1. Write a program that implements an adjacency matrix for an undirected and unweighted graph. struct Edge { int vertexl; Int vertex2; }; Implement the following functions. a. void insertEdge (Edge e, int adjMat[4] [4]); a. //Purpose: Store an edge defined by two vertices. b. void printGraph (int adjMat[4][4]) a. //prints the edges included in an adjacency matrix c. Test your program output with graph G that has 4 vertices (1, 2, 3, and 4) and edges {(1,2), (1,4), (2,1), (2,3). (3,2), (4,1)}. 2. Write a program that implements an adjacency matrix for an undirected and unweighted graph. struct Node { int Vertex; Node* next; }; Implement the following functions. a. void printList (Node adjList[4]) b. //prints the vertices included in //an adjacency list c. Test your program output with graph G that has 4 vertices (1, 2, 3, and 4) and edges {(1,2), (1,4), (2,1), (2,3), (3,2), (4,1)}. Sample Run: Adjacency Matrix: 1 2 3 4 1 (0 (1] (81 (1] 2 [1] (8] (1 (8) 3 (8] (1] [8] (8] 4 [1] (0] (0] (0] Adjacency List:
1. Write a program that implements an adjacency matrix for an undirected and unweighted graph. struct Edge { int vertexl; Int vertex2; }; Implement the following functions. a. void insertEdge (Edge e, int adjMat[4] [4]); a. //Purpose: Store an edge defined by two vertices. b. void printGraph (int adjMat[4][4]) a. //prints the edges included in an adjacency matrix c. Test your program output with graph G that has 4 vertices (1, 2, 3, and 4) and edges {(1,2), (1,4), (2,1), (2,3). (3,2), (4,1)}. 2. Write a program that implements an adjacency matrix for an undirected and unweighted graph. struct Node { int Vertex; Node* next; }; Implement the following functions. a. void printList (Node adjList[4]) b. //prints the vertices included in //an adjacency list c. Test your program output with graph G that has 4 vertices (1, 2, 3, and 4) and edges {(1,2), (1,4), (2,1), (2,3), (3,2), (4,1)}. Sample Run: Adjacency Matrix: 1 2 3 4 1 (0 (1] (81 (1] 2 [1] (8] (1 (8) 3 (8] (1] [8] (8] 4 [1] (0] (0] (0] Adjacency List:
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 10PE
Related questions
Question
In C++ language. Sample run should be exact as the one on image. Thanks
![1. Write a program that implements an adjacency matrix for an undirected and unweighted
graph.
struct Edge
{ int vertexl;
Int vertex2;
} ;
Implement the following functions.
a. void insertEdge (Edge e, int adjMat[4] [4]);
a. //Purpose: Store an edge defined by two vertices.
b. void printGraph (int adjMat[4] [4])
a. //prints the edges included in an adjacency matrix
c. Test your program output with graph G that has 4 vertices (1, 2, 3, and 4) and edges {(1,2),
(1,4), (2,1), (2,3), (3,2), (4,1)}.
2. Write a program that implements an adjacency matrix for an undirected and unweighted graph.
struct Node
( int Vertex;
Node* next;
};
Implement the following functions.
a. void printList (Node adjList[4])
b. //prints the vertices included in //an adjacency list
c. Test your program output with graph G that has 4 vertices (1, 2, 3, and 4) and edges {(1,2),
(1,4), (2,1), (2,3), (3,2), (4,1)}.
Sample Run:
Adjacency Matrix:
1 2 3 4
1 (0] (1] (0] (1)]
2 [11 (8] (1] (8]
3 [8] [1] [0J (0]
4 [1] (0] (0] (0]
Adjacency List:
(1, 2) (1, 4> (2, 1) (2, 3) (3, 2> (4, 1)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F620c60a1-10fe-416e-8b85-1e75a34b227e%2Ff9e67c39-11dd-4553-bd1b-c7f704c4c17b%2F1d2n2as_processed.png&w=3840&q=75)
Transcribed Image Text:1. Write a program that implements an adjacency matrix for an undirected and unweighted
graph.
struct Edge
{ int vertexl;
Int vertex2;
} ;
Implement the following functions.
a. void insertEdge (Edge e, int adjMat[4] [4]);
a. //Purpose: Store an edge defined by two vertices.
b. void printGraph (int adjMat[4] [4])
a. //prints the edges included in an adjacency matrix
c. Test your program output with graph G that has 4 vertices (1, 2, 3, and 4) and edges {(1,2),
(1,4), (2,1), (2,3), (3,2), (4,1)}.
2. Write a program that implements an adjacency matrix for an undirected and unweighted graph.
struct Node
( int Vertex;
Node* next;
};
Implement the following functions.
a. void printList (Node adjList[4])
b. //prints the vertices included in //an adjacency list
c. Test your program output with graph G that has 4 vertices (1, 2, 3, and 4) and edges {(1,2),
(1,4), (2,1), (2,3), (3,2), (4,1)}.
Sample Run:
Adjacency Matrix:
1 2 3 4
1 (0] (1] (0] (1)]
2 [11 (8] (1] (8]
3 [8] [1] [0J (0]
4 [1] (0] (0] (0]
Adjacency List:
(1, 2) (1, 4> (2, 1) (2, 3) (3, 2> (4, 1)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
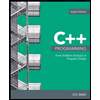
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
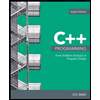
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning