(C++)Write a program that will allow a student to compute for his equivalent semestral grade. The program should ask the user should input his/her first name, last name, middle initial, and student number. After, inputting everything above, the output will clear the current screen and a new screen should open. A sample program that would clear a screen is included below for your reference. On the new screen, the user will then be greeted "Welcome, ! (i.e. Welcome, Louie!)" The program will then ask for the prelim grade, midterm grade and final grade of the student. The program should only accept grades 100 and below. After getting the three grades, the program should compute for the semestral grade. The computation of the semestral grade is as follows: 30%*PrelimGrade + 30%*midtermgrade + 40%finalgrade After inputting all the grades, the screen should clear again and will proceed to another screen. The new screen should output should output the following (this is just a sample output but make sure to follow the format): Name: Louie U. Ferrolino Student Number: 2022333213 Prelim Grade: 100 Midterm Grade: 100 Final Grade: 100 Semestral Grade: 100 Equivalent Grade: 1.00 Remarks: Passed Notes: Remarks should be "Passed" or "Failed" Only. Hint: You may use the relational operators "AND(&&)" and "OR(||)" for your conditions. /**********************SAMPLE PROGRAM THAT CLEARS THE SCREEN ****************************** #include using namespace std; int main() { char fname[32]; cout<<"Enter your first name:"; cin>>fname; cout << "\033[2J\033[1;1H"; //This statement clears the screen after inputting fname and creates a new screen. cout<<"Hi, "</
(C++)Write a program that will allow a student to compute for his equivalent semestral grade. The program should ask the user should input his/her first name, last name, middle initial, and student number. After, inputting everything above, the output will clear the current screen and a new screen should open. A sample program that would clear a screen is included below for your reference. On the new screen, the user will then be greeted "Welcome, ! (i.e. Welcome, Louie!)" The program will then ask for the prelim grade, midterm grade and final grade of the student. The program should only accept grades 100 and below. After getting the three grades, the program should compute for the semestral grade. The computation of the semestral grade is as follows: 30%*PrelimGrade + 30%*midtermgrade + 40%finalgrade After inputting all the grades, the screen should clear again and will proceed to another screen. The new screen should output should output the following (this is just a sample output but make sure to follow the format): Name: Louie U. Ferrolino Student Number: 2022333213 Prelim Grade: 100 Midterm Grade: 100 Final Grade: 100 Semestral Grade: 100 Equivalent Grade: 1.00 Remarks: Passed Notes: Remarks should be "Passed" or "Failed" Only. Hint: You may use the relational operators "AND(&&)" and "OR(||)" for your conditions. /**********************SAMPLE PROGRAM THAT CLEARS THE SCREEN ****************************** #include using namespace std; int main() { char fname[32]; cout<<"Enter your first name:"; cin>>fname; cout << "\033[2J\033[1;1H"; //This statement clears the screen after inputting fname and creates a new screen. cout<<"Hi, "</
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section5.3: Interactive While Loops
Problem 7E
Related questions
Question
(C++)Write a program that will allow a student to compute for his equivalent semestral grade.
- The program should ask the user should input his/her first name, last name, middle initial, and student number.
- After, inputting everything above, the output will clear the current screen and a new screen should open. A sample program that would clear a screen is included below for your reference.
- On the new screen, the user will then be greeted "Welcome, <firstname>! (i.e. Welcome, Louie!)"
- The program will then ask for the prelim grade, midterm grade and final grade of the student. The program should only accept grades 100 and below.
- After getting the three grades, the program should compute for the semestral grade. The computation of the semestral grade is as follows: 30%*PrelimGrade + 30%*midtermgrade + 40%finalgrade
- After inputting all the grades, the screen should clear again and will proceed to another screen.
- The new screen should output should output the following (this is just a sample output but make sure to follow the format):
Name: Louie U. Ferrolino
Student Number: 2022333213
Prelim Grade: 100
Midterm Grade: 100
Final Grade: 100
Semestral Grade: 100
Equivalent Grade: 1.00
Remarks: Passed
Notes:
- Remarks should be "Passed" or "Failed" Only.
Hint:
- You may use the relational operators "AND(&&)" and "OR(||)" for your conditions.
/**********************SAMPLE PROGRAM THAT CLEARS THE SCREEN ******************************
#include <iostream>
using namespace std;
int main()
{
char fname[32];
cout<<"Enter your first name:";
cin>>fname;
cout << "\033[2J\033[1;1H"; //This statement clears the screen after inputting fname and creates a new screen.
cout<<"Hi, "<<fname<<"!";
return 0;
}
*************************************** END OF PROGRAM************************************/
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
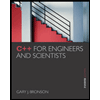
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
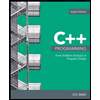
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
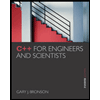
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
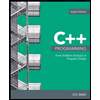
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning