Create a php file for this task. Depending on whether "Top Scorers" or "Leaders" has been clicked, you need to display the top 10 teams sorted by their total scores or total points earned. You have to read the whole CSV file when calculating total scores and total points. Top Scorers Display a table with two columns: Team and Scores. The team that has the highest number of scores is displayed first. You have to display the top 10 teams only. Note: in the CSV file, for the first row, you will see something like Arsenal FC,4-3,Leicester City FC that means for this particular match, Arsenal FC scored 4 and Leicester City FC scored 3. This rule applies to all other rows as well. For this "Top Scorers" display, you need to calculate the total score of every team and then display the top 10 teams. Leaders Display a table with two columns: Team and Points. The team that has the highest number of points is displayed first. You have to display the top 10 teams only. Note: in the CSV file, for the first row, you will see something like Arsenal FC,4-3,Leicester City FC that means for this particular match, Arsenal FC scored 4 and Leicester City FC scored 3, so Arsenal FC wins this match. For a match, the winner earns 3 points, the loser earns zero points. If the match is a draw, both teams earn 1 point. This rule applies to all other rows as well. For this "Leaders" display, you need to calculate the total point of every team and then display the top 10 teams. https://raw.githubusercontent.com/TriDang/cosc2430-2022-s2/main/data/football.csv Hint 1: maintain an associative array like this [ 'team 1 name' => ['scores' => calculated_score, 'points' => 'calculated points'], 'team 2 name' => ['scores' => calculated_score, 'points' => 'calculated points'], 'team 3 name' => ['scores' => calculated_score, 'points' => 'calculated points'] ] In other words, use team names as the keys for the array. The value of each key is itself another array Hint 2: loop through the CSV file If the team name has not existed yet => add a new array element and assign calculated scores and calculated points as zeros. Extract the FT column to get the score of each team. Add the score to each team Based on the score of each team, determine the winner, loser, or drawer. Add appropriate points to each team Hint 3: now you have an array of teams with all the needed information, just create 2 comparison functions and use the sort function to sort the teams. Hint 4: use fgetcsv() to read each row; use explode() to extract the score of each team.
Create a php file for this task. Depending on whether "Top Scorers" or "Leaders" has been clicked, you need to display the top 10 teams sorted by their total scores or total points earned. You have to read the whole CSV file when calculating total scores and total points.
Top Scorers
Display a table with two columns: Team and Scores. The team that has the highest number of scores is displayed first. You have to display the top 10 teams only.
Note: in the CSV file, for the first row, you will see something like
Arsenal FC,4-3,Leicester City FC
that means for this particular match, Arsenal FC scored 4 and Leicester City FC scored 3. This rule applies to all other rows as well. For this "Top Scorers" display, you need to calculate the total score of every team and then display the top 10 teams.
Leaders
Display a table with two columns: Team and Points. The team that has the highest number of points is displayed first. You have to display the top 10 teams only.
Note: in the CSV file, for the first row, you will see something like
Arsenal FC,4-3,Leicester City FC
that means for this particular match, Arsenal FC scored 4 and Leicester City FC scored 3, so Arsenal FC wins this match. For a match, the winner earns 3 points, the loser earns zero points. If the match is a draw, both teams earn 1 point. This rule applies to all other rows as well. For this "Leaders" display, you need to calculate the total point of every team and then display the top 10 teams.
https://raw.githubusercontent.com/TriDang/cosc2430-2022-s2/main/data/football.csv
Hint 1: maintain an associative array like this
[
'team 1 name' => ['scores' => calculated_score, 'points' => 'calculated points'],
'team 2 name' => ['scores' => calculated_score, 'points' => 'calculated points'],
'team 3 name' => ['scores' => calculated_score, 'points' => 'calculated points']
]
In other words, use team names as the keys for the array. The value of each key is itself another array
Hint 2: loop through the CSV file
If the team name has not existed yet => add a new array element and assign calculated scores and calculated points as zeros.
Extract the FT column to get the score of each team. Add the score to each team
Based on the score of each team, determine the winner, loser, or drawer. Add appropriate points to each team
Hint 3: now you have an array of teams with all the needed information, just create 2 comparison functions and use the sort function to sort the teams.
Hint 4: use fgetcsv() to read each row; use explode() to extract the score of each team.

Step by step
Solved in 5 steps with 3 images

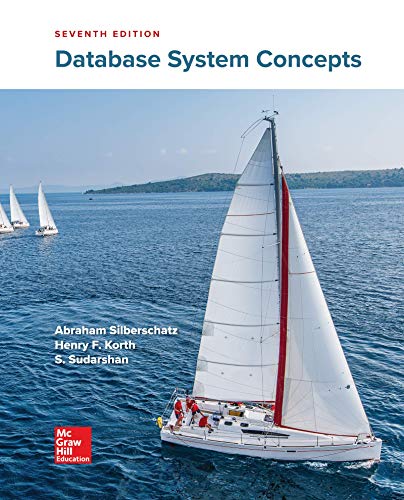
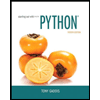
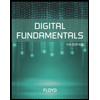
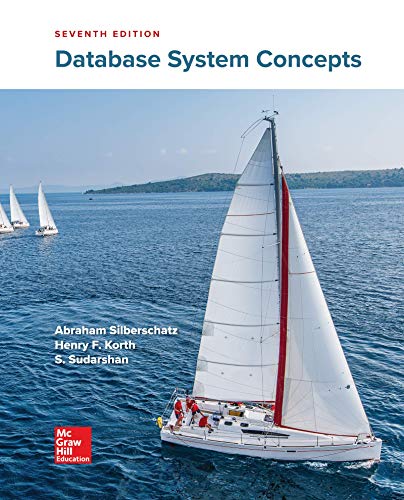
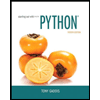
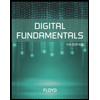
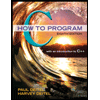
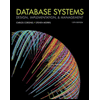
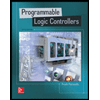