create an interface in java that contains the following methods: boolean myPush(T element); // pushes element onto the stack; returns true if element successfully pushed, false otherwise boolean myPop(); // removes the top element of the stack; returns true if element successfully popped, false otherwise T myTop(); // if the stack is not empty returns the top element of the stack, otherwise returns null String toString(); // returns contents of the stack as a single String int size(); // return number of elements in the stack then create java file that Implements your interface that you created as a linked stack, then use the driver shown in screen shot to test. below is the code for the LLNode class public class LLNode { protected LLNode link; protected T info; public LLNode(T info) { this.info = info; link = null; } public void setInfo(T info) { this.info = info; } public T getInfo() { return info; } public void setLink(LLNode link) { this.link = link; } public LLNode getLink() { return link; } } program should output fruits cost = 35
how to create an interface in java that contains the following methods:
boolean myPush(T element); // pushes element onto the stack; returns true if element successfully pushed, false otherwise
boolean myPop(); // removes the top element of the stack; returns true if element successfully popped, false otherwise
T myTop(); // if the stack is not empty returns the top element of the stack, otherwise returns null
String toString(); // returns contents of the stack as a single String
int size(); // return number of elements in the stack
then create java file that Implements your interface that you created as a linked stack, then use the driver shown in screen shot to test. below is the code for the LLNode class
public class LLNode<T> {
protected LLNode<T> link;
protected T info;
public LLNode(T info) {
this.info = info;
link = null;
}
public void setInfo(T info) {
this.info = info;
}
public T getInfo() {
return info;
}
public void setLink(LLNode<T> link) {
this.link = link;
}
public LLNode<T> getLink() {
return link;
}
}
fruits cost = 35
![public class driver {
public static void main(String[] args) {
mxLinkedstack<String> a = new mybiakedStack<Stringl);
int n = 0;
if (asizel) == 0)
n += 5;
if (amueush("Apple"))
n += 5;
if (atostring().eaualslenereCase("Apple\n"))
n += 5;
if (asizel) == 1)
n += 5;
if (amxPush("Orange") && asize() :
== 2 &&
atostring()-eaualslgnereCase("Orange \aAReleIn"))
n += 5;
if (amxTe().eaualslenereCasel"Orange"))
n += 5;
while (amxlea()) {
}
if (amxTog() == null)
n += 5;
Sustemeuterintla("fruits cost =" +n);
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1b2c1e36-9974-49eb-8a0c-fe4d3c635f06%2Ff77ad18b-2096-4bc4-a4ec-b4aa5b5739b2%2Foj4vmcc_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

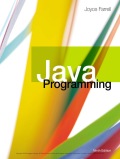
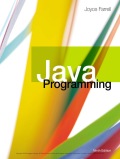