Two stacks are the same if they have the same size and their elements at the corresponding positions are the same. Add the method equalStack to Class StackClass that takes as a parameter a StackClass object, say otherStack and return true if the stack is the same as otherStack. Also write the definition of method equalStack and a program to test your method. In StackClass.java, please finish method - public boolean equalStack(StackClass otherStack) and testing main program – Problem52.java. (Download Problem52.zip to complete this problem) Note: java programming, please use given outline and use given class. also explain in detatil public class Problem52 { publicstaticvoidmain(String[] args) { StackClass intStack = newStackClass(50); StackClass tempStack = newStackClass(50); //Dr. Yu -- Please add your code here! } } public class StackClass implements StackADT { privateintmaxStackSize; //variable to store the //maximum stack size privateintstackTop; //variable to point to //the top of the stack privateT[] list; //array of reference variables //Default constructor //Create an array of size 100 to implement the stack. //Postcondition: The variable list contains the base // address of the array, stackTop = 0, // and maxStackSize = 100. publicStackClass() { maxStackSize = 100; stackTop = 0; //set stackTop to 0 list = (T[]) new Object[maxStackSize]; //create the array }//end default constructor //Constructor with a parameter //Create an array of size stackSize to implement the //stack. //Postcondition: The variable list contains the base // address of the array, stackTop = 0, // and maxStackSize = stackSize. publicStackClass(intstackSize) { if (stackSize <= 0) { System.err.println("The size of the array to " + "implement the stack must be " + "positive."); System.err.println("Creating an array of size 100."); maxStackSize = 100; } else maxStackSize = stackSize; //set the stack size to //the value specified by //the parameter stackSize stackTop = 0; //set stackTop to 0 list = (T[]) new Object[maxStackSize]; //create the array }//end constructor //Method to initialize the stack to an empty state. //Postcondition: stackTop = 0 publicvoidinitializeStack() { for (inti = 0; i < stackTop; i++) list[i] = null; stackTop = 0; }//end initializeStack //Method to determine whether the stack is empty. //Postcondition: Returns true if the stack is empty; // otherwise, returns false. publicbooleanisEmptyStack() { return (stackTop == 0); }//end isEmptyStack //Method to determine whether the stack is full. //Postcondition: Returns true if the stack is full; // otherwise, returns false. publicbooleanisFullStack() { return (stackTop == maxStackSize); }//end isFullStack //Method to add newItem to the stack. //Precondition: The stack exists and is not full. //Postcondition: The stack is changed and newItem // is added to the top of stack. // If the stack is full, the method // throws StackOverflowException publicvoidpush(TnewItem) throwsStackOverflowException { if (isFullStack()) thrownewStackOverflowException(); list[stackTop] = newItem; //add newItem at the //top of the stack stackTop++; //increment stackTop }//end push //Method to return a reference to the top element of //the stack. //Precondition: The stack exists and is not empty. //Postcondition: If the stack is empty, the method // throws StackUnderflowException; // otherwise, a reference to the top // element of the stack is returned. publicTpeek() throwsStackUnderflowException { if (isEmptyStack()) thrownewStackUnderflowException(); return (T) list[stackTop - 1]; }//end peek //Method to remove the top element of the stack. //Precondition: The stack exists and is not empty. //Postcondition: The stack is changed and the top // element is removed from the stack. // If the stack is empty, the method // throws StackUnderflowException publicvoidpop() throwsStackUnderflowException { if (isEmptyStack()) thrownewStackUnderflowException(); stackTop--; //decrement stackTop list[stackTop] = null; }//end pop publicbooleanequalStack(StackClass otherStack) { //Dr. Yu -- Please add your code here! } //end equalStack } public class StackException extends RuntimeException { publicStackException() { } publicStackException(Stringmsg) { super(msg); } } public class StackOverflowException extends StackException { publicStackOverflowException() { super("Stack Overflow"); } publicStackOverflowException(Stringmsg) { super(msg); } } public class StackUnderflowException extends StackException { publicStackUnderflowException() { super("Stack Underflow"); } publicStackUnderflowException(Stringmsg) { super(msg); } }
Two stacks are the same if they have the same size and their elements at the corresponding positions are the same. Add the method equalStack to Class StackClass that takes as a parameter a StackClass object, say otherStack and return true if the stack is the same as otherStack. Also write the definition of method equalStack and a program to test your method. In StackClass.java, please finish method - public boolean equalStack(StackClass otherStack) and testing main program – Problem52.java. (Download Problem52.zip to complete this problem) Note: java programming, please use given outline and use given class. also explain in detatil public class Problem52 { publicstaticvoidmain(String[] args) { StackClass intStack = newStackClass(50); StackClass tempStack = newStackClass(50); //Dr. Yu -- Please add your code here! } } public class StackClass implements StackADT { privateintmaxStackSize; //variable to store the //maximum stack size privateintstackTop; //variable to point to //the top of the stack privateT[] list; //array of reference variables //Default constructor //Create an array of size 100 to implement the stack. //Postcondition: The variable list contains the base // address of the array, stackTop = 0, // and maxStackSize = 100. publicStackClass() { maxStackSize = 100; stackTop = 0; //set stackTop to 0 list = (T[]) new Object[maxStackSize]; //create the array }//end default constructor //Constructor with a parameter //Create an array of size stackSize to implement the //stack. //Postcondition: The variable list contains the base // address of the array, stackTop = 0, // and maxStackSize = stackSize. publicStackClass(intstackSize) { if (stackSize <= 0) { System.err.println("The size of the array to " + "implement the stack must be " + "positive."); System.err.println("Creating an array of size 100."); maxStackSize = 100; } else maxStackSize = stackSize; //set the stack size to //the value specified by //the parameter stackSize stackTop = 0; //set stackTop to 0 list = (T[]) new Object[maxStackSize]; //create the array }//end constructor //Method to initialize the stack to an empty state. //Postcondition: stackTop = 0 publicvoidinitializeStack() { for (inti = 0; i < stackTop; i++) list[i] = null; stackTop = 0; }//end initializeStack //Method to determine whether the stack is empty. //Postcondition: Returns true if the stack is empty; // otherwise, returns false. publicbooleanisEmptyStack() { return (stackTop == 0); }//end isEmptyStack //Method to determine whether the stack is full. //Postcondition: Returns true if the stack is full; // otherwise, returns false. publicbooleanisFullStack() { return (stackTop == maxStackSize); }//end isFullStack //Method to add newItem to the stack. //Precondition: The stack exists and is not full. //Postcondition: The stack is changed and newItem // is added to the top of stack. // If the stack is full, the method // throws StackOverflowException publicvoidpush(TnewItem) throwsStackOverflowException { if (isFullStack()) thrownewStackOverflowException(); list[stackTop] = newItem; //add newItem at the //top of the stack stackTop++; //increment stackTop }//end push //Method to return a reference to the top element of //the stack. //Precondition: The stack exists and is not empty. //Postcondition: If the stack is empty, the method // throws StackUnderflowException; // otherwise, a reference to the top // element of the stack is returned. publicTpeek() throwsStackUnderflowException { if (isEmptyStack()) thrownewStackUnderflowException(); return (T) list[stackTop - 1]; }//end peek //Method to remove the top element of the stack. //Precondition: The stack exists and is not empty. //Postcondition: The stack is changed and the top // element is removed from the stack. // If the stack is empty, the method // throws StackUnderflowException publicvoidpop() throwsStackUnderflowException { if (isEmptyStack()) thrownewStackUnderflowException(); stackTop--; //decrement stackTop list[stackTop] = null; }//end pop publicbooleanequalStack(StackClass otherStack) { //Dr. Yu -- Please add your code here! } //end equalStack } public class StackException extends RuntimeException { publicStackException() { } publicStackException(Stringmsg) { super(msg); } } public class StackOverflowException extends StackException { publicStackOverflowException() { super("Stack Overflow"); } publicStackOverflowException(Stringmsg) { super(msg); } } public class StackUnderflowException extends StackException { publicStackUnderflowException() { super("Stack Underflow"); } publicStackUnderflowException(Stringmsg) { super(msg); } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Two stacks are the same if they have the same size and their elements at the corresponding positions are the same. Add the method equalStack to Class StackClass that takes as a parameter a StackClass object, say otherStack and return true if the stack is the same as otherStack. Also write the definition of method equalStack and a program to test your method. In StackClass.java, please finish method - public boolean equalStack(StackClass<T> otherStack) and testing main program – Problem52.java. (Download Problem52.zip to complete this problem)
Note: java programming, please use given outline and use given class. also explain in detatil
public class Problem52
{
publicstaticvoidmain(String[] args)
{
StackClass<Integer> intStack = newStackClass<Integer>(50);
StackClass<Integer> tempStack = newStackClass<Integer>(50);
//Dr. Yu -- Please add your code here!
}
}
public class StackClass<T> implements StackADT<T>
{
privateintmaxStackSize; //variable to store the
//maximum stack size
privateintstackTop; //variable to point to
//the top of the stack
privateT[] list; //array of reference variables
//Default constructor
//Create an array of size 100 to implement the stack.
//Postcondition: The variable list contains the base
// address of the array, stackTop = 0,
// and maxStackSize = 100.
publicStackClass()
{
maxStackSize = 100;
stackTop = 0; //set stackTop to 0
list = (T[]) new Object[maxStackSize]; //create the array
}//end default constructor
//Constructor with a parameter
//Create an array of size stackSize to implement the
//stack.
//Postcondition: The variable list contains the base
// address of the array, stackTop = 0,
// and maxStackSize = stackSize.
publicStackClass(intstackSize)
{
if (stackSize <= 0)
{
System.err.println("The size of the array to "
+ "implement the stack must be "
+ "positive.");
System.err.println("Creating an array of size 100.");
maxStackSize = 100;
}
else
maxStackSize = stackSize; //set the stack size to
//the value specified by
//the parameter stackSize
stackTop = 0; //set stackTop to 0
list = (T[]) new Object[maxStackSize]; //create the array
}//end constructor
//Method to initialize the stack to an empty state.
//Postcondition: stackTop = 0
publicvoidinitializeStack()
{
for (inti = 0; i < stackTop; i++)
list[i] = null;
stackTop = 0;
}//end initializeStack
//Method to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty;
// otherwise, returns false.
publicbooleanisEmptyStack()
{
return (stackTop == 0);
}//end isEmptyStack
//Method to determine whether the stack is full.
//Postcondition: Returns true if the stack is full;
// otherwise, returns false.
publicbooleanisFullStack()
{
return (stackTop == maxStackSize);
}//end isFullStack
//Method to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
// is added to the top of stack.
// If the stack is full, the method
// throws StackOverflowException
publicvoidpush(TnewItem) throwsStackOverflowException
{
if (isFullStack())
thrownewStackOverflowException();
list[stackTop] = newItem; //add newItem at the
//top of the stack
stackTop++; //increment stackTop
}//end push
//Method to return a reference to the top element of
//the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the method
// throws StackUnderflowException;
// otherwise, a reference to the top
// element of the stack is returned.
publicTpeek() throwsStackUnderflowException
{
if (isEmptyStack())
thrownewStackUnderflowException();
return (T) list[stackTop - 1];
}//end peek
//Method to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
// element is removed from the stack.
// If the stack is empty, the method
// throws StackUnderflowException
publicvoidpop() throwsStackUnderflowException
{
if (isEmptyStack())
thrownewStackUnderflowException();
stackTop--; //decrement stackTop
list[stackTop] = null;
}//end pop
publicbooleanequalStack(StackClass<T> otherStack)
{
//Dr. Yu -- Please add your code here!
} //end equalStack
}
public class StackException extends RuntimeException
{
publicStackException()
{
}
publicStackException(Stringmsg)
{
super(msg);
}
}
public class StackOverflowException extends StackException
{
publicStackOverflowException()
{
super("Stack Overflow");
}
publicStackOverflowException(Stringmsg)
{
super(msg);
}
}
public class StackUnderflowException extends StackException
{
publicStackUnderflowException()
{
super("Stack Underflow");
}
publicStackUnderflowException(Stringmsg)
{
super(msg);
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
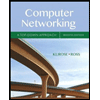
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
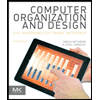
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
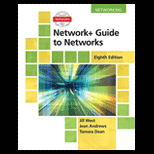
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
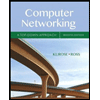
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
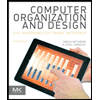
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
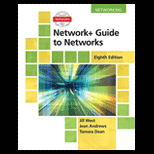
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
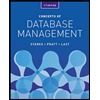
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
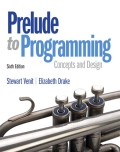
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
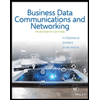
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY