create a file in c++. In this lab, you will replace the existing functions with more flexible ones, and you will add additional functions to perform modular tasks. The "old" functions for displaying "hello", "goodbye", "invalid selection" and "invalid input" all do basically the same thing. They simply display a message to the user. Replace the old functions with a new function called displayMessage. The function should take a string argument, and display it. void displayMessage(string); //prototype The old function for displaying the menu would be more useful if it also returned the menu selection. Replace the old function with a new one called getMenuSelection that will return the menu selection as a char variable. Make sure to use the function result when you call it (assignment, display, use in expression, another function call, etc.). char getMenuSelection(); //prototype Wouldn't it be nice to check the menu selection for an invalid input? How about checking to see if the exit condition was selected? Write (and use) new functions that return boolean values for each check. bool isInvalidSelection(char); //prototype (returns true if argument is an invalid selection) bool isExitSelection(char); //prototype (returns true if argument matches the exit condition(s) X or x) All the math menu selections are also very similar. Ask the user for two numbers, perform an operation, and display the result. If the selection was for division, then input validation was required for the second number. Write a single function to perform any math operation, and write another check function for input validation during division. void doMath(int); //prototype (int is a constant that determines which operation to perform) bool isInvalidInput(int); //prototype (returns true if argument is equal to 0) Using constants would be very helpful... const int ADD = 1; //etc. for other operations const string HELLO = "Hello! Welcome to the program.\n"; //etc. for each message You can use the result of a boolean function in test expressions... while (isInvalidInput(num2)) { } When completed, your calculator program should be very "modular", with very little happening in the main() function! Prototypes should be written for each function Function definitions can be in any order, after main() The program should: do everything that the previous versions have done use constants and functions as indicated above
create a file in c++.
In this lab, you will replace the existing functions with more flexible ones, and you will add additional functions to perform modular tasks.
The "old" functions for displaying "hello", "goodbye", "invalid selection" and "invalid input" all do basically the same thing. They simply display a message to the user. Replace the old functions with a new function called displayMessage. The function should take a string argument, and display it.
void displayMessage(string); //prototype
The old function for displaying the menu would be more useful if it also returned the menu selection. Replace the old function with a new one called getMenuSelection that will return the menu selection as a char variable. Make sure to use the function result when you call it (assignment, display, use in expression, another function call, etc.).
char getMenuSelection(); //prototype
Wouldn't it be nice to check the menu selection for an invalid input? How about checking to see if the exit condition was selected? Write (and use) new functions that return boolean values for each check.
bool isInvalidSelection(char); //prototype (returns true if argument is an invalid selection)
bool isExitSelection(char); //prototype (returns true if argument matches the exit condition(s) X or x)
All the math menu selections are also very similar. Ask the user for two numbers, perform an operation, and display the result. If the selection was for division, then input validation was required for the second number. Write a single function to perform any math operation, and write another check function for input validation during division.
void doMath(int); //prototype (int is a constant that determines which operation to perform)
bool isInvalidInput(int); //prototype (returns true if argument is equal to 0)
Using constants would be very helpful...
const int ADD = 1; //etc. for other operations
const string HELLO = "Hello! Welcome to the
You can use the result of a boolean function in test expressions...
while (isInvalidInput(num2)) { }
When completed, your calculator program should be very "modular", with very little happening in the main() function!
Prototypes should be written for each function
Function definitions can be in any order, after main()
The program should:
- do everything that the previous versions have done
- use constants and functions as indicated above

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

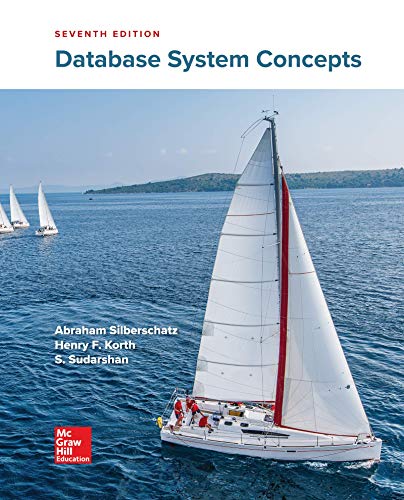
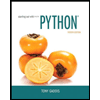
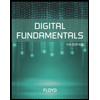
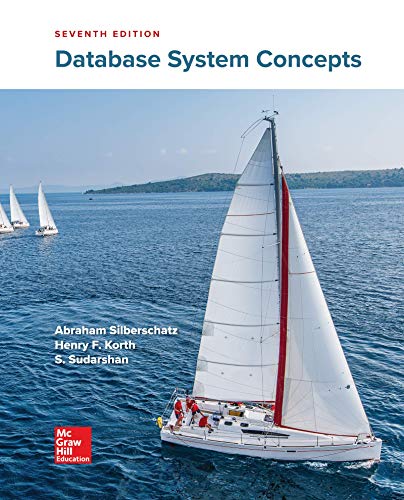
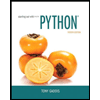
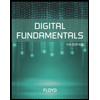
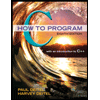
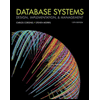
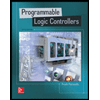