A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings), separated by a comma. That list is followed by a name, and your program should output the phone number associated with that name. Assume that the list will always contain less than 20 word pairs.
Please read the directions and check my java code and help me to solve...
My code said error...
My code:
import java.util.Scanner;
public class LabProgram {
public static String getPhoneNumber(String[] nameArr, String[] phoneNumberArr, String contactName, int arraySize) {
//Looping
for(int i = 0; i < arraySize; i++) {
//Seaching contact name
if(nameArr[i].equals(contactName))
//If found return the phone number
return phoneNumberArr[i];
}
//otherwise return error message
return "The contact name is not found.";
}
//Defining main()
public static void main(String[] args) {
//Creating scanner object
Scanner input = new Scanner(System.in);
Scanner in = new Scanner(System.in);
//Getting list size
int n=input.nextInt();
//Based on size creating name and phone number array
String[] nameArr = new String[n];
String[] phoneNumberArr = new String[n];
//Name to be searched
String ContactName;
//Looping
for(int i = 0; i < n; i++) {
//Getting input
nameArr[i] = input.next();
phoneNumberArr[i] = in.next();
}
//Getting input for search contact name
ContactName = input.next();
//Calling and printing the result
System.out.println(getPhoneNumber(nameArr, phoneNumberArr, ContactName, n));
input.close();
}
}
![7.7 LAB: Contact list
A contact list is a place where you can store a specific contact with other associated information such as a phone
number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the
number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings),
separated by a comma. That list is followed by a name, and your program should output the phone number
associated with that name. Assume that the list will always contain less than 20 word pairs.
Ex: If the input is:
3 Joe, 123-5432 Linda, 983-4123 Frank,867-5309 Frank
the output is:
867-5309
Your program must define and call the following method. The return value of getPhoneNumber() is the phone
number associated with the specific contact name.
public static String getPhoneNumber (String [] nameArr, String [] phoneNumberArr, String contactName,
int arraySize)
Hint: Use two arrays: One for the string names, and the other for the string phone numbers.
346124.2040586.qx3zqy7](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Feb5afa1b-1353-498c-8a28-0fcb52042fa5%2F73531351-7a81-48e5-9a41-bf60b2f1ebe9%2F1ujwyhj_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

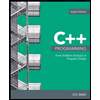
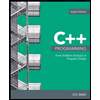