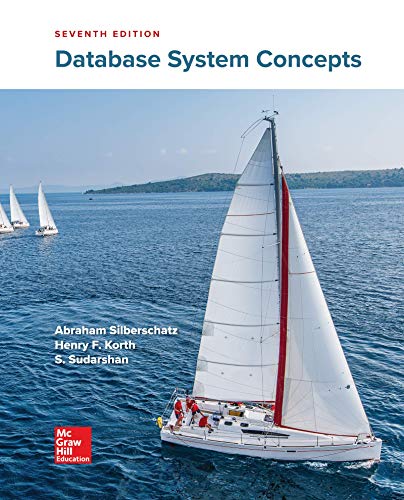
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
thumb_up100%
Java Selection Sort but make it read the data 64, 25, 12, 22, 11 from a file not an array
// Java program for implementation of Selection Sort
class SelectionSort
{
void sort(int arr[])
{
int n = arr.length;
// One by one move boundary of unsorted subarray
for (int i = 0; i < n-1; i++)
{
// Find the minimum element in unsorted array
int min_idx = i;
for (int j = i+1; j < n; j++)
if (arr[j] < arr[min_idx])
min_idx = j;
// Swap the found minimum element with the first
// element
int temp = arr[min_idx];
arr[min_idx] = arr[i];
arr[i] = temp;
}
}
// Prints the array
void printArray(int arr[])
{
int n = arr.length;
for (int i=0; i<n; ++i)
System.out.print(arr[i]+" ");
System.out.println();
}
// Driver code to test above
public static void main(String args[])
{
SelectionSort ob = new SelectionSort();
int arr[] = {64,25,12,22,11}; // make it read from a file instead of having this
ob.sort(arr);
System.out.println("Sorted array");
ob.printArray(arr);
}
}
/* This code is contributed by Rajat Mishra*/
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please run this code for n=6 for arrays 1,34,0,6,78,11 and n=5 for array 8,7,6,5,4 #include <stdio.h>void selection_sort(int arr[], int n) { if (n<=1) return ; int max_id=0; for(int i=0;i<n;i++) { if(arr[i]>arr[max_id]) max_id=i; } int temp=arr[max_id]; arr[max_id]=arr[n-1]; arr[n-1]=temp; selection_sort(arr,n-1); return;}arrow_forwardJAVAsimulate the process of selection sort for array {3,1,2,4}arrow_forwardint [] list = {18,57,8,89,7} and the length = 5 Sort the array using insertion sort algorithm. Fill out the trace table public class InsertionSort { /** The method for sorting the numbers */ public static void insertionSort(int[] list) { for (int i = 1; i < list.length; i++) { /** insert list[i] into a sorted sublist list[0..i-1] so that list[0..i] is sorted. */ int currentElement = list[i]; int k; for (k = i - 1; k >= 0 && list[k] > currentElement; k--) { list[k + 1] = list[k]; } // Insert the current element into list[k+1] list[k + 1] = currentElement; } } /** A test method */ public static void main(String[] args) { int[] list = {18,57,8,89,7}; insertionSort(list); for (int i = 0; i < list.length; i++) System.out.print(list[i] + " "); } }arrow_forward
- Select the for-loop which iterates through all even index values of an array.A. for(int idx = 0; idx < length; idx++)B. for(int idx = 0; idx < length; idx%2)C. for(int idx = 0; idx < length; idx+2)D. for(int idx = 0; idx < length; idx=idx+2)arrow_forwardSort the array using insertion sort algorithm list ={18,57,8,89,7}arrow_forwardoperation of insertion-sort on the array A= {31,41,59,26,41,58}arrow_forward
- The optimised bubble sort offers none of the following benefits over conventional sorts for items that have already been sorted.arrow_forwardIn c language Consider this array unsigned int data [ ] = { 0xA3A4A5A6, 0x12131415 } write a for loop to print the array values in this order 0x1415 0x1213 0xA5A6 0xA3A4arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
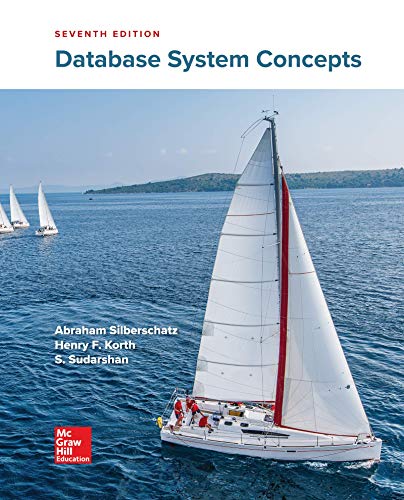
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
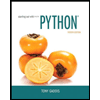
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
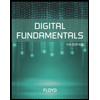
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
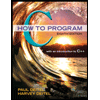
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
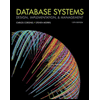
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
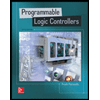
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education