Create a new version of the restaurant ordering program BELOW that uses lists to look up menu items, record the items ordered by the user, and print a full receipt. The following requirements: a. Use a list to look up the names of the menu items; call this list menu_items. This list will replace the use of the multi-way condition. Create the list with the names of the menu items, in the order they appear in the program you created for Part X of Assignment Y. Notice that, because the first position in a list is zero (0), item number 1 in the menu is stored in menu_items[0].
Improved Taking a Restaurant Order Program.
Create a new version of the restaurant ordering program BELOW that uses lists to look up menu items, record the items ordered by the user, and print a full receipt. The following requirements:
a. Use a list to look up the names of the menu items; call this list menu_items. This list will replace the use of the multi-way condition. Create the list with the names of the menu items, in the order they appear in the program you created for Part X of Assignment Y. Notice that, because the first position in a list is zero (0), item number 1 in the menu is stored in menu_items[0].
b. Use a list to look up the prices of the menu items; call this list menu_prices. This list will also replace the use of a multi-way condition to obtain this information. Create the list with the prices of the menu items. Make sure this list aligns with the list in part (a) above. That is, for index value item_nro, the price in menu_prices[item_nro] corresponds to the dish in menu_item[item_nro].
c. Use a list to record the items ordered by the user; call this list ordered_items. This list contains the items’ menu numbers, not their names.
d. Use a list to record the quantity of each item ordered; call this list ordered_quantity. Make sure this list aligns with the ordered_items list. That is, for example, if the customer first orders 3 Cheese Sandwiches (item 7 in the menu), and then 2 Sodas (item 10), then ordered_items[0] is 6, ordered_quantity[0] is 3, ordered_items[1] is 9, and ordered_quantity[1] is 2.
e. After taking an order, the program prints a full receipt, listing the items ordered, quantities, and prices, together with subtotal, tax, tip, and grand total. You can look at a sample receipt from the restaurant you selected, or search online for “restaurant receipt,” for the overall layout of the receipt.
import sys
#Define the function Menu that displays the menu
def Menu():
print("Menu Card")
print("1. Cajun Calamari price is $9.00")
print("2. Shrimp Cocktail price is $9.00")
print("3. Spicy Garlic Mussels price is $9.00")
print("4. Crispy Ahi price is $10.50")
print("5. Spinach Artichoke Dip price is $8.00")
print("6. Steamed Clams price is $10.00")
print("7. Chips and Dip price is $6.50")
print("8. Beer Battered Ribs price is $6.00")
print("9. St.Louis Ribs price is $8.00")
print("10. Mozzarella Sticks price is $7.00")
#Define the function that read the user input and return the subtotal
def userInputCalc():
prices = 0
subtotal =0
continueLoop ="y"
#Enter number for each dish and price for users
while continueLoop =="y":
DishNumber= int(input("\nEnter numbers between 1 and 10:"))
if DishNumber == 1:
prices = 9.0
print("Cajun Calamari price is $",prices)
elif DishNumber== 2:
prices = 9.0
print("Shrimp Cocktail price is $",prices)
elif DishNumber== 3:
prices = 9.0
print("Spicy Garlic Mussels price is $",prices)
elif DishNumber== 4:
prices = 10.50
print("Crispy Ahi price is $",prices)
elif DishNumber== 5:
prices = 8.0
print("Spinach Artichoke Dip price is $",prices)
elif DishNumber== 6:
prices = 10.0
print("Steamed Clams price is $",prices)
elif DishNumber== 7:
prices = 6.50
print("Chips and Dip price is $",prices)
elif DishNumber== 8:
prices = 6.0
print("Beer Battered Ribs price is $",prices)
elif DishNumber== 9:
prices = 8.0
print("St.Louis Ribs price is $",prices)
elif DishNumber== 10:
prices = 7.0
print("Mozzarella Sticks price is $",prices)
else:
print("You have selected an invalid input.")
continueLoop = input("\nEnter y to Continue or n to quit:")
subtotal = subtotal + prices
return subtotal
#Calculate tax, total, tip
def total(subtotal):
sales_tax = subtotal*0.085
subtotal = subtotal + sales_tax
tip = subtotal*0.15
grand_total = subtotal + tip
return sales_tax, grand_total, tip
#Define the function that print receipt
def printReceipt(subtotal, sales_tax, grand_total, tip):
print("\n------Reciept------")
print("\nSubtotal: ",subtotal,"$")
print("Sales tax : ",sales_tax,"$")
print("Grand total : ",grand_total,"$")
print("Suggested tip : ",tip,"$")
#Define the main function
def main():
Menu()
subtotal = userInputCalc()
sales_tax, grand_total, tip =total(subtotal)
printReceipt(subtotal, sales_tax, grand_total, tip)
main()

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

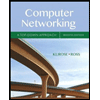
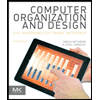
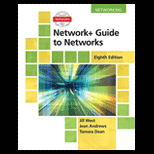
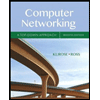
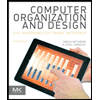
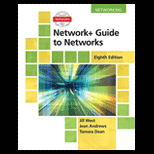
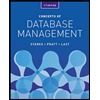
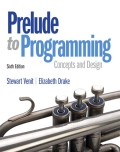
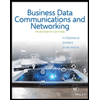