Define a Rectangle class that provides getLength and getWidth. Using the findMax routines in Figure 1.25, write a main that creates an array of Rectangle and finds the largest Rectangle first on the basis of area and then on the basis of perimeter. Figure 1.25 1 // Generic findMax, with a function object, C++ style. 2 // Precondition: a.size( ) > 0. 3 template 4 const Object & findMax( const vector & arr, Comparator isLessThan ) 5 { 6 int maxIndex = 0; 7 8 for( int i = 1; i < arr.size( ); ++i ) 9 if( isLessThan( arr[ maxIndex ], arr[ i ] ) ) 10 maxIndex = i; 11 12 return arr[ maxIndex ]; 13 } 14 15 // Generic findMax, using default ordering. 16 #include 17 template 18 const Object & findMax( const vector & arr ) 19 { 20 return findMax( arr, less{ } ); 21 } 22 23 class CaseInsensitiveCompare 24 { 25 public: 26 bool operator( )( const string & lhs, const string & rhs ) const 27 { return strcasecmp( lhs.c_str( ), rhs.c_str( ) ) < 0; } 28 }; 29 30 int main( ) 31 { 32 vector arr = { "ZEBRA", "alligator", "crocodile" }; 33 34 cout << findMax( arr, CaseInsensitiveCompare{ } ) << endl; 35 cout << findMax( arr ) << endl; 36 37 return 0; 38 }
Define a Rectangle class that provides getLength and getWidth. Using the findMax routines in Figure 1.25, write a main that creates an array of Rectangle and finds the largest Rectangle first on the basis of area and then on the basis of perimeter.
Figure 1.25
1 // Generic findMax, with a function object, C++ style.
2 // Precondition: a.size( ) > 0.
3 template <typename Object, typename Comparator>
4 const Object & findMax( const
5 {
6 int maxIndex = 0;
7
8 for( int i = 1; i < arr.size( ); ++i )
9 if( isLessThan( arr[ maxIndex ], arr[ i ] ) )
10 maxIndex = i;
11
12 return arr[ maxIndex ];
13 }
14
15 // Generic findMax, using default ordering.
16 #include <functional>
17 template <typename Object>
18 const Object & findMax( const vector<Object> & arr )
19 {
20 return findMax( arr, less<Object>{ } );
21 }
22
23 class CaseInsensitiveCompare
24 {
25 public:
26 bool operator( )( const string & lhs, const string & rhs ) const
27 { return strcasecmp( lhs.c_str( ), rhs.c_str( ) ) < 0; }
28 };
29
30 int main( )
31 {
32 vector<string> arr = { "ZEBRA", "alligator", "crocodile" };
33
34 cout << findMax( arr, CaseInsensitiveCompare{ } ) << endl;
35 cout << findMax( arr ) << endl;
36
37 return 0;
38 }

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

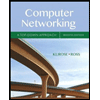
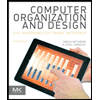
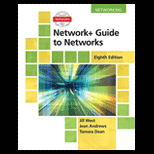
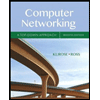
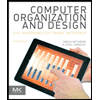
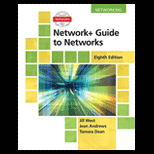
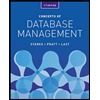
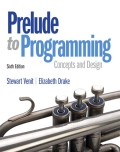
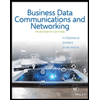