ctive of this lab is to create the beginnings for a python-based card game. We DO NOT expect a fully functioning card game. What we do expect is that you main function and various functions that will accomplish the following goals: la single-dimension array to keep track of the location of every card NOT move cards around. Just use the array to keep track of where each card is ard data is really integers - Use other arrays to translate integers to suits, ranks, and player names ards will start in the DECK e a function that translates a card number to a card name. HINT - look at the suitName and rankName arrays e a function to assign a card to a given player ing a card involves picking a card number and assigning a new location to the corresponding element of cardLoc e a function that displays the location of every card. (Early versions should show numeric values for the card number and location. Later versions can include g values for prettier output.) e a function that prints the name of every card in a given hand er Code egin by copying the following code to your editor. You will not need to change my code at all, (in fact, you may not change the main code) but you will need to eral new functions to make it work correctly. Game.py ic card game framework os track of card locations for as many hands as needed ndom import * 5- 52 - 1 - [0] * NUMCARDS e- ("hearts", "diamonds", "spades", "clubs") e- ("Ace", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King") ame = ("deck", "player", "computer") Deck() in range(5): ignCard(PLAYER)) gnCard(COMP) eck() and(PLAYER) and(COMP)
ctive of this lab is to create the beginnings for a python-based card game. We DO NOT expect a fully functioning card game. What we do expect is that you main function and various functions that will accomplish the following goals: la single-dimension array to keep track of the location of every card NOT move cards around. Just use the array to keep track of where each card is ard data is really integers - Use other arrays to translate integers to suits, ranks, and player names ards will start in the DECK e a function that translates a card number to a card name. HINT - look at the suitName and rankName arrays e a function to assign a card to a given player ing a card involves picking a card number and assigning a new location to the corresponding element of cardLoc e a function that displays the location of every card. (Early versions should show numeric values for the card number and location. Later versions can include g values for prettier output.) e a function that prints the name of every card in a given hand er Code egin by copying the following code to your editor. You will not need to change my code at all, (in fact, you may not change the main code) but you will need to eral new functions to make it work correctly. Game.py ic card game framework os track of card locations for as many hands as needed ndom import * 5- 52 - 1 - [0] * NUMCARDS e- ("hearts", "diamonds", "spades", "clubs") e- ("Ace", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King") ame = ("deck", "player", "computer") Deck() in range(5): ignCard(PLAYER)) gnCard(COMP) eck() and(PLAYER) and(COMP)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Objective
The objective of this lab is to create the beginnings for a python-based card game. We DO NOT expect a fully functioning card game. What we do expect is that you
create a main function and various functions that will accomplish the following goals:
• Build a single-dimension array to keep track of the location of every card
• DO NOT move cards around.. Just use the array to keep track of where each card is
• All card data is really integers - Use other arrays to translate integers to suits, ranks, and player names
• All cards will start in the DECK
• Write a function that translates a card number to a card name. HINT - look at the suitName and rankName arrays
• Write a function to assign a card to a given player
• Dealing a card involves picking a card number and assigning a new location to the corresponding element of cardLoc
• Write a function that displays the location of every card. (Early versions should show numeric values for the card number and location. Later versions can include
string values for prettier output.)
• Write a function that prints the name of every card in a given hand
Starter Code
Please begin by copying the following code to your editor. You will not need to change my code at all, (in fact, you may not change the main code) but you will need to
add several new functions to make it work correctly.
cardGame.py
basic card game framework
keeps track of card locations for as many hands as needed
from random import *
NUMCARDS - 52
DECK - 0
PLAYER - 1
COMP - 2
cardLoc - [0] * NUMCARDS
suitName - ("hearts", "diamonds", "spades", "clubs")
rankName - ("Ace", "Two", "Three", "Four", "Five", "Six", "Seven",
"Eight", "Nine", "Ten", "Jack", "Queen", "King")
playerName = ("deck", "player", "computer")
def main():
clearDeck()
for i in range(5):
assignCard(PLAYER)
assignCard(COMP)
showDeck()
showHand(PLAYER)
showHand(COMP)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5148db4e-d09c-458a-b821-dbbe2cf3fe9b%2Faeed9a46-9e1a-454b-8d80-58597e58ef29%2F8k4jfw8_processed.png&w=3840&q=75)
Transcribed Image Text:Objective
The objective of this lab is to create the beginnings for a python-based card game. We DO NOT expect a fully functioning card game. What we do expect is that you
create a main function and various functions that will accomplish the following goals:
• Build a single-dimension array to keep track of the location of every card
• DO NOT move cards around.. Just use the array to keep track of where each card is
• All card data is really integers - Use other arrays to translate integers to suits, ranks, and player names
• All cards will start in the DECK
• Write a function that translates a card number to a card name. HINT - look at the suitName and rankName arrays
• Write a function to assign a card to a given player
• Dealing a card involves picking a card number and assigning a new location to the corresponding element of cardLoc
• Write a function that displays the location of every card. (Early versions should show numeric values for the card number and location. Later versions can include
string values for prettier output.)
• Write a function that prints the name of every card in a given hand
Starter Code
Please begin by copying the following code to your editor. You will not need to change my code at all, (in fact, you may not change the main code) but you will need to
add several new functions to make it work correctly.
cardGame.py
basic card game framework
keeps track of card locations for as many hands as needed
from random import *
NUMCARDS - 52
DECK - 0
PLAYER - 1
COMP - 2
cardLoc - [0] * NUMCARDS
suitName - ("hearts", "diamonds", "spades", "clubs")
rankName - ("Ace", "Two", "Three", "Four", "Five", "Six", "Seven",
"Eight", "Nine", "Ten", "Jack", "Queen", "King")
playerName = ("deck", "player", "computer")
def main():
clearDeck()
for i in range(5):
assignCard(PLAYER)
assignCard(COMP)
showDeck()
showHand(PLAYER)
showHand(COMP)
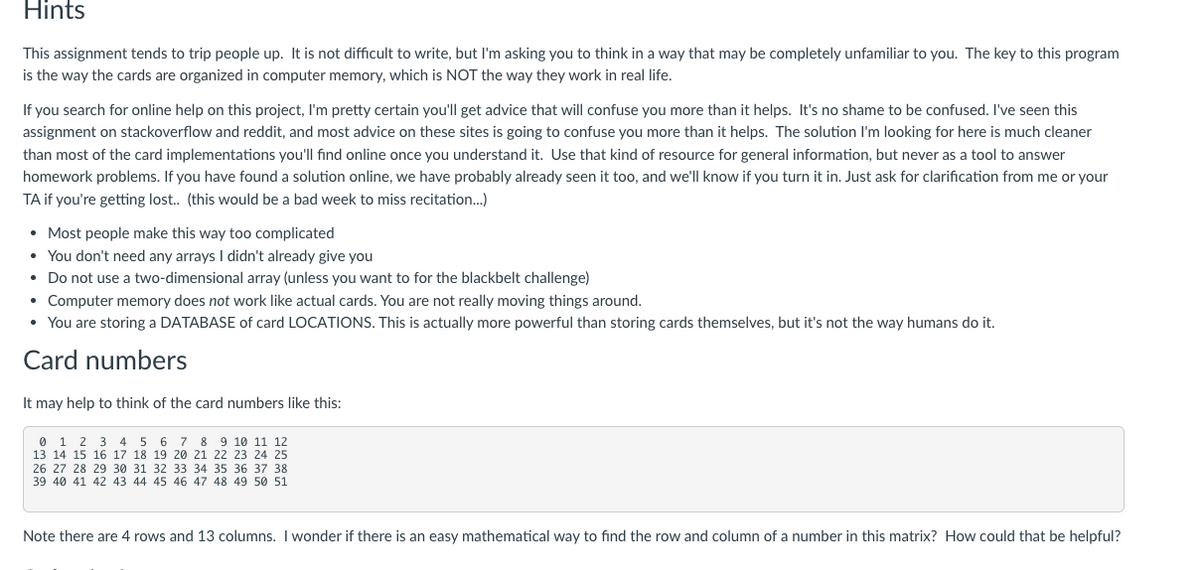
Transcribed Image Text:Hints
This assignment tends to trip people up. It is not difficult to write, but I'm asking you to think in a way that may be completely unfamiliar to you. The key to this program
is the way the cards are organized in computer memory, which is NOT the way they work in real life.
If you search for online help on this project, I'm pretty certain you'll get advice that will confuse you more than it helps. It's no shame to be confused. I've seen this
assignment on stackoverflow and reddit, and most advice on these sites is going to confuse you more than it helps. The solution I'm looking for here is much cleaner
than most of the card implementations you'll find online once you understand it. Use that kind of resource for general information, but never as a tool to answer
homework problems. If you have found a solution online, we have probably already seen it too, and we'll know if you turn it in. Just ask for clarification from me or your
TA if you're getting lost.. (this would be a bad week to miss recitation..)
• Most people make this way too complicated
• You don't need any arrays didn't already give you
• Do not use a two-dimensional array (unless you want to for the blackbelt challenge)
• Computer memory does not work like actual cards. You are not really moving things around.
• You are storing a DATABASE of card LOCATIONS. This is actually more powerful than storing cards themselves, but it's not the way humans do it.
Card numbers
It may help to think of the card numbers like this:
1 2 3 4 5 6 7 8 9 10 11 12
13 14 15 16 17 18 19 20 21 22 23 24 25
26 27 28 29 30 31 32 33 34 35 36 37 38
39 40 41 42 43 44 45 46 47 48 49 50 51
Note there are 4 rows and 13 columns. I wonder if there is an easy mathematical way to find the row and column of a number in this matrix? How could that be helpful?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
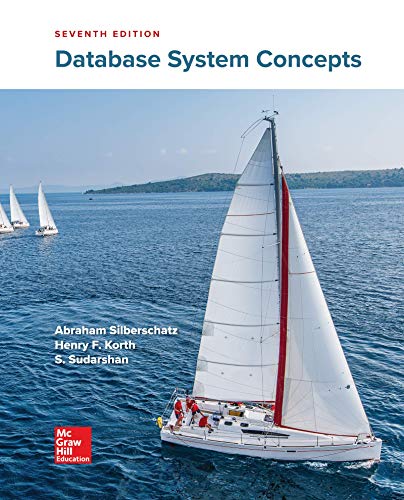
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
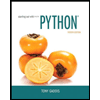
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
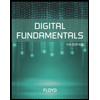
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
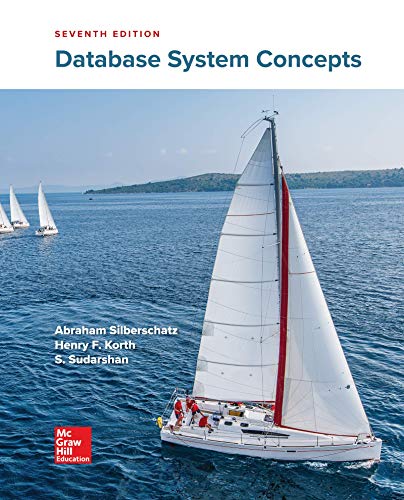
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
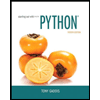
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
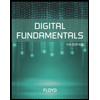
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
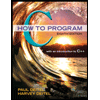
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
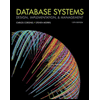
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
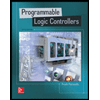
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education