Create a class of records for a gradebook called Rec. The data should be private, and should include a firstname, lastname, array of three grades, and a field for average grade. Build two constructors: a default constructor and a constructor that takes the first and last name. Build a function to read the data, either from a file or from cin. The read function reads the two names and three grades, but does not read the average grade. Build a write function that writes the data either to a file or to cout. The write function prints all of the data on one line with spaces between fields. Build a function to calculate the average grade field. I will provide you with a driver called hw1.cpp in ~cthorpe/public/142 and with a test file HW1.txt in the same directory HW1.txt contains: Pete Jones 1 2 4 This is what I have done so far: #include #include #include using namespace std; class Rec { private: string fname; string lname; int grades[3]; int avg_grade; public: Rec() { fname = "student"; lname = "one"; avg_grade = 0; grades = {0}; } Rec(string first, string last) { fname = first; lname = last; } void read(ifstream &in, string fname, string lname, int grades[], int avg_grade) { int i = 0; while (!in.eof()) { in >> fname >> lname >> grades[i]; i++; } } void write(string fname, string lname, int grades[],int avg_grade) { int i = 0; for (int i = 0; i < 3; i++) { cout << fname << " " << lname << " "; cout << grades[i] << " " << avg_grade << endl; } } }; int main() { Rec r1; Rec r2("Smith", "Jean"); ifstream instr; instr.open("HW1.txt"); cout << "Blank record" << endl; r1.write(cout); cout << "Initialized record" << endl; r2.write(cout); r1.read(instr); if (r1 == r2) cout << "same"; else cout << "different"; cout << endl; r1.calc_avg(); r1.write(cout); cout << endl; return 0; } I need help with the rest and want to know if I did anything wrong so far.
Create a class of records for a gradebook called Rec.
The data should be private, and should include a firstname, lastname, array of three grades, and a field for average grade.
Build two constructors: a default constructor and a constructor that takes the first and last name.
Build a function to read the data, either from a file or from cin. The read function reads the two names and three grades, but does not read the average grade.
Build a write function that writes the data either to a file or to cout. The write function prints all of the data on one line with spaces between fields.
Build a function to calculate the average grade field.
I will provide you with a driver called hw1.cpp in ~cthorpe/public/142 and with a test file HW1.txt in the same directory
HW1.txt contains:
Pete Jones 1 2 4
This is what I have done so far:
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Rec {
private:
string fname;
string lname;
int grades[3];
int avg_grade;
public:
Rec()
{
fname = "student";
lname = "one";
avg_grade = 0;
grades = {0};
}
Rec(string first, string last)
{
fname = first;
lname = last;
}
void read(ifstream &in, string fname, string lname, int grades[], int avg_grade)
{
int i = 0;
while (!in.eof())
{
in >> fname >> lname >> grades[i];
i++;
}
}
void write(string fname, string lname, int grades[],int avg_grade)
{
int i = 0;
for (int i = 0; i < 3; i++)
{
cout << fname << " " << lname << " ";
cout << grades[i] << " " << avg_grade << endl;
}
}
};
int main()
{
Rec r1;
Rec r2("Smith", "Jean");
ifstream instr;
instr.open("HW1.txt");
cout << "Blank record" << endl;
r1.write(cout);
cout << "Initialized record" << endl;
r2.write(cout);
r1.read(instr);
if (r1 == r2) cout << "same"; else cout << "different";
cout << endl;
r1.calc_avg();
r1.write(cout);
cout << endl;
return 0;
}
I need help with the rest and want to know if I did anything wrong so far.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

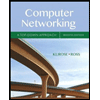
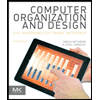
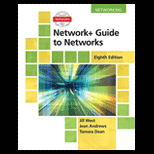
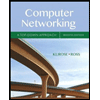
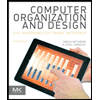
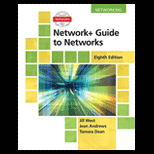
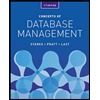
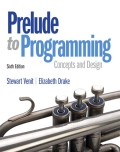
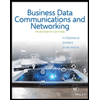