
void insertTheMineClues(int board[MAX_ROWS][MAX_COLUMNS])
{
}
// The function definition below is finished. Make no changes to it.
void incrementTheNeighborSquares(int board[MAX_ROWS][MAX_COLUMNS], int bombRow, int bombColumn)
{
for (int row = bombRow - 1; row <= bombRow + 1; row++)
{
if ( (row >= 0) && (row < MAX_ROWS) )
{
for (int column = bombColumn - 1; column <= bombColumn + 1; column++)
{
if ( (column >= 0) && (column < MAX_COLUMNS) && (board[row][column] != BOMB_DIGIT) )
board[row][column]++;
}
}
}
}
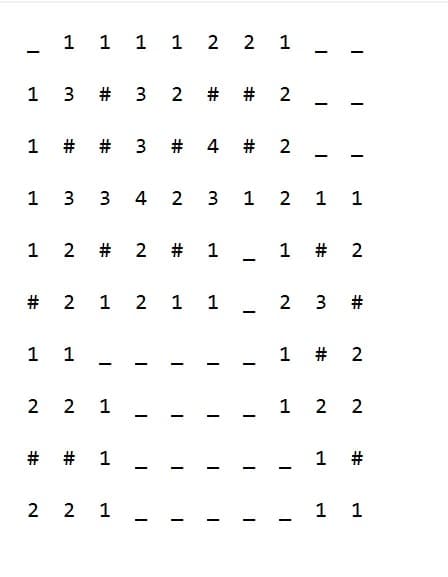

Step by step
Solved in 3 steps with 2 images

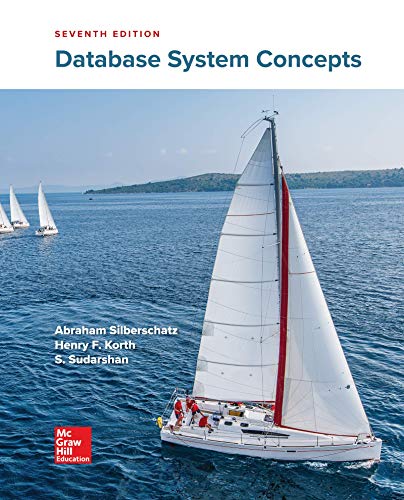
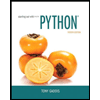
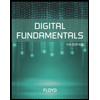
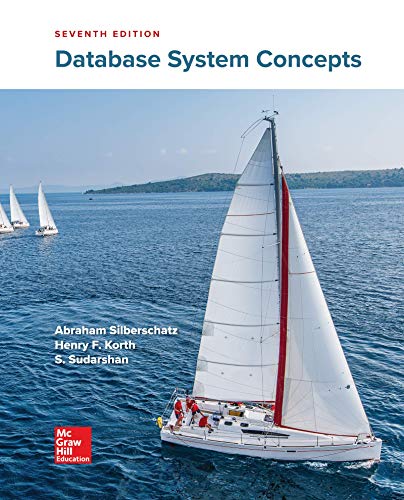
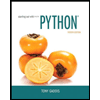
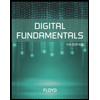
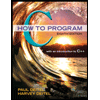
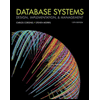
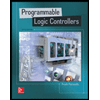