Dart game: Dart location Write a function called DartNumbers() that takes the location of darts thrown as an input, and finds the number of darts that hit the regions yellow, red, blue, and green. A 20x20 square dart board, as shown in the image below, consists of concentric circles with the center at (0,0). The radius of the yellow circle is 3, the red circle is 5, and the blue circle is 10. A dart player throws 10 darts at the board randomly such that any place on the board can be hit with an equal chance. The rand() function can be used to obtain a number with uniform distribution between -10 and 10. Uniform distribution with a given interval means that an equal probability exists to get a number in that interval. Dart Board 10 The inputs of the function DartNumbers() are: throwX: Indicates the x positions of the darts on the board. throwX is a 1x10 array of random numbers between -10 and 10 with a uniform distribution using the rand function. throwY: Indicates the y positions of the darts on the board. throwY is a 1x10 array of random numbers between -10 and 10 with a uniform distribution using the rand function.
Dart game: Dart location Write a function called DartNumbers() that takes the location of darts thrown as an input, and finds the number of darts that hit the regions yellow, red, blue, and green. A 20x20 square dart board, as shown in the image below, consists of concentric circles with the center at (0,0). The radius of the yellow circle is 3, the red circle is 5, and the blue circle is 10. A dart player throws 10 darts at the board randomly such that any place on the board can be hit with an equal chance. The rand() function can be used to obtain a number with uniform distribution between -10 and 10. Uniform distribution with a given interval means that an equal probability exists to get a number in that interval. Dart Board 10 The inputs of the function DartNumbers() are: throwX: Indicates the x positions of the darts on the board. throwX is a 1x10 array of random numbers between -10 and 10 with a uniform distribution using the rand function. throwY: Indicates the y positions of the darts on the board. throwY is a 1x10 array of random numbers between -10 and 10 with a uniform distribution using the rand function.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![The outputs of the function DartNumbers consist of four numerical scalars (ex: a 1x1 numerical array):
yellow: A numerical scalar of the number of darts in the yellow region.
red: A numerical scalar of the number of darts in the red region.
blue: A numerical scalar of the number of darts in the blue region.
green: A numerical scalar of the number of darts in the green region.
Hint: For each point of throwX and throwY, calculate the radius r =
Vx² + y², which is the distance from the center (0,0) to the point (x,y).
Use this radius array and logical indexing to find the number of points in each region. The radii of the three circles on
dart board are 3,
5, and 10. Thus, if a point has distance 3.45, the point lies between circles of radius 3 and 5, which is the red region.
Restrictions: Do not use if statements or switch statements. Logical indexing should be used.
Ex:
For random values of inputs,
throwX
[ 6.2945
8.1158
-7.4603
8.2675
2.6472
-8.0492
-4.4300
0.9376
9.1501
9.2978]
throwY
[-6.8477
9.4119
9.1433
-0.2925
6.0056
-7.1623
-1.5648
8.3147
5.8441
9.1898]
From throwX, throwY, the first dart has x,y coordinates as (6.2945, -6.8477), and the 10th dart has x,y coordinates as (9.2978, 9.1898).
On calling
[yellow, red, blue, green] = DartNumbers(throwX,throwY)
%3D
The output is:
yellow
red = 1
blue
4
%3D
green
5
The output indicates that for given random arrays of throwX and throwY, out of total 10 darts thrown, 0 hit the board in the yellow region, 1
hits the board in the red region, 4 hit the board in the blue region, and 5 hit the board in the green region.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8de9ecd0-3cc9-475b-b184-ad0ee68f5a8e%2F32965b48-64a9-4b77-b89d-2652fd208b81%2Fg0fg8zi_processed.png&w=3840&q=75)
Transcribed Image Text:The outputs of the function DartNumbers consist of four numerical scalars (ex: a 1x1 numerical array):
yellow: A numerical scalar of the number of darts in the yellow region.
red: A numerical scalar of the number of darts in the red region.
blue: A numerical scalar of the number of darts in the blue region.
green: A numerical scalar of the number of darts in the green region.
Hint: For each point of throwX and throwY, calculate the radius r =
Vx² + y², which is the distance from the center (0,0) to the point (x,y).
Use this radius array and logical indexing to find the number of points in each region. The radii of the three circles on
dart board are 3,
5, and 10. Thus, if a point has distance 3.45, the point lies between circles of radius 3 and 5, which is the red region.
Restrictions: Do not use if statements or switch statements. Logical indexing should be used.
Ex:
For random values of inputs,
throwX
[ 6.2945
8.1158
-7.4603
8.2675
2.6472
-8.0492
-4.4300
0.9376
9.1501
9.2978]
throwY
[-6.8477
9.4119
9.1433
-0.2925
6.0056
-7.1623
-1.5648
8.3147
5.8441
9.1898]
From throwX, throwY, the first dart has x,y coordinates as (6.2945, -6.8477), and the 10th dart has x,y coordinates as (9.2978, 9.1898).
On calling
[yellow, red, blue, green] = DartNumbers(throwX,throwY)
%3D
The output is:
yellow
red = 1
blue
4
%3D
green
5
The output indicates that for given random arrays of throwX and throwY, out of total 10 darts thrown, 0 hit the board in the yellow region, 1
hits the board in the red region, 4 hit the board in the blue region, and 5 hit the board in the green region.
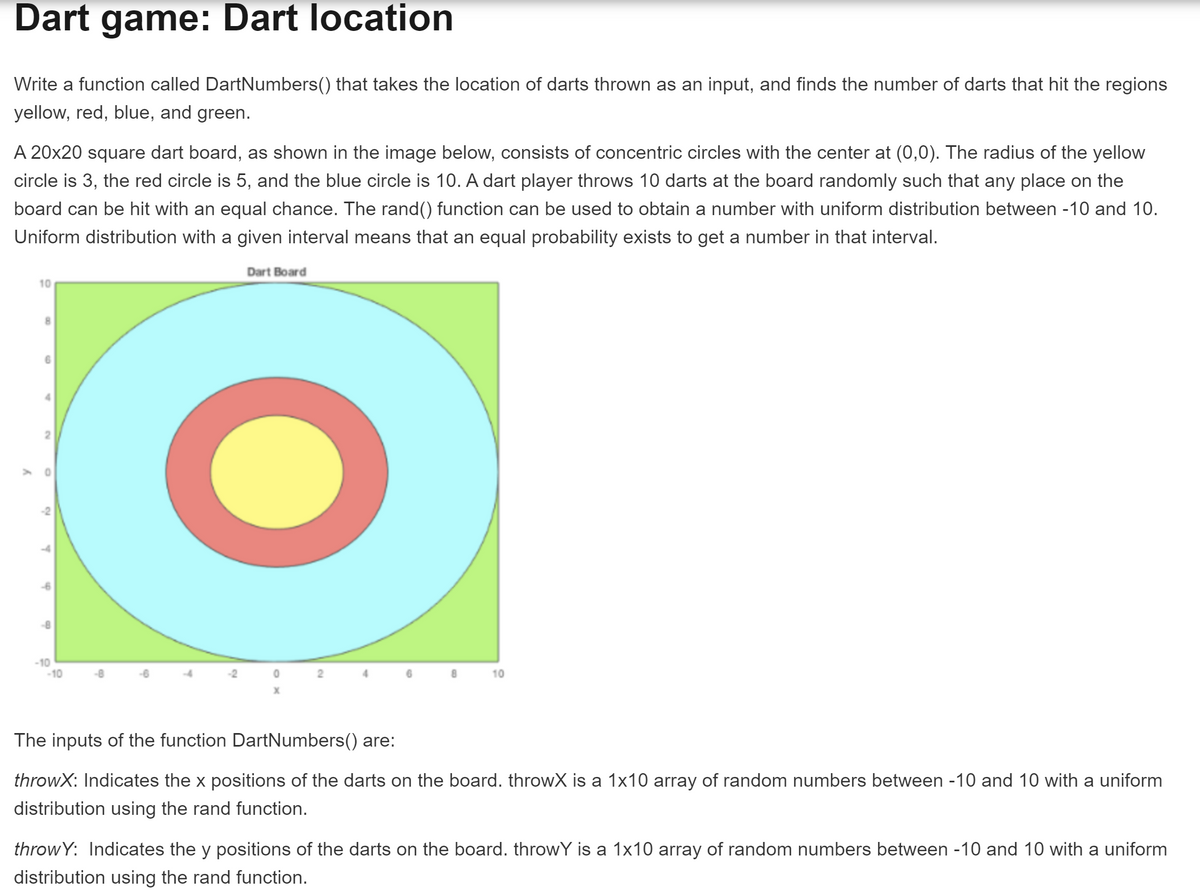
Transcribed Image Text:Dart game: Dart location
Write a function called DartNumbers() that takes the location of darts thrown as an input, and finds the number of darts that hit the regions
yellow, red, blue, and green.
A 20x20 square dart board, as shown in the image below, consists of concentric circles with the center at (0,0). The radius of the yellow
circle is 3, the red circle is 5, and the blue circle is 10. A dart player throws 10 darts at the board randomly such that any place on the
board can be hit with an equal chance. The rand() function can be used to obtain a number with uniform distribution between -10 and 10.
Uniform distribution with a given interval means that an equal probability exists to get a number in that interval.
Dart Board
10
2.
-2
-10
-10
-2
4.
8.
10
The inputs of the function DartNumbers() are:
throwX: Indicates the x positions of the darts on the board. throwX is a 1x10 array of random numbers between -10 and 10 with a uniform
distribution using the rand function.
throw Y: Indicates the y positions of the darts on the board. throwY is a 1x10 array of random numbers between -10 and 10 with a uniform
distribution using the rand function.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
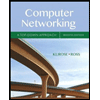
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
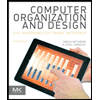
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
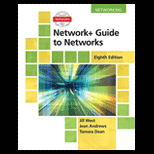
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
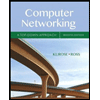
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
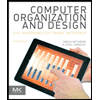
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
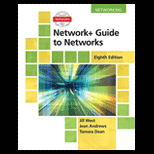
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
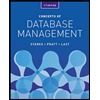
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
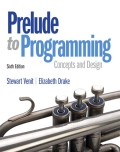
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
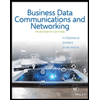
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY