• Define a class name engineClass that is used to create objects of engines. • The engine should has a data member horse_power , which is an integer o The engine should has a data member type , which is a string and stores the type information of the engine (e.g., diesel, gas, and electricity) o The engine should has a data member status , which is an integer that indicates whether the engine is running (1) or not (0) o Please define a member function operation , which accepts the user input to operate the engine (start or stop) by changing the value of status o Define a constructor that accepts user inputs for horse_power , type . The initial status should be 0 o Define a print member function that outputs the contents of the engine information (you can decide the format by yourself, but please include all the information stored in data members) • Define a class name vehicleClass that is used to create objects of vehicles vehicleclass should has a data member engine with the type engineClass vehicleclass should has another data member brand , which is a string o Define a constructor for vehicleClass , which should accept information from the user to build the data member engine and initialize the value brand o Define a print member function to print the contents of the vehicle information, it should also call the print function of data member engine
• Define a class name engineClass that is used to create objects of engines. • The engine should has a data member horse_power , which is an integer o The engine should has a data member type , which is a string and stores the type information of the engine (e.g., diesel, gas, and electricity) o The engine should has a data member status , which is an integer that indicates whether the engine is running (1) or not (0) o Please define a member function operation , which accepts the user input to operate the engine (start or stop) by changing the value of status o Define a constructor that accepts user inputs for horse_power , type . The initial status should be 0 o Define a print member function that outputs the contents of the engine information (you can decide the format by yourself, but please include all the information stored in data members) • Define a class name vehicleClass that is used to create objects of vehicles vehicleclass should has a data member engine with the type engineClass vehicleclass should has another data member brand , which is a string o Define a constructor for vehicleClass , which should accept information from the user to build the data member engine and initialize the value brand o Define a print member function to print the contents of the vehicle information, it should also call the print function of data member engine
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 5PE: Using classes, design an online address book to keep track of the names, addresses, phone numbers,...
Related questions
Question
C++
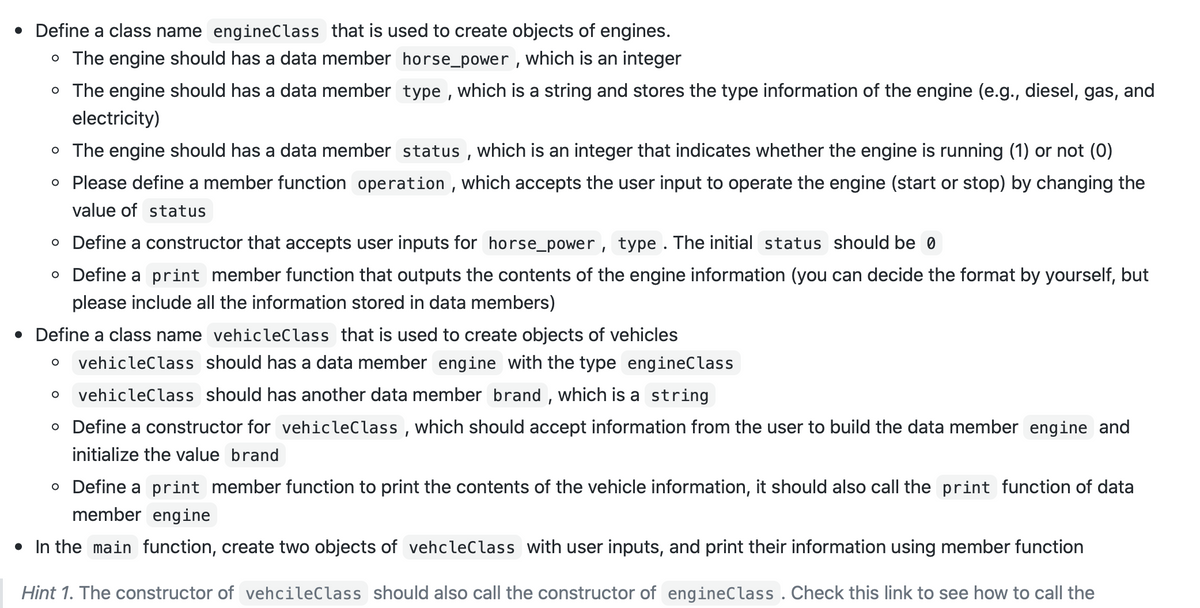
Transcribed Image Text:• Define a class name engineClass that is used to create objects of engines.
o The engine should has a data member horse_power , which is an integer
o The engine should has a data member type , which is a string and stores the type information of the engine (e.g., diesel, gas, and
electricity)
o The engine should has a data member status , which is an integer that indicates whether the engine is running (1) or not (0)
O Please define a member function operation , which accepts the user input to operate the engine (start or stop) by changing the
value of status
o Define a constructor that accepts user inputs for horse_power, type . The initial status should be 0
o Define a print member function that outputs the contents of the engine information (you can decide the format by yourself, but
please include all the information stored in data members)
• Define a class name vehicleClass that is used to create objects of vehicles
vehicleClass should has a data member engine with the type engineClass
vehicleClass should has another data member brand , which is a string
o Define a constructor for vehicleClass , which should accept information from the user to build the data member engine and
initialize the value brand
o Define a print member function to print the contents of the vehicle information, it should also call the print function of data
member engine
• In the main function, create two objects of vehcleClass with user inputs, and print their information using member function
Hint 1. The constructor of vehcileClass should also call the constructor of engineClass . Check this link to see how to call the
Expert Solution

Step 1: code
#include<iostream>
using namespace std;
//Defining class engineClass
class engineClass
{
public:
//An integer variable horse_power to store the power of vehicle
int horse_power;
//A string variable type to store the type of engine eg:diesel,gas etc
string type;
//An integer variable used to indicate whether the engine is running or not
int status;
//Function to change the status of the engine
void operation()
{
//Displaying the prperties of engine You can delete this line if you dont want to display it
cout<<"Engine type = "<<type<<" Horse power = "<<horse_power<<endl;
//If the sttus of engine is 1 then status is changed to 0 and "Engine stopped " is printed on screen
if(status==1)
{
status=0;
cout<<"Engine stopped\n";
}
//Else status is changed to 1 and a message "Engine is running is " is printed on screen
else
{
status=1;
cout<<"Engine is running\n";
}
}
};
//Defining class vehicleClass
class vehicleClass
{
//An object of engineClass engine is created inside vehicleClass
public:
engineClass engine;
};
int main()
{
//Creating 2 objects of vehicleClass
vehicleClass vehicle1,vehicle2;
//Setting the properties of vehicle1
vehicle1.engine.type="Diesel";
vehicle1.engine.horse_power=1200;
vehicle1.engine.status=0;
//Calling the function operation() in engine class
vehicle1.engine.operation();
vehicle1.engine.operation();
//Setting the properties of vehicle2
vehicle2.engine.type="gas";
vehicle2.engine.horse_power=950;
vehicle1.engine.status=0;
vehicle2.engine.operation();
return 0;
}
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
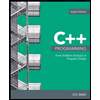
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
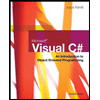
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
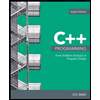
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
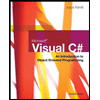
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,