Currently, the client simply sends to the server everything the end-user types. When the server receives these messages it simply echoes them to all clients. Add a mechanism so that the user of the client can type commands that perform special functions. Each command should start with the ’#’ symbol - in fact, anything that starts with that symbol should be considered a command. You should implement the following commands: (a) #quit cause the client to terminate gracefully. Make sure the connection to the server is terminated before exiting the program. (b) #logoff causes the client to disconnect from the server, but not quit. (c) #sethost calls the setHost method in the client. Only allowed if the client is logged off; displays an error message otherwise. (d) #setport calls the setPort method in the client, with the same constraints as #sethost. (e) #login causes the client to connect to the server. Only allowed if the client is not already connected; display an error message otherwise. (f) #gethost displays the current host name. (g) #getport displays the current port number. ChatIF.java (The Java interface used to help isolate the user interface from the functional part of the code.) package common; /** * This interface implements the abstract method used to display * objects onto the client or server UIs. * * @author Dr Robert Laganière * @author Dr Timothy C. Lethbridge * @version July 2000 */ publicinterfaceChatIF { /** * Method that when overriden is used to display objects onto * a UI. */ publicabstractvoiddisplay(Stringmessage); } ChatClient.java. (The functional layer of SimpleChat's client side) package client; import ocsf.client.*; import common.*; import java.io.*; public class ChatClient extends AbstractClient { ChatIF clientUI; public ChatClient(String host, int port, ChatIF clientUI) throws IOException { super(host, port); //Call the superclass constructor this.clientUI = clientUI; openConnection(); } public void handleMessageFromServer(Object msg) { clientUI.display(msg.toString()); } public void handleMessageFromClientUI(String message) { try { sendToServer(message); } catch(IOException e) { clientUI.display ("Could not send message to server. Terminating client."); quit(); } } public void quit() { try { closeConnection(); } catch(IOException e) {} System.exit(0); } } //End of ChatClient class ClientConsole.java. (The textual user interface of SimpleChat's client side) import java.io.*; import client.*; import common.*; publicclassClientConsoleimplementsChatIF { finalpublicstaticintDEFAULT_PORT = 5555; ChatClientclient; publicClientConsole(Stringhost, intport) { try { client= new ChatClient(host, port, this); } catch(IOExceptionexception) { System.out.println("Error: Can't setup connection!" + " Terminating client."); System.exit(1); } } publicvoidaccept() { try { BufferedReaderfromConsole = newBufferedReader(newInputStreamReader(System.in)); Stringmessage; while (true) { message = fromConsole.readLine(); client.handleMessageFromClientUI(message); } } catch (Exceptionex) { System.out.println ("Unexpected error while reading from console!"); } } publicvoiddisplay(Stringmessage) { System.out.println("> " + message); } publicstaticvoidmain(String[] args) { Stringhost = ""; intport = 0; //The port number try { host = args[0]; } catch(ArrayIndexOutOfBoundsExceptione) { host = "localhost"; } ClientConsolechat= newClientConsole(host, DEFAULT_PORT); chat.accept(); //Wait for console data } } //End of ConsoleChat class EchoServer.java.( The server off SimpleChat phase 1, which lacks any capability for user input.) import java.io.*; import ocsf.server.*; publicclassEchoServerextendsAbstractServer { finalpublicstaticintDEFAULT_PORT = 5555; publicEchoServer(intport) { super(port); } publicvoid handleMessageFromClient (Object msg, ConnectionToClient client) { System.out.println("Message received: " + msg + " from " + client); this.sendToAllClients(msg); } /** * This method overrides the one in the superclass. Called * when the server starts listening for connections. */ protectedvoidserverStarted() { System.out.println ("Server listening for connections on port " + getPort()); } /** * This method overrides the one in the superclass. Called * when the server stops listening for connections. */ protectedvoidserverStopped() { System.out.println ("Server has stopped listening for connections."); } //Class methods *************************************************** /** * This method is responsible for the creation of * the server instance (there is no UI in this phase). * * @param args[0] The port number to listen on. Defaults to 5555 * if no argument is entered. */ publicstaticvoidmain(String[] args) { intport = 0; //Port to listen on try { port = Integer.parseInt(args[0]); //Get port from command line } catch(Throwablet) { port = DEFAULT_PORT; //Set port to 5555 } EchoServersv = newEchoServer(port); try { sv.listen(); //Start listening for connections } catch (Exceptionex) { System.out.println("ERROR - Could not listen for clients!"); } } } //End of EchoServer class
Currently, the client simply sends to the server everything the end-user types. When the server receives these messages it simply echoes them to all clients. Add a
ChatIF.java (The Java interface used to help isolate the user interface from the functional part of the code.)

Step by step
Solved in 3 steps with 1 images

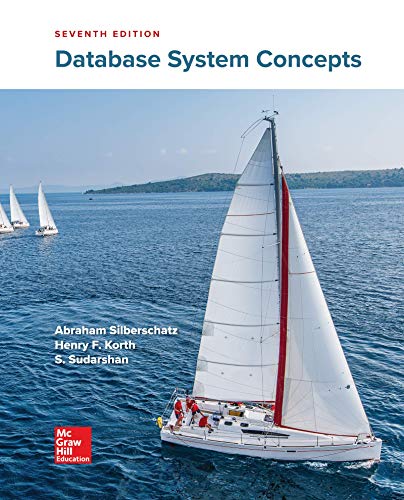
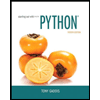
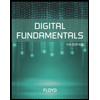
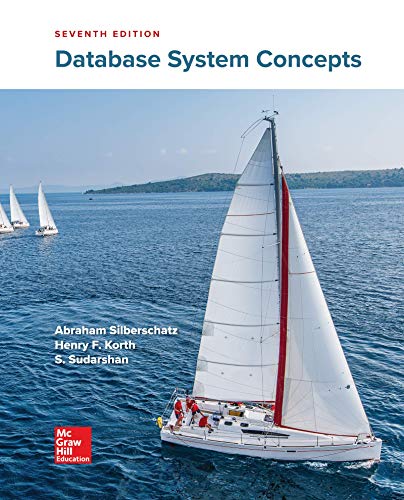
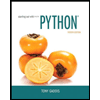
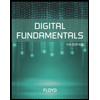
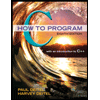
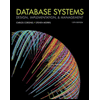
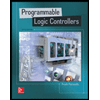