Create a Java program that plays a game of rock, paper, scissors. Your program should allow the user to enter "rock", "paper", or "scissors". The program will randomly select "rock", "paper", or "scissors". The winner is then printed. GameFunctions Class (GameFunctions.java) This class should contain the following: • Two private fields. o generator - A Random object for generating random numbers. • This field must not be static. computerChoice - A String for holding the computer's current choice. This field must be static. One public constructor that accepts no arguments. O The constructor must assign an empty String to computerChoice field and instantiate a Random object for the generator field. Do not give the Random object a seed value. • Two public methods. o A void method named choose that accepts no arguments. • When called, the method randomly picks a number between 1, 2 and 3, with each associated with "rock", "paper", or "scissors". The method then assigns the String value "rock", "paper", or "scissors" to the computerChoice field. Finally, the method prints the computer's choice. o Astatic method named whoWon that accepts one String argument • A String passed as to the method as an argument will be the user's choice. The function should return a String: "user" if the user won "computer" if the computer won "tie" if neither won. Rules for determining the winner: • Rock beats Scissors • Scissors beats Paper • Paper beats Rock If the user and computer made the same choice, it's a tie.
Create a Java program that plays a game of rock, paper, scissors. Your program should allow the user to enter "rock", "paper", or "scissors". The program will randomly select "rock", "paper", or "scissors". The winner is then printed. GameFunctions Class (GameFunctions.java) This class should contain the following: • Two private fields. o generator - A Random object for generating random numbers. • This field must not be static. computerChoice - A String for holding the computer's current choice. This field must be static. One public constructor that accepts no arguments. O The constructor must assign an empty String to computerChoice field and instantiate a Random object for the generator field. Do not give the Random object a seed value. • Two public methods. o A void method named choose that accepts no arguments. • When called, the method randomly picks a number between 1, 2 and 3, with each associated with "rock", "paper", or "scissors". The method then assigns the String value "rock", "paper", or "scissors" to the computerChoice field. Finally, the method prints the computer's choice. o Astatic method named whoWon that accepts one String argument • A String passed as to the method as an argument will be the user's choice. The function should return a String: "user" if the user won "computer" if the computer won "tie" if neither won. Rules for determining the winner: • Rock beats Scissors • Scissors beats Paper • Paper beats Rock If the user and computer made the same choice, it's a tie.
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 9E: Write a program named SalespersonDemo that instantiates objects using classes named Real...
Related questions
Question
100%
Hello there,
Can you help me here please??
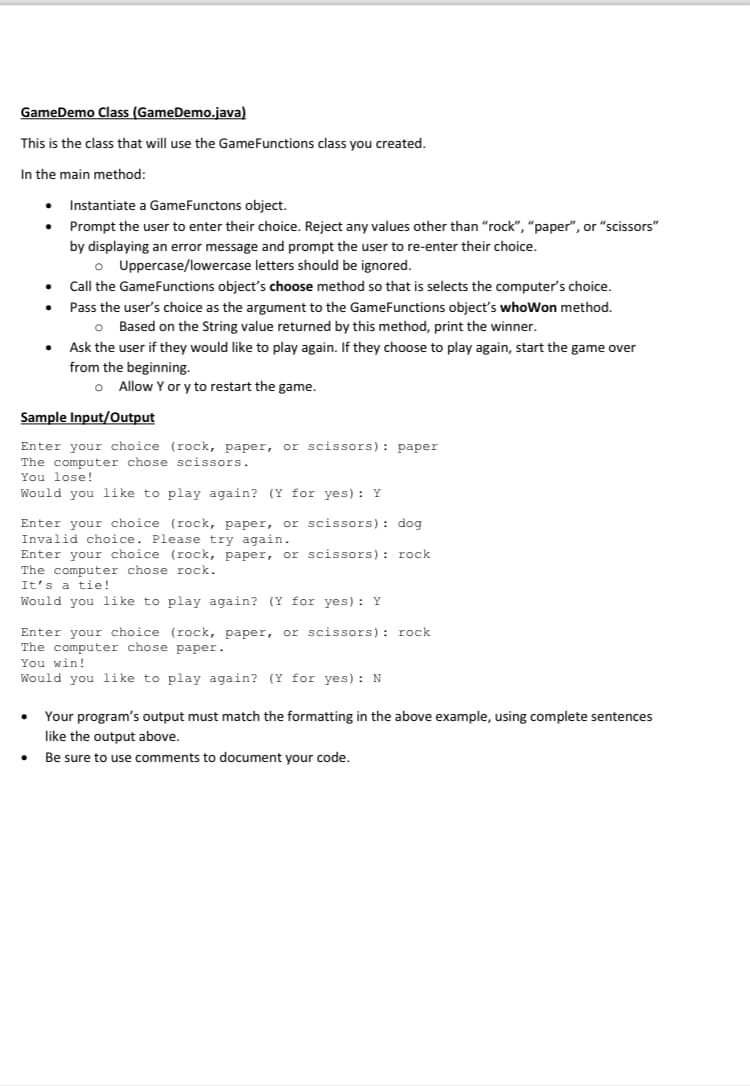
Transcribed Image Text:GameDemo Class (GameDemo.java)
This is the class that will use the GameFunctions class you created.
In the main method:
Instantiate a GameFunctons object.
Prompt the user to enter their choice. Reject any values other than "rock", "paper", or "scissors"
by displaying an error message and prompt the user to re-enter their choice.
Uppercase/lowercase letters should be ignored.
Call the GameFunctions object's choose method so that is selects the computer's choice.
Pass the user's choice as the argument to the GameFunctions object's whoWon method.
Based on the String value returned by this method, print the winner.
Ask the user if they would like to play again. If they choose to play again, start the game over
from the beginning.
Allow
or y to restart the game.
Sample Input/Output
Enter your choice (rock, paper, or scissors): paper
The computer chose scissors.
You lose!
Would you like to play again? (Y for yes) : Y
Enter your choice (rock, paper, or scissors): dog
Invalid choice. Please try aqain.
Enter your choice (rock, paper, or scissors): rock
The computer chose rock.
It's a tie!
Would you like to play again? (Y for yes): Y
Enter your choice (rock, paper, or scissors): rock
The computer chose paper.
You win!
Would you like to play again? (Y for yes) : N
Your program's output must match the formatting in the above example, using complete sentences
like the output above.
Be sure to use comments to document your code.
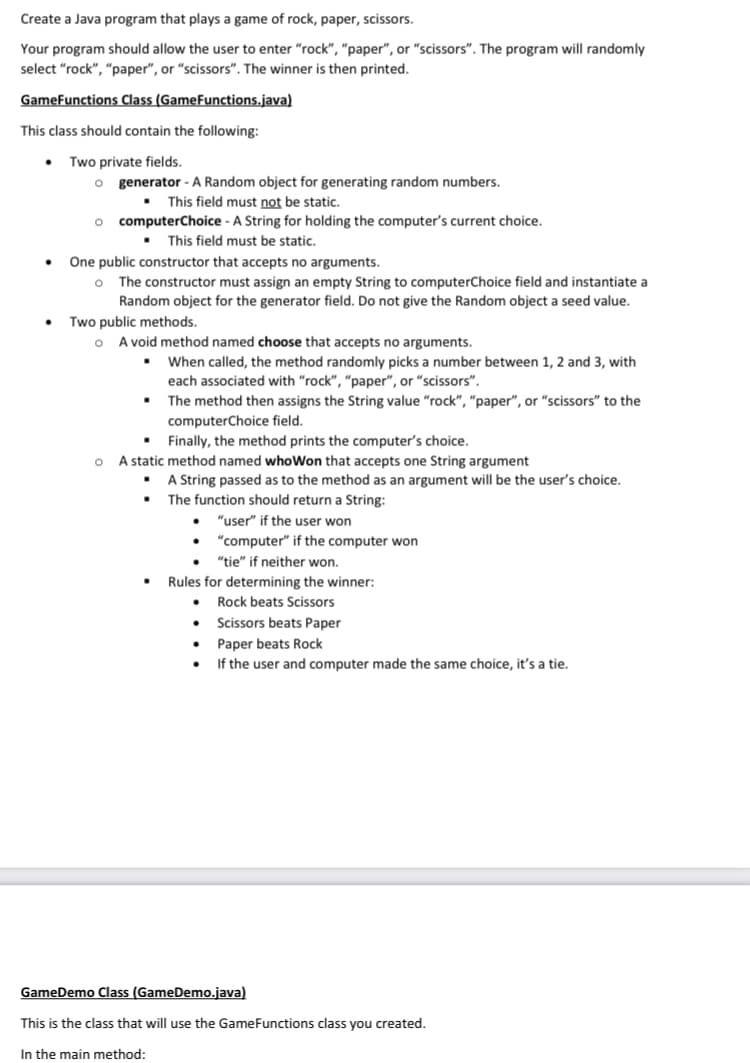
Transcribed Image Text:Create a Java program that plays a game of rock, paper, scissors.
Your program should allow the user to enter "rock", “paper", or "scissors". The program will randomly
select "rock", "paper", or "scissors". The winner is then printed.
GameFunctions Class (GameFunctions.java)
This class should contain the following:
Two private fields.
generator - A Random object for generating random numbers.
This field must not be static.
computerChoice - A String for holding the computer's current choice.
This field must be static.
One public constructor that accepts no arguments.
The constructor must assign an empty String to computerChoice field and instantiate a
Random object for the generator field. Do not give the Random object a seed value.
Two public methods.
A void method named choose that accepts no arguments.
When called, the method randomly picks a number between 1, 2 and 3, with
each associated with "rock", "paper", or "scissors".
The method then assigns the String value "rock", “paper", or "scissors" to the
computerChoice field.
Finally, the method prints the computer's choice.
A static method named whoWon that accepts one String argument
A String passed as to the method as an argument will be the user's choice.
The function should return a String:
"user" if the user won
"computer" if the computer won
"tie" if neither won.
Rules for determining the winner:
Rock beats Scissors
Scissors beats Paper
Paper beats Rock
If the user and computer made the same choice, it's a tie.
GameDemo Class (GameDemo.java)
This is the class that will use the GameFunctions class you created.
In the main method:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
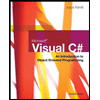
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
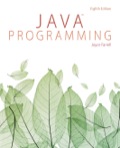
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
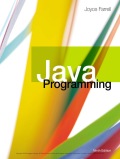
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
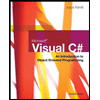
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
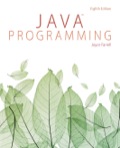
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
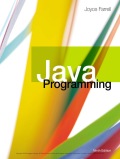
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT